How To Store Image In Database
Store image in Database
In JDBC, PreparedStatement setBinaryStream() method is used to set the binary information into the the parameterIndex.
We can store the images in the database in Java by the use of PreparedStatement interface.
Syntax of setBinaryStream method
public void setBinaryStream(int paramIndex,InputStream stream) throws SQLException
public void setBinaryStream(int paramIndex,InputStream stream,long length) throws SQLException
BLOB (Binary Large Object) data type is used in the table to store an image into the database.
Example
CREATE TABLE "IMAGETABLE"
( "NAME" VARCHAR2(2000),
"PHOTO" BLOB
)
Let’s see an example, given below.
Code
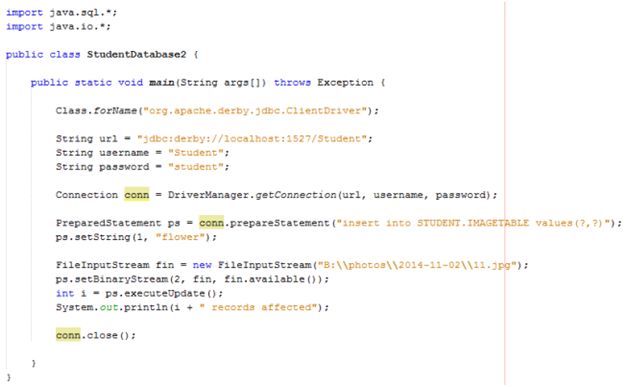
- import java.sql.*;
- import java.io.*;
- public class StudentDatabase2 {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- PreparedStatement ps = conn.prepareStatement("insert into STUDENT.IMAGETABLE values(?,?)");
- ps.setString(1, "flower");
- FileInputStream fin = new FileInputStream("B:\\photos\\2014-11-02\\11.jpg");
- ps.setBinaryStream(2, fin, fin.available());
- int i = ps.executeUpdate();
- System.out.println(i + " records affected");
- conn.close();
- }
- }
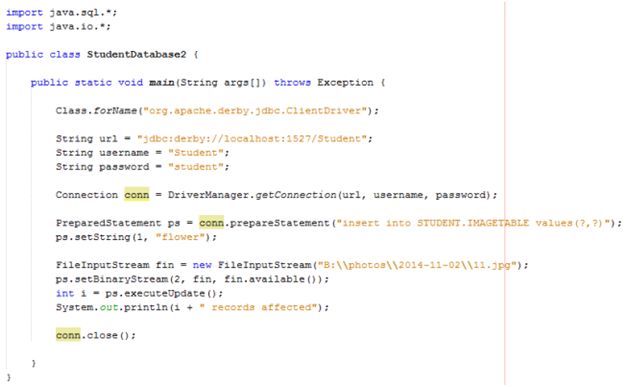
Output


In the example shown above, we are using “B:\\photos\\2014-11-02\\11.jpg” for the location of an image. We can change it according to the image location.
Summary
Thus, we learned that we can store images in the database in Java by the use of PreparedStatement interface and also learn how to store image in Java database.