Difference Between StringBuffer And StrinBuilder In Java
Differentiate Between StringBuffer And StrinBuilder
StringBuilder class is similar to StringBuffer class, except that it is non-synchronized, which means that it is not thread safe and due to this, its methods are also non-synchronized in Java.
Let’s see the differences between StringBuffer and StringBuilder are given below.
StringBuffer | StringBulider |
StringBuffer is synchronized, which means two threads cannot call the methods of StringBuffer at the same time. | StringBuilder is non-synchronized, which means two threads can call the methods of StringBuilder at the same time. |
StringBuffer is slow compared to StringBuilder. | StringBuilder is fast compared to StringBuffer. |
StringBuffer methods are synchronized. | StringBuilder methods are non-synchronized. |
StringBuffer is thread safe in Java. | StringBuilder is not thread safe in Java. |
StringBuffer is old class. it’s there in JDK from first release in Java. | StringBuilder is relatively new class. It introduced later in release of JDK 1.5. |
Example of StringBuffer class. public class BufferExp {
public static void main(String[] args) {
StringBuffer b = new StringBuffer("Bob");
b.append("Dick");
System.out.println(b);
}
}
|
Example of StringBuilder class. public class BuilderExp {
public static void main(String[] args) {
StringBuilder b = new StringBuilder("Peter");
b.append("John");
System.out.println(b);
}
}
|
Let’s check the performance of StringBuffer and StringBuilder.
Code
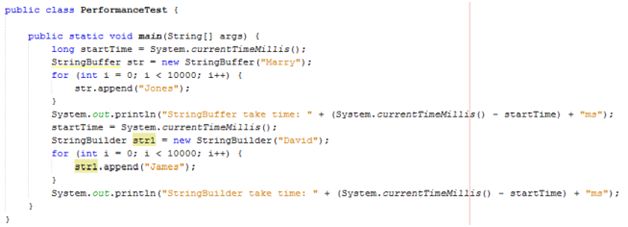
- public class PerformanceTest {
- public static void main(String[] args) {
- long startTime = System.currentTimeMillis();
- StringBuffer str = new StringBuffer("Marry");
- for (int i = 0; i < 10000; i++) {
- str.append("Jones");
- }
- System.out.println("StringBuffer take time: " + (System.currentTimeMillis() - startTime) + "ms");
- startTime = System.currentTimeMillis();
- StringBuilder str1 = new StringBuilder("David");
- for (int i = 0; i < 10000; i++) {
- str1.append("James");
- }
- System.out.println("StringBuilder take time: " + (System.currentTimeMillis() - startTime) + "ms");
- }
- }
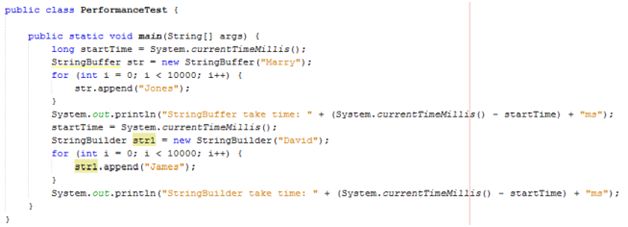
Output
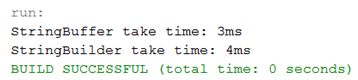
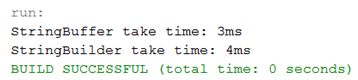
Summary
Thus, we learnt that StringBuilder class is same as StringBuffer class, except that it is non-synchronized and also learnt their differences in Java.