Interface In Java
Interface
An interface in Java is similar to a class and we can say that it is a blueprint of a class.
- Interface is mainly used to achieve full abstraction in Java.
- Interface has static constants and abstract method body only and not method body.
- Interface supports multiple inheritance in Java.
- An interface shows IS-A relationship also.
- Interface can’t be instantiated, as in case of an abstract class.
Main advantages of interface in Java are,
- To achieve full abstraction.
- To achieve multiple inheritance.
- To achieve loose coupling.
In Java, the compiler by default adds public and abstract keywords before the interface method and public, static and final keyword before the variables. It means that all the variables of an interface are public, static, final and methods are public and abstract.
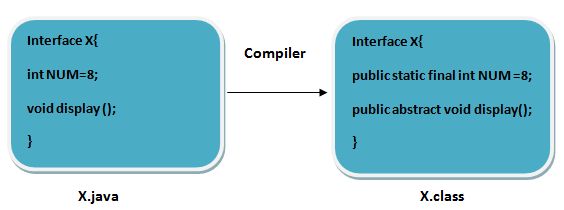
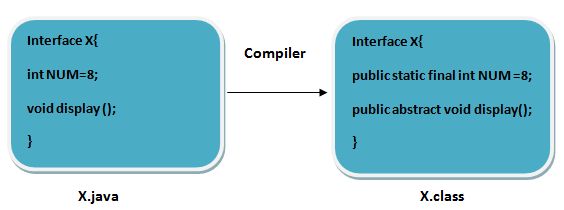
Relationship between class and interface
- A class extends another class.
- An interface extends another interface.
- A class implements an interface.
Now, let’s see an example of an interface, given below.
Code
- interface Car {
- void run();
- }
- public class Audi implements Car {
- public void run() {
- System.out.println("Audi is a fast car");
- }
- public static void main(String args[]) {
- Audi obj = new Audi();
- obj.run();
- }
- }
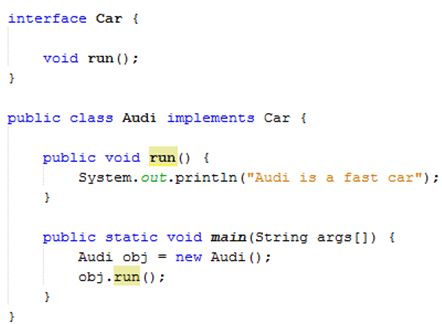
Output


Multiple inheritance through interface
Multiple inheritance means when a class implements multiple interfaces or an interface extends multiple interfaces.
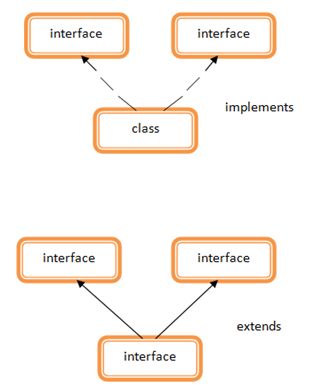
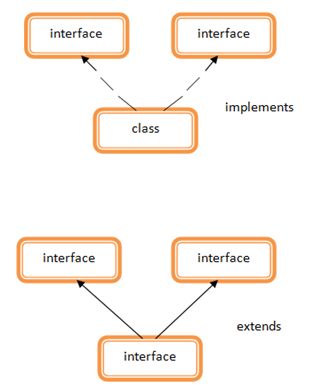
Let’s see an example of multiple inheritance in Java through an interface, given below.
Code
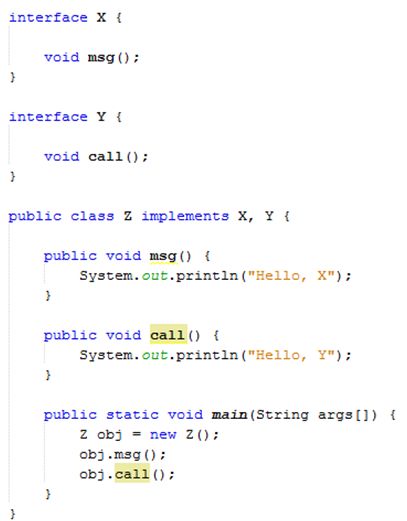
- interface X {
- void msg();
- }
- interface Y {
- void call();
- }
- public class Z implements X, Y {
- public void msg() {
- System.out.println("Hello, X");
- }
- public void call() {
- System.out.println("Hello, Y");
- }
- public static void main(String args[]) {
- Z obj = new Z();
- obj.msg();
- obj.call();
- }
- }
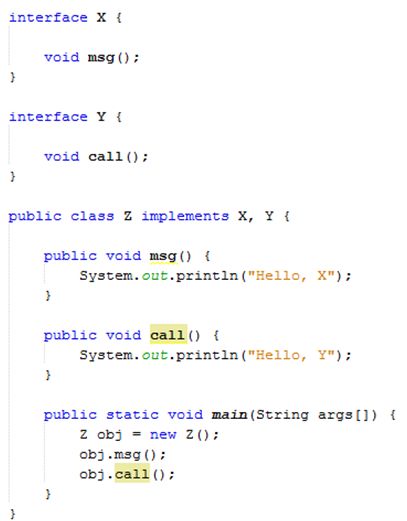
Output
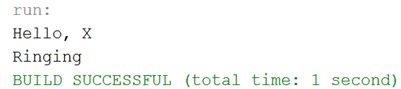
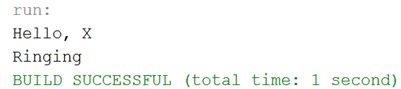
In Java, Multiple inheritance is not supported through class. It is possible through an interface because interface methods implementation provided by the other class. Hence, there is no ambiguity.
Let’s see an example, given below.
Code
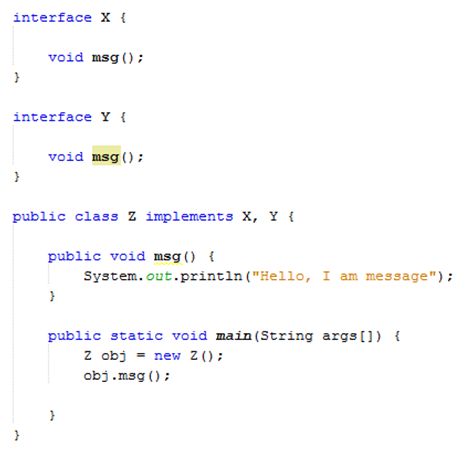
- interface X {
- void msg();
- }
- interface Y {
- void msg();
- }
- public class Z implements X, Y {
- public void msg() {
- System.out.println("Hello, I am message");
- }
- public static void main(String args[]) {
- Z obj = new Z();
- obj.msg();
- }
- }
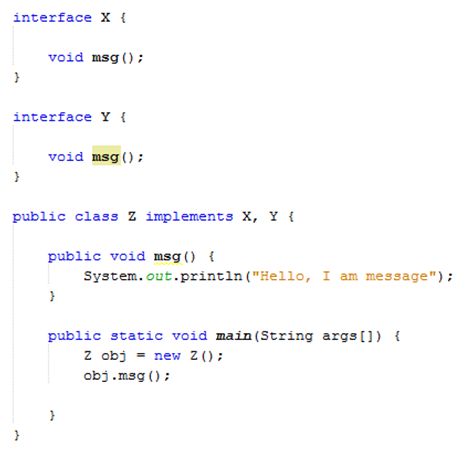
Output


In the example, shown above, X and Y interface have same methods but its implementation is provided by class Z due to which there is no ambiguity.
Interface inheritance in Java
In Java, interface inheritance means one interface extends another interface.
Let’s see an example, given below.
Code
- interface Car {
- void run();
- }
- interface Bike extends Car {
- void stop();
- }
- public class ExampleTest implements Bike {
- public void run() {
- System.out.println("Car running...");
- }
- public void stop() {
- System.out.println("Bike stop...");
- }
- public static void main(String args[]) {
- ExampleTest obj = new ExampleTest();
- obj.run();
- obj.stop();
- }
- }
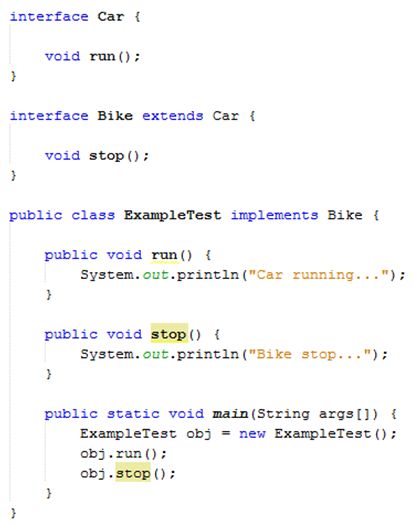
Output
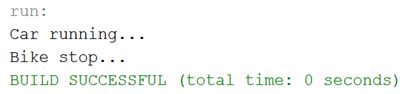
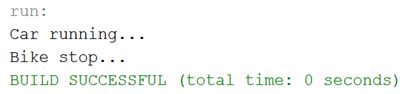
Marker interface
In Java, an interface has no members or no body due to which, it is called as a marker interface or tagged interface. For example- Serializable, Cloneable, Remote etc. Marker interface is mainly used to provide some essential information to JVM and JVM is used it to perform some useful operations.
Nested interface
When an interface is declared in another interface, it is called as a nested interface in Java.
Summary
Thus, we learnt that an interface is mainly used to achieve full abstraction in Java and also learnt multiple inheritance through an interface.