String Class Methods In Java
String Class Methods
String class provides many methods to work on the strings.
With the help of these methods, we can perform many operations on the string such as trimming, concatenating, converting, comparing, replacing strings, formatting string, substring etc.
Java string is a robust and very useful concept because everywhere we use string. Everything is treated as a string, when we submit any form in Window based, Web based or mobile Application, we use the string.
Let's see some important methods of String class, which we use mostly in programming.
String toUpperCase() and toLowerCase() method in Java
In Java, string toUpperCase() method is used to convert the string into uppercase letter and string toLowerCase() method is used to convert the string into lowercase letter.
Let’s see an example, given below.
Code
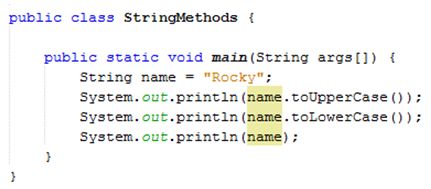
- public class StringMethods {
- public static void main(String args[]) {
- String name = "Rocky";
- System.out.println(name.toUpperCase());
- System.out.println(name.toLowerCase());
- System.out.println(name);
- }
- }
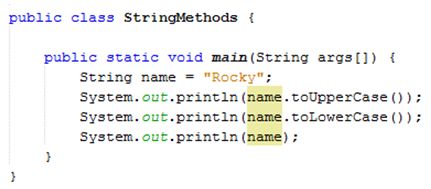
Output
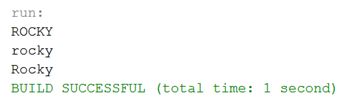
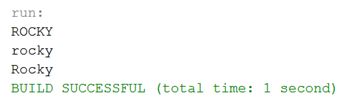
String trim() method in Java
In Java, string trim() method is used to eliminate the white spaces before and after the string.
Let’s see an example, given below.
Code
- public class StringMethods {
- public static void main(String args[]) {
- String animal = " White Elephant ";
- System.out.println(animal);
- System.out.println(animal.trim());
- }
- }
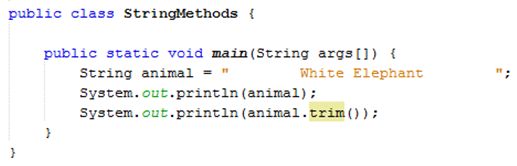
Output
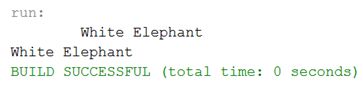
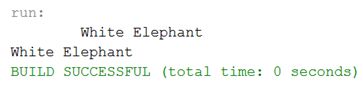
String startsWith() and endsWith() method in Java
In Java, string startsWith() and endsWith method is used to checks if string starts or ends with given prefix. Hence, it returns true else it returns false.
Let’s see an example, given below.
Code
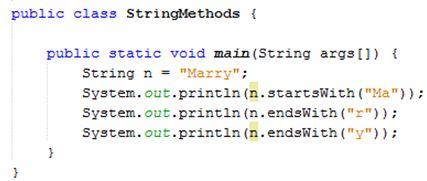
- public class StringMethods {
- public static void main(String args[]) {
- String n = "Marry";
- System.out.println(n.startsWith("Ma"));
- System.out.println(n.endsWith("r"));
- System.out.println(n.endsWith("y"));
- }
- }
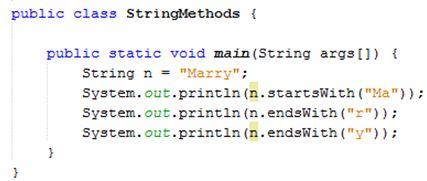
Output
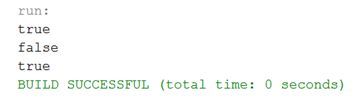
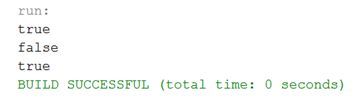
String charAt() method in Java
In Java, string charAt() method is used to return a character at particular index.
Let’s see an example, given below.
Code
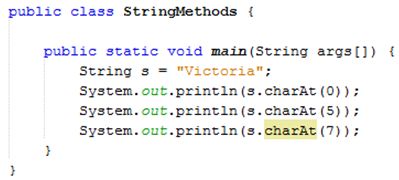
- public class StringMethods {
- public static void main(String args[]) {
- String s = "Victoria";
- System.out.println(s.charAt(0));
- System.out.println(s.charAt(5));
- System.out.println(s.charAt(7));
- }
- }
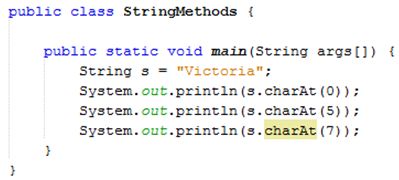
Output
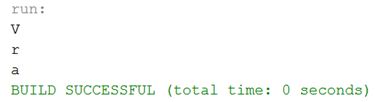
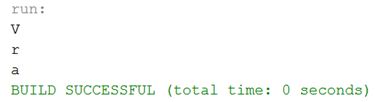
String length() method in Java
In Java, string length() method returns the length of the string.
Let’s see an example, given below.
Code
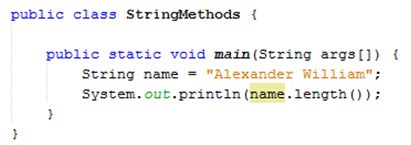
- public class StringMethods {
- public static void main(String args[]) {
- String name = "Alexander William";
- System.out.println(name.length());
- }
- }
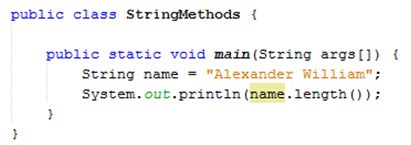
Output


String intern() method in Java
In Java, a pool of strings is initially empty. It’s maintained privately by the class String.
If the intern method is called and the pool already contains a string equal to the String object as determined by the equals() method, the string from the pool is returned. The string object is added to the pool and a reference to the string object is returned.
Let’s see an example, given below.
Code
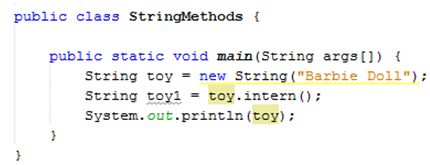
- public class StringMethods {
- public static void main(String args[]) {
- String toy = new String("Barbie Doll");
- String toy1 = toy.intern();
- System.out.println(toy);
- }
- }
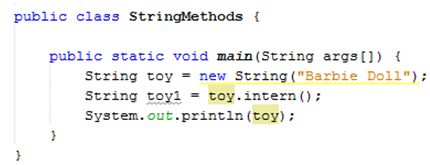
Output


String valueOf() method in Java
In Java, this string method is used to change the given data type into the string.
Let’s see an example, given below.
Code
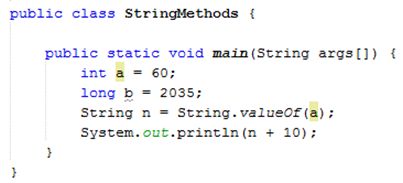
- public class StringMethods {
- public static void main(String args[]) {
- int a = 60;
- long b = 2035;
- String n = String.valueOf(a);
- System.out.println(n + 10);
- }
- }
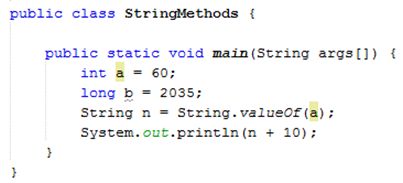
Output


String replace() method in Java
In Java, this method is used to replace all the occurrences of the first order of character with the second order of character.
Let’s see an example, given below.
Code
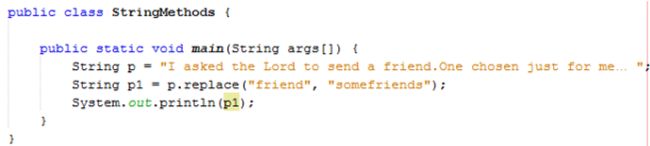
- public class StringMethods {
- public static void main(String args[]) {
- String p = "I asked the Lord to send a friend.One chosen just for me… ";
- String p1 = p.replace("friend", "somefriends");
- System.out.println(p1);
- }
- }
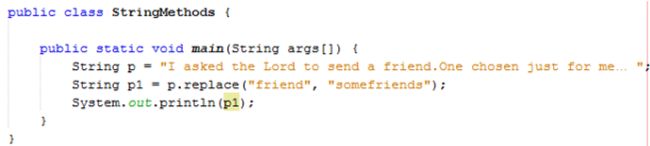
Output


Summary
Thus, we learnt that string class provides many methods to work on the strings and also learnt their usage.