Introduction Of Collections In Java
Collections
Collection is a framework, which provides the structural design to store and manipulate the group of the objects.
All the operations, which we perform on a data such as searching, sorting, insertion, manipulation, deletion can be performed by collections in Java.
In other words, collection simply means a single unit of objects.
Collection represents a single unit of objects, which means a group in Java.
What is framework?
- It provides readymade architecture.
- It represents set of classes and interface.
- It is optional.
What is collection framework?
In Java, collections framework is a collection of interfaces and classes, which helps to store and process the data efficiently. It has several useful classes, which have a lot of useful functions, which makes a programmer task very easy.
In Java,collection framework provides many interfaces likeSet, List, Queueetc and classes like ArrayList, Vector, LinkedList, HashSet, TreeSet etc.
It represents a unified structural design to store and manipulate the group of objects. It has following,
- Interfaces and its classes
- Algorithm
Hierarchy of Collection Framework
Let’s see the hierarchy, given below.
It is java.util package, which has all the classes and interfaces for the collection framework.
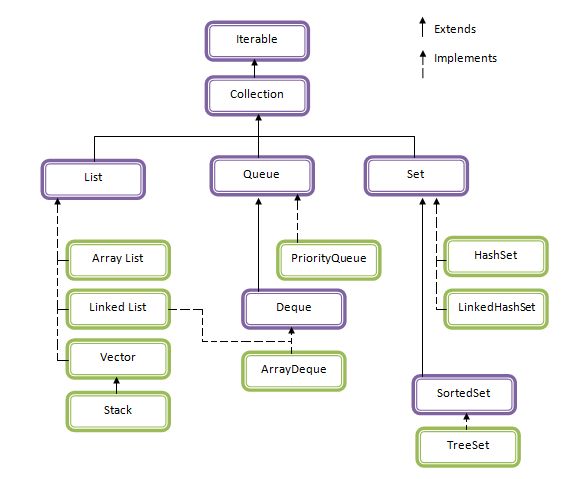
Methods of collection interface
public boolean add(Object element)
This method is used to insert an element in this collection.
public booleanaddAll(Collection c)
This method is used to insert the specified collection elements in the invoking collection.
public boolean remove(Object element)
This method is used to delete an element from this collection.
public booleanremoveAll(Collection c)
This method is used to delete all the elements of the specified collection from the invoking collection.
public booleanretainAll(Collection c)
This method is used to delete all the elements of invoking the collection except the specified collection.
public int size()
This method is used to return the total number of elements in the collection.
public void clear()
This method is used to remove the total no of elements from the collection.
public boolean contains(Object element)
This method is used to search an element.
public booleancontainsAll(Collection c)
This method is used to search the specified collection in this collection.
public Iterator iterator()
This method is used to return an iterator.
public Object[] toArray()
This method is used to convert the collection into an array.
public booleanisEmpty()
This method is used to check, if the collection is empty or not.
public boolean equals(Object element)
This method is used to match two collection.
public inthashCode()
This method is used to return the hashcode number for collection.
Iterator interface
Iterator interface is mainly used to iterate the elements in forward direction only in Java.
Methods of Iterator interface
publicbooleanhasNext()
This method is used to return true, if the iterator has more elements.
public object next()
This method is used to return the element and move the cursor pointer to the next element.
public void remove()
This method is used to remove the last elements, returned by the iterator.
Summary
Thus, we learnt that the collection is a framework, which provides the structural design to store and manipulate the group of objects and also its important methods in Java.