Local Inner Class In Java
Local inner class
A class which is created inside the method is known as a local inner class in Java and if we want to call the methods of local inner class then we must instantiate this class inside the method.
In other words, when we write any class within a method and that class called as a local type such as local variables scope of the local inner class is restricted within the method (it can't be private, public or protected) and local inner classes can instantiated only in the method, where the inner class is defined in the program.
Let’s see an example with an instance variable, given below.
Code
- public class Tiger {
- private int age = 20;
- void display() {
- class Cub {
- void calcAge() {
- System.out.println(age);
- }
- }
- Cub t = new Cub();
- t.calcAge();
- }
- public static void main(String args[]) {
- Tiger t1 = new Tiger();
- t1.display();
- }
- }
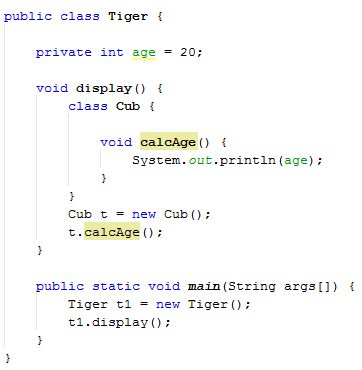
Output


In the example, mentioned above, internally the compiler generated a class, which has the reference of the outer class.
Some important rules are,
Local inner class can’t be called from outside the method in a class.
Local inner class can’t access non-final local variable till JDK 1.7. With JDK 1.8, it is possible to access the non-final local variable in local inner class.
Let’s see an example with the local variable, given below.
Code
- public class Tiger {
- private int age = 20;
- void display() {
- int tigerAge = 50; //local variable must be final till jdk 1.7 only
- class Cub {
- void calcAge() {
- System.out.println(tigerAge);
- }
- }
- Cub t = new Cub();
- t.calcAge();
- }
- public static void main(String args[]) {
- Tiger t1 = new Tiger();
- t1.display();
- }
- }
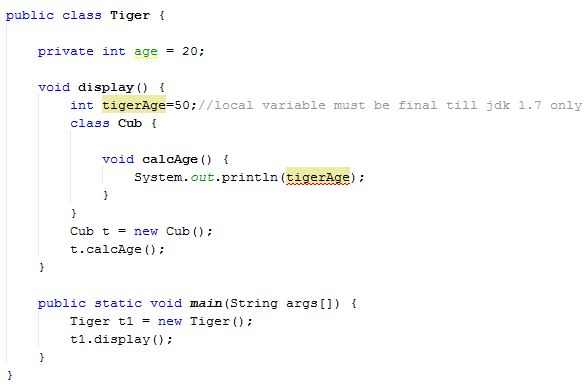
In the example, mentioned above, we can see that the program gives a compile time error because we use jdk 8.0 and if we use older version of jdk, this program runs successfully.
Summary
Thus, we learnt that a class, which is created inside the method is known as a local inner class in Java and also learnt some important rules of Local inner class.