How To Create Custom Annotation In Java
Custom Annotation
A custom annotation means user-defined annotations. Custom annotations are easy to create and use.
The @interface element is used to create an annotation.
For example
@interface UserAnnotation{}
Now, userannotation is the custom annotation name.
Some important points for Java custom annotation signature are given below.
There are some points that must be kept in mind by the programmer.
- Method must not have any throws clauses.
- Method must return one of the following- primitive data types, String, Class, enum or an array of these data types.
- Method must not have any parameter.
- We must attach @ just before interface keyword to define annotation.
- It may give a default value to the method.
Types of Annotation
In Java, there are three kinds of annotations, which are,
- Marker Annotation
An annotation, which has no method is called as a Marker annotation.
For example
@interface UserAnnotation{}
The @Override and @Deprecated are Marker annotations.
Single-Value Annotation
An annotation, which has one method is called as a Single-value annotation.
For example
@interface UserAnnotation{
int value();
}
We can give the default value also.
For example
@interface UserAnnotation{
int value() default 0;
}
To apply Single-Value Annotation
Let's see an example to apply the Single Value annotation, given below.
@UserAnnotation(value=30)
The value can be anything.
Mulit-Value Annotation
An annotation, which has more than one method is called as a Multi-Value annotation.
For example
@interface UserAnnotation{
int value1();
String value2();
String value3();
}
}
We can give the default value also.
For example
@interface UserAnnotation{
int value1() default 1;
String value2() default "";
String value3() default "xyz";
}
To apply Multi-Value Annotation
Let's see an example to apply the multi-value annotation, given below.
@MyAnnotation(value1=132,value2="David James",value3="Newyork")
Built-in Annotations, which are used in custom annotations are,
@Target
@Target annotation is used to specify at which type, the annotation is used.
The java.lang.annotation.ElementType enum creates several constants to specify the type of the element, where annotation is to be applied like TYPE, METHOD and FIELD etc.
The constants of ElementType enum
Element Types | Annotation can be applied |
TYPE | This can be applied on the class, interface or enumeration |
FIELD | This can be applied on the fields |
METHOD | This can be applied on the fields |
CONSTRUCTOR | This can be applied on the constructors |
LOCAL_VARIABLE | This can be applied on the local variables |
ANNOTATION_TYPE | This can be applied on annotation type |
PARAMETER | This can be applied on the parameter |
Let’s see an example to specify annotation for a class, given below.
@Target(ElementType.TYPE)
@interface UserAnnotation{
int value1();
String value2();
}
Let’s see an example to specify annotation for a class, methods or fields, given below.
@Target({ElementType.TYPE, ElementType.FIELD, ElementType.METHOD})
@interface UserAnnotation{
int value1();
String value2();
}
@Retention
@Retention annotation is used to indicate what level of annotation will be available.
RetentionPolicy | Availability |
RetentionPolicy.SOURCE | It refers to the source code and discarded during the compilation and It will not be presented in the compiled class. |
RetentionPolicy.CLASS | It refers to the .class file and available to Java compiler but not to JVM and It is included in the class file. |
RetentionPolicy.RUNTIME | It refers to the runtime, available to Java compiler and JVM. |
Let’s see an example to specify the RetentionPolicy, given below.
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
@interface UserAnnotation{
int value1();
String value2();
}
Let's see an example of creating, applying and accessing annotation, given below.
Code
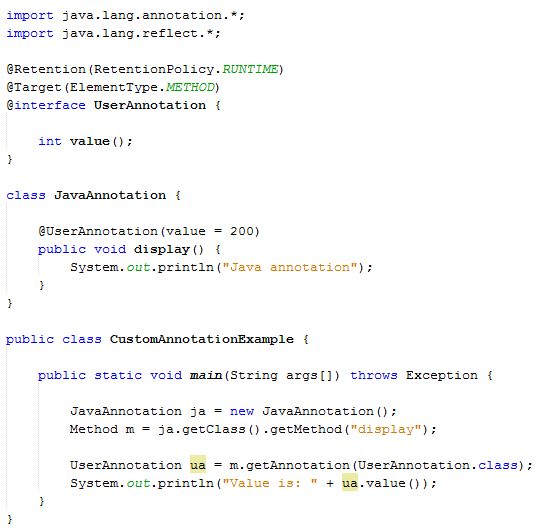
- import java.lang.annotation.*;
- import java.lang.reflect.*;
- @Retention(RetentionPolicy.RUNTIME)
- @Target(ElementType.METHOD)
- @interface UserAnnotation {
- int value();
- }
- class JavaAnnotation {
- @UserAnnotation(value = 200)
- public void display() {
- System.out.println("Java annotation");
- }
- }
- public class CustomAnnotationExample {
- public static void main(String args[]) throws Exception {
- JavaAnnotation ja = new JavaAnnotation();
- Method m = ja.getClass().getMethod("display");
- UserAnnotation ua = m.getAnnotation(UserAnnotation.class);
- System.out.println("Value is: " + ua.value());
- }
- }
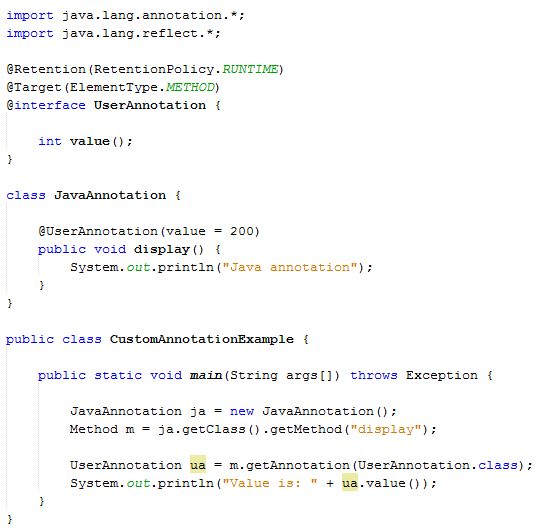
Output
Built-in annotations are used in real scenario
Java programmers only need to apply annotation. They don’t need to create and access annotation. Creating and accessing annotation is done by the implementation provider. On behalf of the annotation, the compiler or JVM does some additional operations.
@Inherited
Annotations are not inherited to sub classes by default. The @Inherited annotation marks the annotation to be inherited to the sub classes.
@Inherited
@interface ForEveryone { }// it will be available to subclass also
@interface ForEveryone { }
class Parentclass{}
class Childclass extends Parentclass{}
@Documented
The @Documented tags the annotation for the inclusion in the documentation.
Summary
Thus, we learnt that a custom annotation means user-defined annotations. These annotations are easy to create, use and also learnt how to create a custom annotation in Java.