Package In Java
Package
In Java, package is a collection of similar types of classes, interfaces and sub-packages. Package is used to avoid name conflicts and control the access of a class, interface and enumeration etc. Using package, it can be converted easily to locate the related classes.
Packages are two types in Java, which are,
- Built-in package
Built-in package is predefined in Java.
For example- java, lang, awt, javax, swing, net, io, util, sql etc. - User-defined package
User-defined package is created by the user.
For Example- mypack, pack etc.
Why we use package in Java
- Packages are used to classify the classes and interfaces, so that they can be easily maintained.
- Packages give an access protection.
- Package eliminates the naming collision.
Let’s see an example of package, given below.
In Java, package keyword is used to create a package. Creating package in Java is very easy. First, include a package command, followed by the name of the package as the first statement in Java source file.
Code
- package Brands;
- public class Puma {
- public static void main(String args[]) {
- System.out.println("Welcome to our Brand");
- }
- }
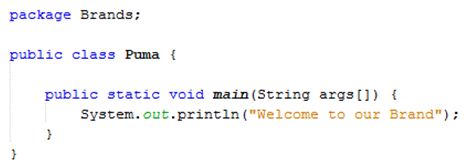
Output


In the example, mentioned above, we create a package, whose name is Brands and one simple class Puma.
If we are not using any IDE, how can we compile Java package?
Syntax
javac –d directory javafilename
Let’s see an example.
javac –d . Puma.java
The -d switch identify is the destination, where to place the generated class file. We can use any directory name like a:/xyz (in case of windows) etc. If we want to keep the package within the same directory, we can use . (dot).
Now, how to run Java package program
We need to use fully qualify the name of program.
Example
Compile: javac –d . Puma.java
Run: java brands.Puma
The -d is a switch, which tells the compiler, where to locate the class file i.e. it represents the destination. The . represents the current folder.
Access package from another package
Import Keyword
In Java, import keyword is used to import built-in and the user-defined packages into our Java source file. Thus, our class can refer to a class, which is in another package by directly using its name.
Three approaches to access the package from outside the package are,
- import package.*;
- import package.classname;
- Using fully qualified name.
Import all the classes from the particular package. (import package.*)
The import keyword is used to put together the classes and interfaces of this package will be accessible.
When we use package.* then all the classes and interfaces of this package will be accessible.
For example.
Code
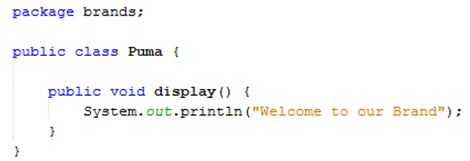
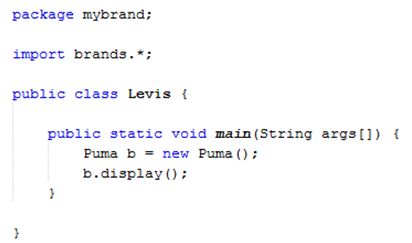
- package brands;
- public class Puma {
- public void display() {
- System.out.println("Welcome to our Brand");
- }
- }
- package mybrand;
- import brands.*;
- public class Levis {
- public static void main(String args[]) {
- Puma b = new Puma();
- b.display();
- }
- }
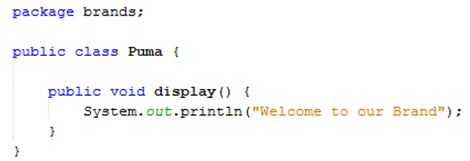
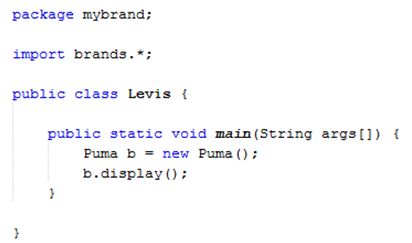
Output


Import the only class you want to use. (package.classname)
When we import package.classname, only declared class of this package will be accessible.
For example
Code
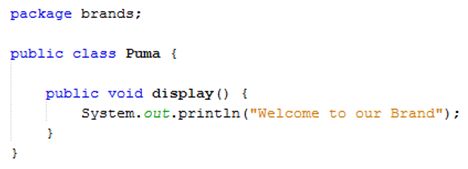
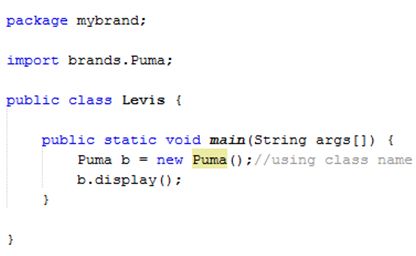
- package brands;
- public class Puma {
- public void display() {
- System.out.println("Welcome to our Brand");
- }
- }
- package mybrand;
- import brands.Puma;
- public class Levis {
- public static void main(String args[]) {
- Puma b = new Puma();
- b.display();
- }
- }
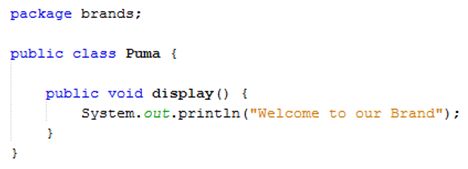
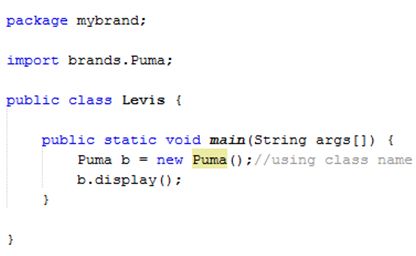
Output


Using fully qualified name
When we use fully qualified name, only declared class of this package will be accessible. Now, there is no need to import the package but we need to use fully qualified name each or every time, when we want to access the class or an interface but this is not a good practice. It is generally used when two packages have same class name e.g. java.util and java.sql packages contain Date class.
For example
Code
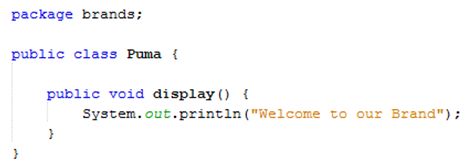
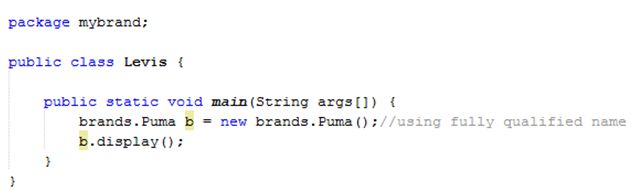
- package brands;
- public class Puma {
- public void display() {
- System.out.println("Welcome to our Brand");
- }
- }
- package mybrand;
- public class Levis {
- public static void main(String args[]) {
- brands.Puma b = new brands.Puma(); //using fully qualified name
- b.display();
- }
- }
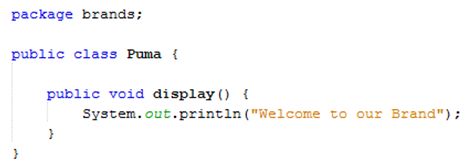
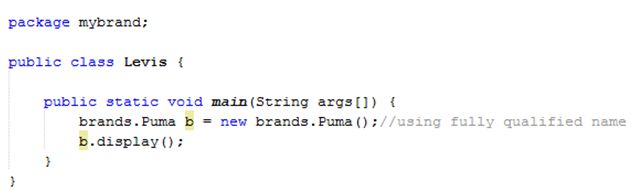
Output


Import statement must be writing after the package statement.
For example
- package mybrand;
- import java.lang.*;
If we are not creating any package in our program and import statement will be the first statement of our Java program.
If we import a package, subpackages will not be imported.
When we import a package, all the classes and interface of that package will be imported but not including the classes and interfaces of the subpackages. Hence, if we want to use any subpackage that time, we need to import the subpackage as well.
Let’s see the sequence of the program, which is,
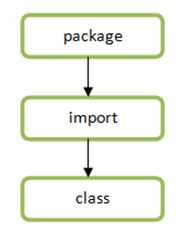
When we declare one package inside another package, it is called the sub-package in Java. It should be created to categorize the package further.
Summary
Thus, we learnt that package is a collection of similar types of classes, interfaces, sub-packages and also learnt how to use it in Java.