How To Call Private Method From Another Class In Java
Call private method from another class
In Java, we can call the private method from outside the class through changing the runtime behaviour of the class and with the help of java.lang.Class and java.lang.reflect.Method classes. We can call private method from any other class.
Methods of Method class
public void setAccessible(boolean status) throws SecurityException
This method is used to set the accessibility of the method.
public Object invoke(Object method, Object... args) throws IllegalAccessException, IllegalArgumentException, InvocationTargetException
This method is used to call the method.
Method of Class class
public Method getDeclaredMethod(String name,Class[] parameterTypes)throws NoSuchMethodException,SecurityException
This method is used to return a method object, which reflects the specific declared method of the class or an interface, represented by the class object.
Let's see an example to call private method from another class.
Code
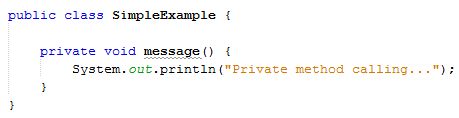
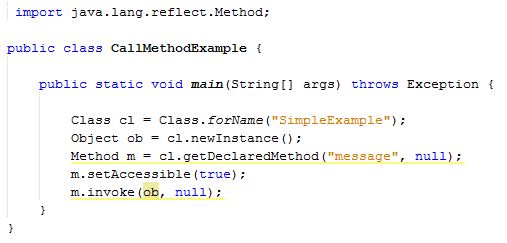
- public class SimpleExample {
- private void message() {
- System.out.println("Private method calling...");
- }
- }
- import java.lang.reflect.Method;
- public class CallMethodExample {
- public static void main(String[] args) throws Exception {
- Class cl = Class.forName("SimpleExample");
- Object ob = cl.newInstance();
- Method m = cl.getDeclaredMethod("message", null);
- m.setAccessible(true);
- m.invoke(ob, null);
- }
- }
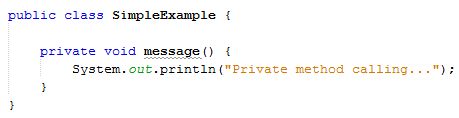
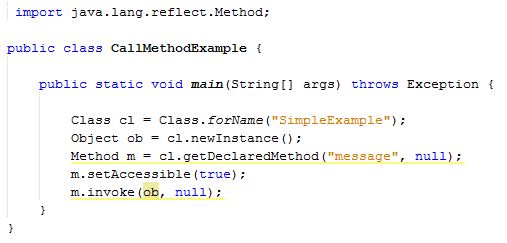
Let's see another example to call the parameterized private method from another class.
Code
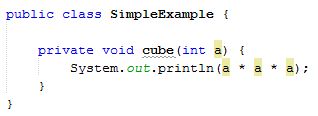
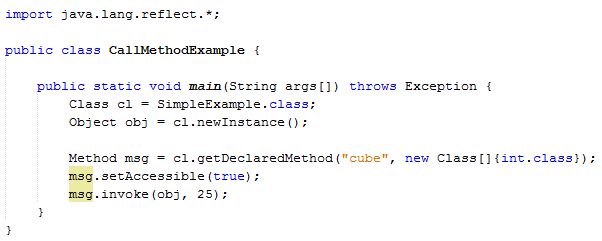
- public class SimpleExample {
- private void cube(int a) {
- System.out.println(a * a * a);
- }
- }
- import java.lang.reflect.*;
- public class CallMethodExample {
- public static void main(String args[]) throws Exception {
- Class cl = SimpleExample.class;
- Object obj = cl.newInstance();
- Method msg = cl.getDeclaredMethod("cube", new Class[]{int.class});
- msg.setAccessible(true);
- msg.invoke(obj, 25);
- }
- }
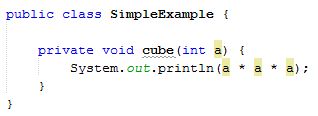
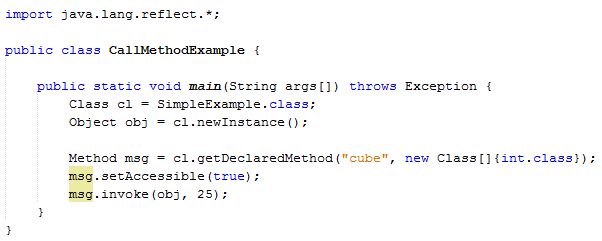
Output


Summary
Thus, we learnt that with the help of java.lang.Class and java.lang.reflect.Method classes, we can call private method from any other class and also learnt how to create it in Java.