How To Open Dialog Box In Swing Java
Open Dialog Box in Swing
Let’s see an example of open dialog box in Swin, given below.
Code
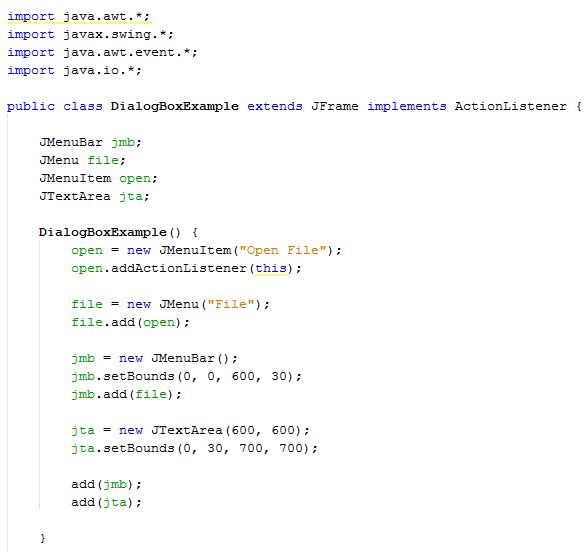
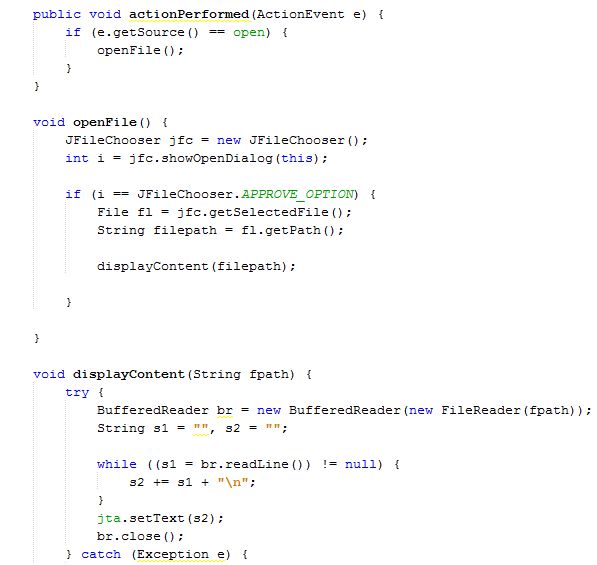
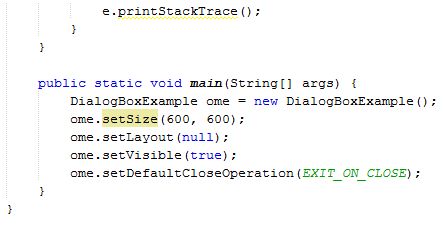
- import java.awt.*;
- import javax.swing.*;
- import java.awt.event.*;
- import java.io.*;
- public class DialogBoxExample extends JFrame implements ActionListener {
- JMenuBar jmb;
- JMenu file;
- JMenuItem open;
- JTextArea jta;
- DialogBoxExample() {
- open = new JMenuItem("Open File");
- open.addActionListener(this);
- file = new JMenu("File");
- file.add(open);
- jmb = new JMenuBar();
- jmb.setBounds(0, 0, 600, 30);
- jmb.add(file);
- jta = new JTextArea(600, 600);
- jta.setBounds(0, 30, 700, 700);
- add(jmb);
- add(jta);
- }
- public void actionPerformed(ActionEvent e) {
- if (e.getSource() == open) {
- openFile();
- }
- }
- void openFile() {
- JFileChooser jfc = new JFileChooser();
- int i = jfc.showOpenDialog(this);
- if (i == JFileChooser.APPROVE_OPTION) {
- File fl = jfc.getSelectedFile();
- String filepath = fl.getPath();
- displayContent(filepath);
- }
- }
- void displayContent(String fpath) {
- try {
- BufferedReader br = new BufferedReader(new FileReader(fpath));
- String s1 = "", s2 = "";
- while ((s1 = br.readLine()) != null) {
- s2 += s1 + "\n";
- }
- jta.setText(s2);
- br.close();
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
- public static void main(String[] args) {
- DialogBoxExample ome = new DialogBoxExample();
- ome.setSize(600, 600);
- ome.setLayout(null);
- ome.setVisible(true);
- ome.setDefaultCloseOperation(EXIT_ON_CLOSE);
- }
- }
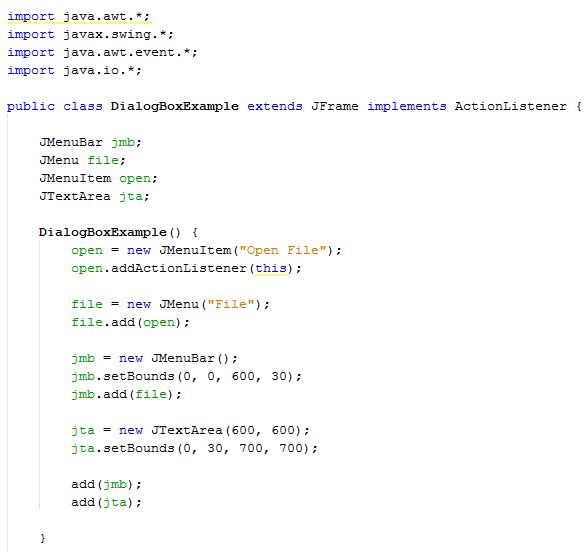
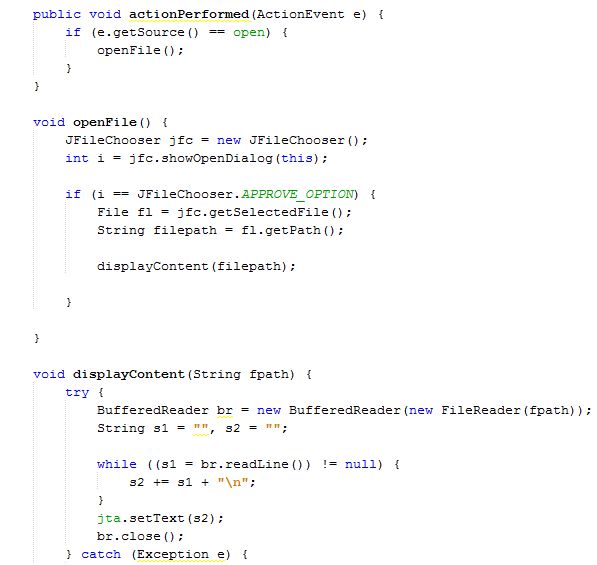
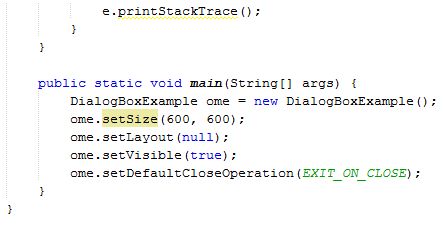
Output
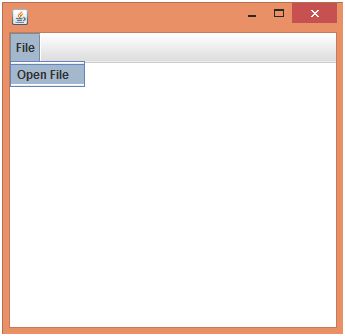
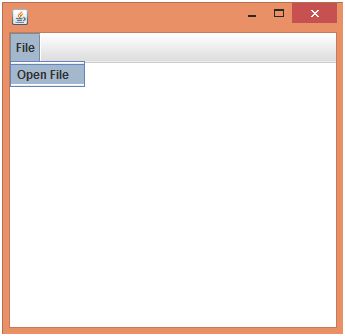
Summary
Thus, we learnt how to open dialog box in Swing Java.