Properties Class In Java
Properties Class
In Java, the properties object holds both key and value pair as a string. It is the sub class of Hashtable and we can use to get the property value, based on the property key.
Properties class provides the methods to get the data from the properties file and store the data into the properties file. Besides, it can be used to get the properties of the system.
Advantage of Properties File
Maintenance is very easy- When any information is changed from the properties file, we don't need to recompile the class of Java. It is generally used to hold the variable information i.e. to be changed.
Constructors of Properties Class
Properties( )
This constructor is used to create properties object, which has no default values.
Properties(Properties propDefault)
This constructor is used to create an object, which uses propDefault for its default values. In both cases, the property list is empty.
Methods of Properties Class
String getProperty(String key)
This method is used to return the value associated with the key. A null object is returned, if the key is neither in the list nor in the default property list.
String getProperty(String key, String defaultProperty)
This method is used to return the value associated with the key. defaultProperty is returned, when the key is neither in the list nor in the default property list.
void list(PrintStream streamOut)
This method is used to send the property list to the output stream linked to streamOut.
void list(PrintWriter streamOut)
This method is used to send the property list to the output stream linked to streamOut.
void load(InputStream streamIn) throws IOException
This method is used to input a property list from the input stream linked to streamIn.
Enumeration propertyNames( )
This method is used to return an enumeration of the keys. It includes those keys, which are found in the default property list, too.
Object setProperty(String key, String value)
This method is used to associate value with the key. It returns the previous value associated with key, or returns null, if no such association exists.
void store(OutputStream streamOut, String description)
This method is used after writing the string, specified by the description, the property list is written to the output stream linked to streamOut.
Let’s see an example, given below.
Code
- import java.util.*;
- public class PropertiesClass {
- public static void main(String args[]) {
- Properties c = new Properties();
- Set s;
- String st;
- c.put("Washington", "Olympia");
- c.put("California", "Sacramento");
- c.put("Indiana", "Indianapolis");
- s = c.keySet();
- Iterator i = s.iterator();
- while (i.hasNext()) {
- st = (String) i.next();
- System.out.println("The capital of " + st + " is " + c.getProperty(st) + ".");
- }
- System.out.println();
- st = c.getProperty("Florida", "Not Found");
- System.out.println("The capital of Florida is " + st + ".");
- }
- }
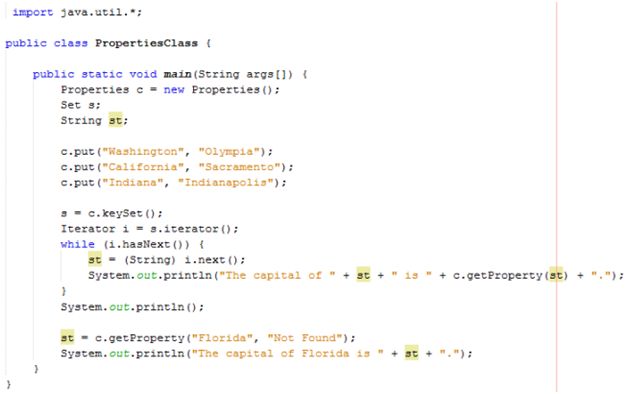
Output
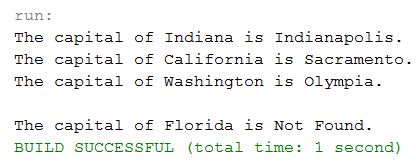
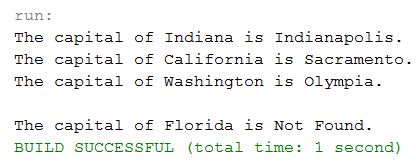
Summary
Thus, we learnt that the properties object holds both key and value pair as a string. It is the sub class of Hashtable and we can use it to get the property value, based on the property key and we also learnt how to create it in Java.