Garbage Collection In Java
Garbage Collection
Garbage simply means unreferenced objects. It is a process of reclaiming the runtime unused memory automatically.
In other words, we can say that it is an easy way to destroy the unused objects.
In Java, it is performed automatically. Thus, Java provides better memory management.
Advantages of Garbage Collection
- It makes memory efficient.
- It is a part of JVM and work automatically. Thus, we don't need to make extra efforts (save time).
We can an object be unreferenced in many ways. Such as,
- By nulling a reference.
- Car c=new Car();
- c=null;
- By assigning a reference to another.
- Bike b1=new Bike();
- Bike b2=new Bike();
- b1=b2; // b1 is available for garbage collection
- By annonymous object.
- new Student();
finalize() method
The finalize() method is called each time before the object is garbage collected. It can be used to perform cleanup processing and it is defined in Object class like,
protected void finalize(){}
Usage of finalize() method
The garbage collector collects only those objects, which are created by new keyword. Thus, if we create any object without new keyword, we need to use finalize method to perform cleanup processing.
gc() method
The gc() method is used to call the garbage collector to perform cleanup processing. The gc() method is defined in System and Runtime classes like,
public static void gc(){}
Garbage collection is performed with a daemon thread called garbage collector. This thread calls the finalize() method before the object is garbage collected.
Let’s see an example of the garbage collection, given below.
Code
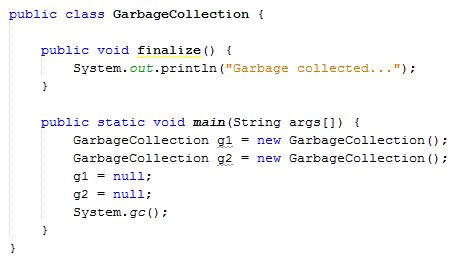
- public class GarbageCollection {
- public void finalize() {
- System.out.println("Garbage collected...");
- }
- public static void main(String args[]) {
- GarbageCollection g1 = new GarbageCollection();
- GarbageCollection g2 = new GarbageCollection();
- g1 = null;
- g2 = null;
- System.gc();
- }
- }
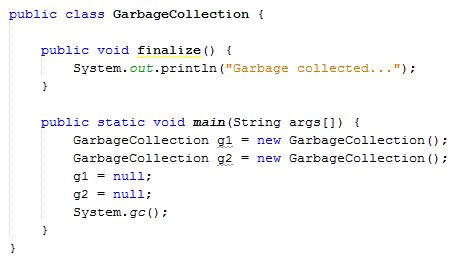
Output
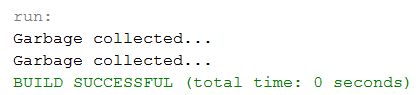
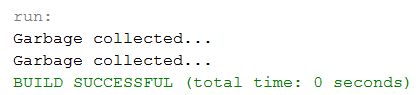
Summary
Thus, we learnt that garbage collection is a process of reclaiming the runtime unused memory automatically and also learnt its advantages in Java.