Display Image In Swing Java
Display Image
In Swing Java, we can use the method drawImage() of Graphics class to display image.
Method drawImage()
public abstract boolean drawImage(Image img, int x, int y, ImageObserver observer)
This method is used to draw the specific image.
Let’s see an example of display image in Swing, given below.
Code
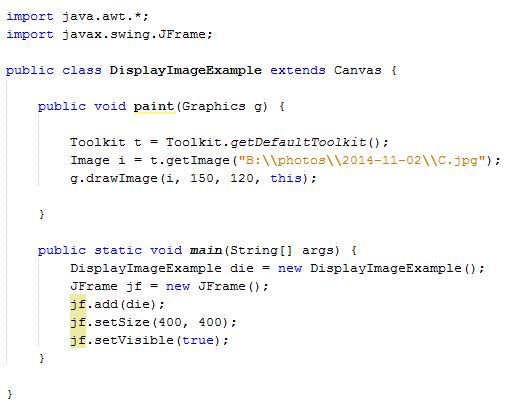
- import java.awt.*;
- import javax.swing.JFrame;
- public class DisplayImageExample extends Canvas {
- public void paint(Graphics g) {
- Toolkit t = Toolkit.getDefaultToolkit();
- Image i = t.getImage("B:\\photos\\2014-11-02\\C.jpg");
- g.drawImage(i, 150, 120, this);
- }
- public static void main(String[] args) {
- DisplayImageExample die = new DisplayImageExample();
- JFrame jf = new JFrame();
- jf.add(die);
- jf.setSize(400, 400);
- jf.setVisible(true);
- }
- }
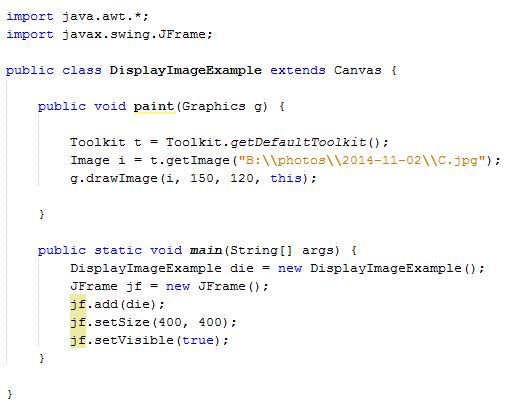
Output
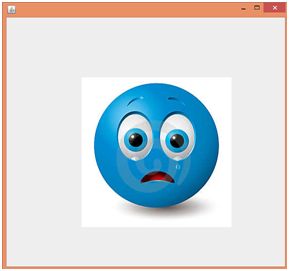
Summary
Thus, we learnt that we can use the method drawImage() of Graphics class to display an image and also learnt how to display an image in Swing Java.