Display Graphics In Swing Java
Display Graphics in Swing
In Java, java.awt.Graphics class gives many methods for the graphics programming.
Methods of Graphics class
public abstract void drawString(String str, int x, int y)
This method is used to draw the specific string.
public void drawRect(int x, int y, int width, int height)
This method is used to draw a rectangle with the particular width and height.
public abstract void fillRect(int x, int y, int width, int height)
This method is used to fill rectangle with the default color, particular width and height.
public abstract void drawOval(int x, int y, int width, int height)
This method is used to draw oval with the particular width and height.
public abstract void fillOval(int x, int y, int width, int height)
This method is used to fill oval with the default color, particular width and height.
public abstract void drawLine(int x1, int y1, int x2, int y2)
This method is used to draw the line between the points(x1, y1) and (x2, y2).
public abstract boolean drawImage(Image img, int x, int y, ImageObserver observer)
This method is used draw the specific image.
public abstract void drawArc(int x, int y, int width, int height, int startAngle, int arcAngle)
This method is used to draw a circular or elliptical arc.
public abstract void fillArc(int x, int y, int width, int height, int startAngle, int arcAngle)
This method is used to fill a circular or elliptical arc.
public abstract void setColor(Color c)
This method is used to set the graphics current color to the particular color.
public abstract void setFont(Font font)
This method is used to set the graphics current font to the specific font.
Let’s see an example of display graphics in Swing, given below.
Code
- import java.awt.*;
- import javax.swing.JFrame;
- public class DisplayGraphicsExample extends Canvas {
- public void paint(Graphics g) {
- g.drawString("Java Swing", 50, 50);
- setBackground(Color.WHITE);
- g.fillRect(120, 40, 100, 80);
- setForeground(Color.GREEN);
- }
- public static void main(String[] args) {
- DisplayGraphicsExample dge = new DisplayGraphicsExample();
- JFrame jf = new JFrame();
- jf.add(dge);
- jf.setSize(400, 400);
- jf.setVisible(true);
- }
- }
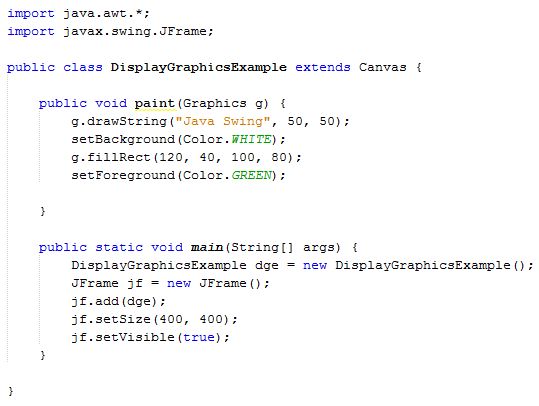
Output
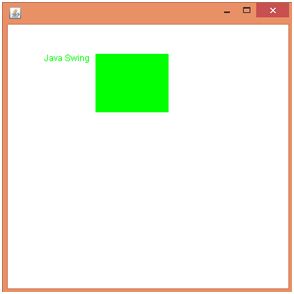
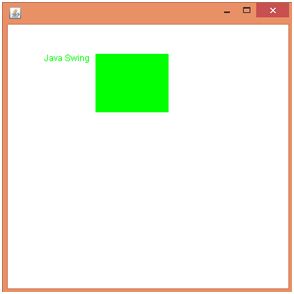
Summary
Thus, we learnt that the java.awt.Graphics class gives many methods for the graphics programming and also learnt how to display graphics in Swing Java.