Deadlock In Java
Deadlock
In Java, deadlock is a part of multithreading. Deadlock can arise in a situation when a thread is waiting for an object lock, that is obtained by another thread and second thread is waiting for an object lock that is obtained by first thread. As both threads are waiting for each other to free the lock, the condition is called a deadlock.
Let’s see an example, given below.
Code
- public class DeadLock {
- public static void main(String[] args) {
- final String s1 = "James";
- final String s2 = "Harry";
- Thread t1 = new Thread() {
- public void run() {
- synchronized(s1) {
- System.out.println(" s1 locked ");
- try {
- Thread.sleep(1500);
- } catch (Exception e) {}
- synchronized(s2) {
- System.out.println(" s2 locked ");
- }
- }
- }
- };
- Thread t2 = new Thread() {
- public void run() {
- synchronized(s2) {
- System.out.println(" s2 locked... ");
- try {
- Thread.sleep(1500);
- } catch (Exception e) {}
- synchronized(s1) {
- System.out.println(" s1 locked... ");
- }
- }
- }
- };
- t1.start();
- t2.start();
- }
- }
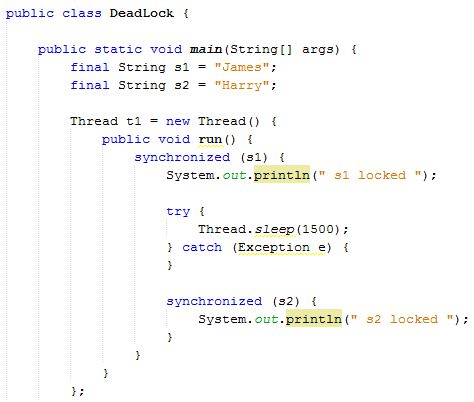
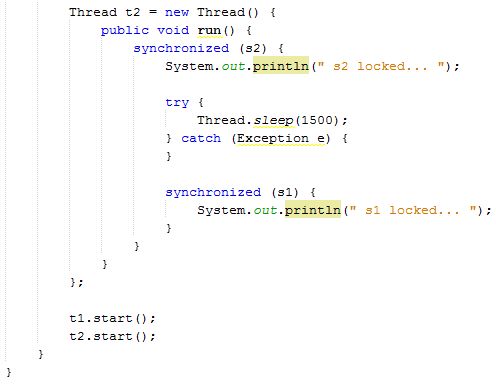
Output
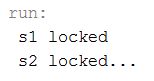
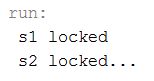
Summary
Thus, we learnt that In Java, deadlock is a part of multithreading and it can arise in a situation when a thread is waiting for an object lock.