Instance Initializer Block In Java
Instance Initializer Block
In Java, instance initializer block is used to initialize the instance variables inside a class. It is a very simple block without any modifiers. We can use it inside any class. It runs each and every time, when an object of the class is created.
We can initialize the instance variable directly. With the usage of instance initializer block, we can perform extra operations as initializing the instance variable in the instance initializer block.
In Java, we can use an instance initializer block operations in three places, which are,
- Method
- Constructor
- block
Let’s see an example, given below, to initialize a value directly.
Code
- class Student {
- int rollno = 101; // directly initialize a value.
- }
Now, let’s see an example with the instance initializer block, given below.
Code
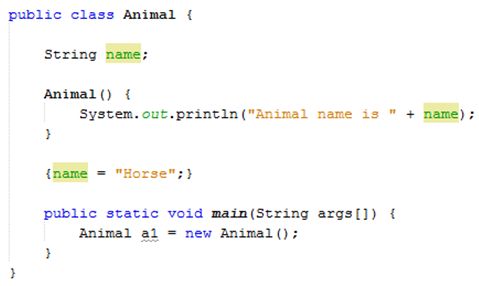
- public class Animal {
- String name;
- Animal() {
- System.out.println("Animal name is " + name);
- } {
- name = "Horse";
- } //initialize a value in Instance initializer block
- public static void main(String args[]) {
- Animal a1 = new Animal();
- }
- }
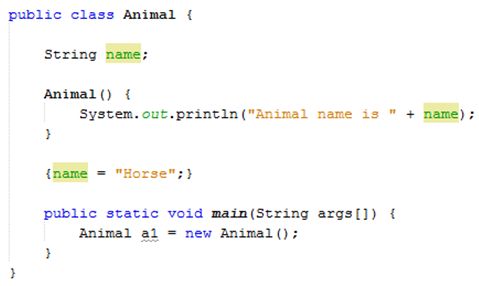
Output


In Java, why we use instance initializer block?
Instance initializer block is used to perform some extra operations as initializing a value to instance variables. For example- for loop is required to fill a complex array etc.
Rules for instance initializer block
- Instance initializer block is created when an instance of the class is created.
- Instance initializer block is calling after the parent class constructor (i.e. after super() constructor call).
- Instance initializer block comes in the order in which they appear.
Instance initializer block or constructor is invoked first?
Let’s see
Code
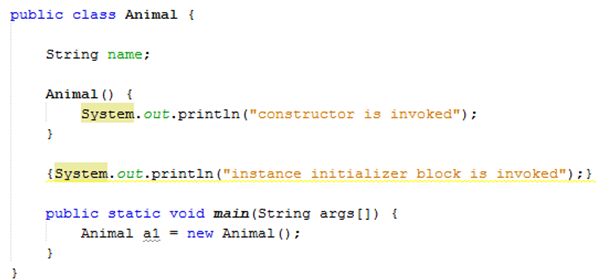
- public class Animal {
- String name;
- Animal() {
- System.out.println("constructor is invoked");
- } {
- System.out.println("instance initializer block is invoked");
- }
- public static void main(String args[]) {
- Animal a1 = new Animal();
- }
- }
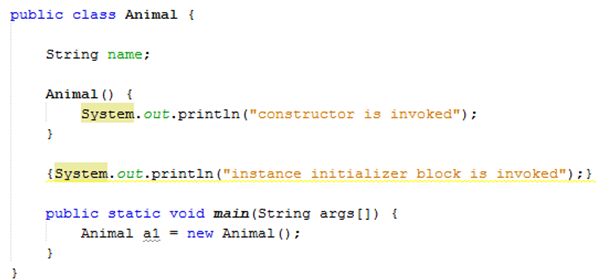
Output
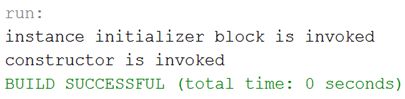
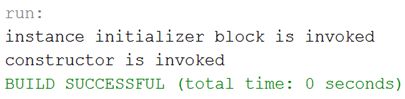
In the above example, when object is created, an instance intializer block is invoked. In this example, we say that instance initialize block is invoked first but no, it is not the fact. In Java, the compiler copies the instance initializer block in the constructor after super (). Hence, the constructor is invoked first.
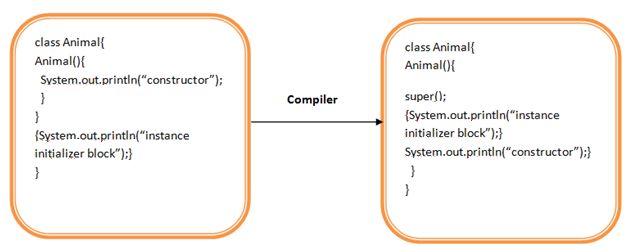
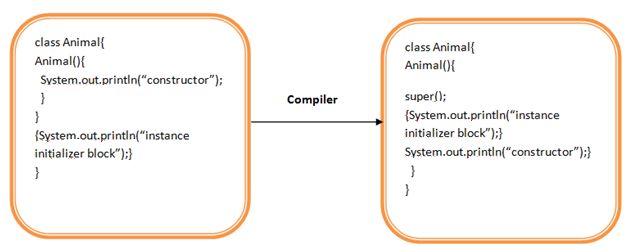
Instance initializer block is call after the parent class constructor (i.e. after super () constructor call).
Let’s see an example, given below.
Code
- class Animal {
- Animal() {
- System.out.println("parent constructor");
- }
- }
- public class Horse extends Animal {
- Horse() {
- super();
- System.out.println("child constructor");
- } {
- System.out.println("instance initializer block");
- }
- public static void main(String args[]) {
- Horse h = new Horse();
- }
- }
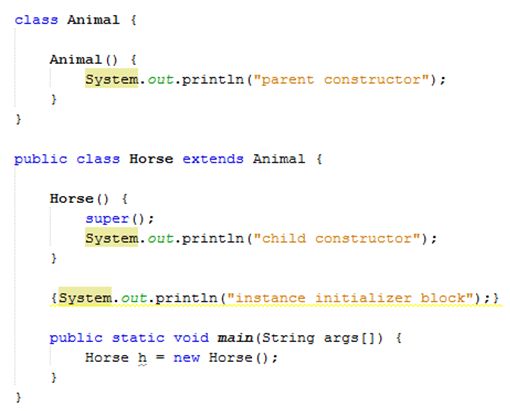
Output
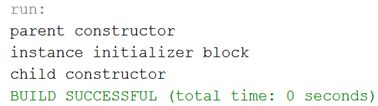
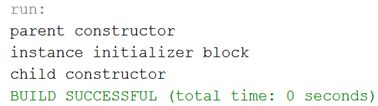
In the example, shown above, instance initializer block is called after the parent class constructor i.e. after super() constructor is called.
Summary
Thus, we learnt instance initializer block is used to initialize the instance variables inside a class and also learnt how to use it.