Finally Block In Java
Finally Block
In Java, finally block is used to perform important code such as closing connection, stream etc.
Finally block is mainly used in Java. It always execute whether an exception is handled or not. It must be followed by try or catch block.
Let’s understand with the flowchart, given below.
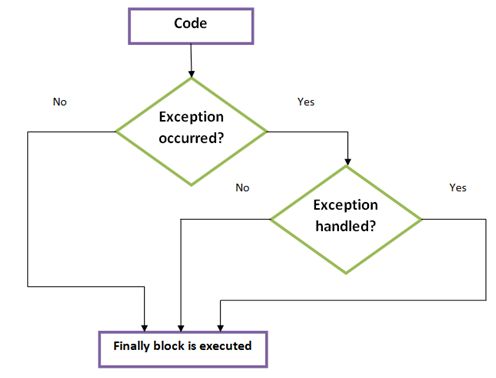
Why we use Java finally?
Finally block allows us to run any cleanup-type statements, which we want to execute, no matter what happens in the protected code such as closing a file, closing connection etc.
Usage of finally block
Let's see an example, given below, where an exception doesn't occur.
Code
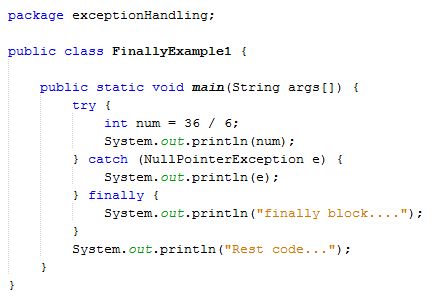
- package exceptionHandling;
- public class FinallyExample1 {
- public static void main(String args[]) {
- try {
- int num = 36 / 6;
- System.out.println(num);
- } catch (NullPointerException e) {
- System.out.println(e);
- } finally {
- System.out.println("finally block....");
- }
- System.out.println("Rest code...");
- }
- }
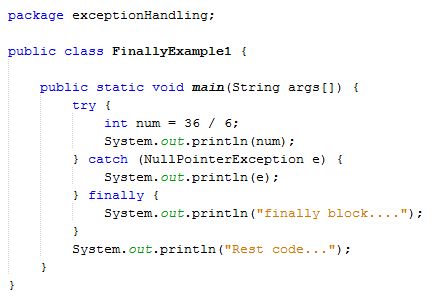
Output
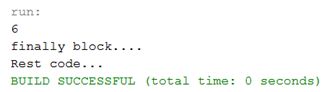
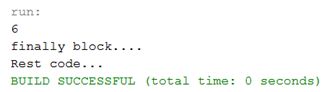
Let's see an example, given below, where an exception occurs and not handled.
Code
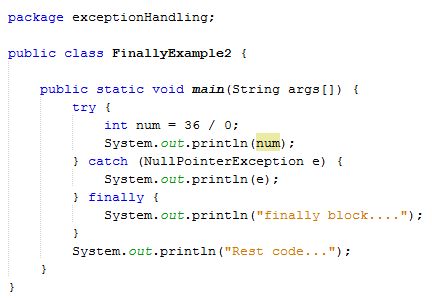
- package exceptionHandling;
- public class FinallyExample2 {
- public static void main(String args[]) {
- try {
- int num = 36 / 0;
- System.out.println(num);
- } catch (NullPointerException e) {
- System.out.println(e);
- } finally {
- System.out.println("finally block....");
- }
- System.out.println("Rest code...");
- }
- }
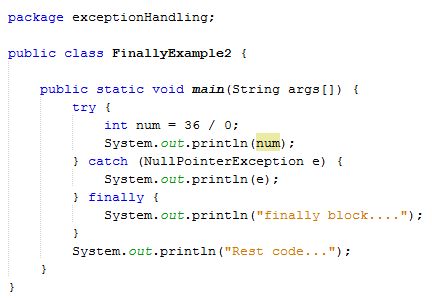
Output


Let's see an example, given below, where an exception occurs and handles.
Code
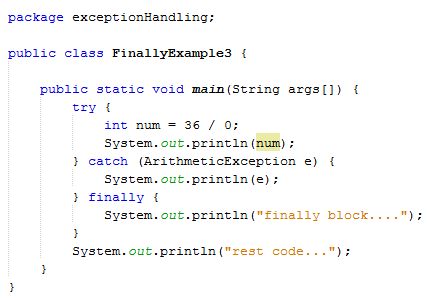
- package exceptionHandling;
- public class FinallyExample3 {
- public static void main(String args[]) {
- try {
- int num = 36 / 0;
- System.out.println(num);
- } catch (ArithmeticException e) {
- System.out.println(e);
- } finally {
- System.out.println("finally block....");
- }
- System.out.println("rest code...");
- }
- }
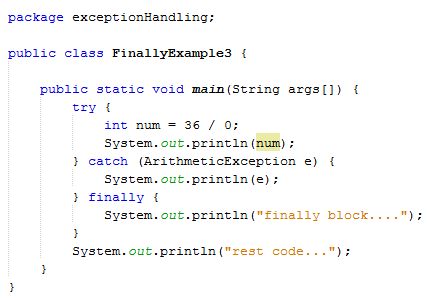
Output
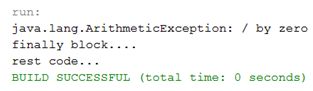
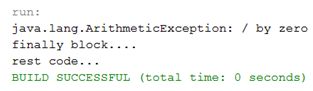
When we don't handle the exception before terminating the program, JVM executes finally block automatically.
Each try block can be zero or more catch blocks, but only one finally block and the finally block does not execute if the program exits.
Summary
Thus, we learnt that finally block is used to perform an important code and also learnt why we need to use it in Java.