Introduction Of Java Regex
Java Regex
Regular expressions or Java Regex is an API to define the string patterns, which can be used to search, manipulate and edit a text.
Java provides java.util.regex package for the pattern matching with the regular expressions.
In other words, we can say that a regular expression is a special sequence of characters, which helps us to match or find other strings, using a specialized syntax held in a pattern and it is used to search, edit, or manipulate the text and data.
java.util.regex package
It provides three classes and one interface for the regular expressions. The Matcher and Pattern classes are generally used in Java regular expression.
- Matcher class
- Pattern class
- PatternSyntaxException class
- MatchResult interface
Matcher class of regular expression
It implements MatchResult interface and it is a Regex engine, which is used to perform the match operations on a character sequence.
Methods
boolean matches()
This method tests whether the regular expression matches the pattern.
boolean find()
This method finds the next expression that matches the pattern.
boolean find(int start)
This method finds the next expression that matches the pattern from the given start number.
String group()
This method returns the matched subsequence.
int start()
This method returns the starting index of the matched subsequence.
int end()
This method returns the ending index of the matched subsequence.
int groupCount()
This method returns the total number of the matched subsequence.
Pattern class of regular expression
It is the compiled version of a regular expression and is used to define a pattern for Regex engine.
Methods
static Pattern compile(String regex)
This method is used to compile the given Regex and return the instance of the pattern.
Matcher matcher(CharSequence input)
This method is used to create a matcher, which matches the given input with the pattern.
static boolean matches(String regex, CharSequence input)
It works as the combination of compile and matcher methods. It compiles the regular expression and matches the particular input with the pattern.
String[] split(CharSequence input)
This method is used to split the given input string around matches of the given pattern.
String pattern()
This method is used to return Regex pattern.
Regex Character classes
Character Classes
[abc]
a, b, c (Simple Class)
[^abc]
Any character except for a, b, c (Negation)
[a-zA-Z]
a through z , A through Z, inclusive (Range)
[a-d[m-p]]
a through d, m through p: [a-dm-p] (Union)
[a-z&&[def]]
d, e, f (Intersection)
[a-z&&[^bc]]
a through z, except for b and c: [ad-z] (Subtraction)
[a-z&&[^m-p]]
a through z, not m through p: [a-lq-z](Subtraction)
Regex Quantifiers
The quantifiers identify the number of occurrences of a character.
Regex
X?
This X occurs once or not at all
X+
This X occurs once or more times
X*
This X occurs zero or more times
X{n}
This X occurs n times only
X{n,}
This X occurs n or more times
X{y,z}
This X occurs at least y times but less than z times
Regex Metacharacters
The regular expression metacharacters works as a short code.
Regex
.
All character (may or may not match terminator)
\d
All digits, short of [0-9]
\D
All non-digit, short for [^0-9]
\s
All whitespace character, short for [\t\n\x0B\f\r]
\S
All non-whitespace character, short for [^\s]
\w
All word character, short for [a-zA-Z_0-9]
\W
All non-word character, short for [^\w]
\b
A word boundary line
\B
A non word boundary line
Examples of Regular Expressions
Let’s see an example1 of regular expressions, given below.
There are three ways to write Regex in Java.
Code
- import java.util.regex.*;
- public class RegularExpressionEx {
- public static void main(String args[]) {
- Pattern p = Pattern.compile(".o"); //1st way to write code
- Matcher m = p.matcher("to");
- boolean m1 = m.matches();
- boolean m2 = Pattern.compile(".c").matcher("Dick").matches(); //2nd way to write code
- boolean m3 = Pattern.matches("..m", "Tom"); //3rd way to write code
- System.out.println(m1 + " " + m2 + " " + m3);
- }
- }
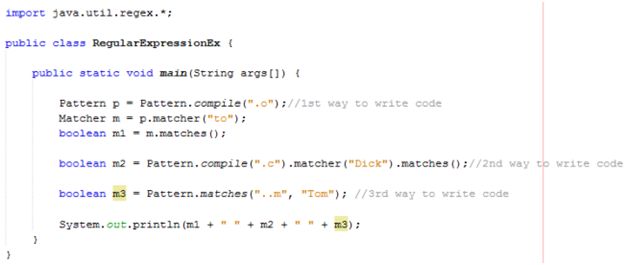
Output

Let’s see an example2 of regular expressions, given below.
Code
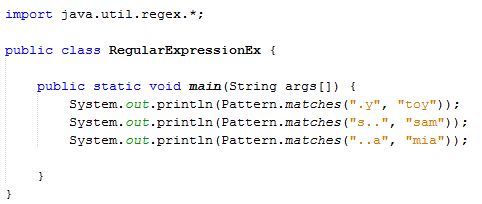
- import java.util.regex.*;
- public class RegularExpressionEx {
- public static void main(String args[]) {
- System.out.println(Pattern.matches(".y", "toy"));
- System.out.println(Pattern.matches("s..", "sam"));
- System.out.println(Pattern.matches("..a", "mia"));
- }
- }
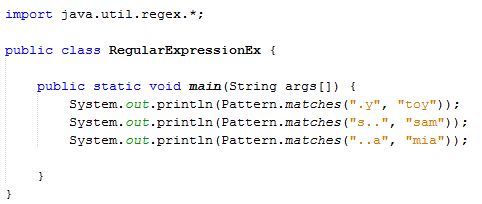
Output
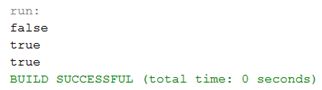
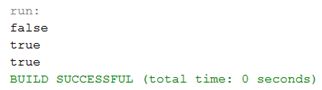
Let’s see an example3 of regular expression Character classes, given below.
Code
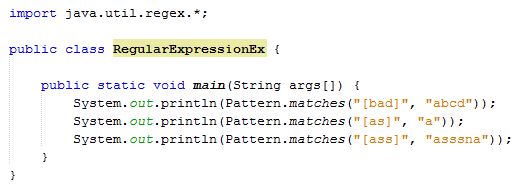
- import java.util.regex.*;
- public class RegularExpressionEx {
- public static void main(String args[]) {
- System.out.println(Pattern.matches("[bad]", "abcd"));
- System.out.println(Pattern.matches("[as]", "a"));
- System.out.println(Pattern.matches("[ass]", "asssna"));
- }
- }
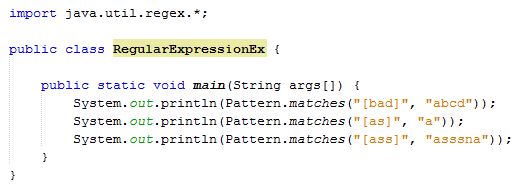
Output
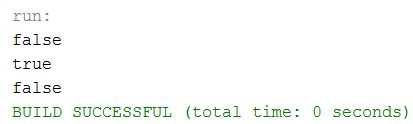
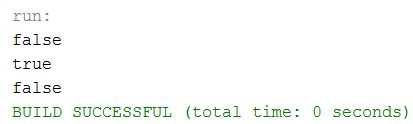
Let’s see an example4 regular expression Character Classes with quantifiers, given below.
Code
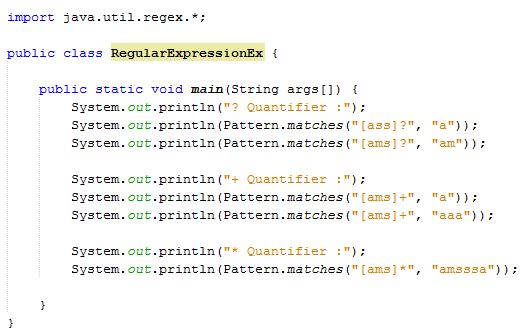
- import java.util.regex.*;
- public class RegularExpressionEx {
- public static void main(String args[]) {
- System.out.println("? Quantifier :");
- System.out.println(Pattern.matches("[ass]?", "a"));
- System.out.println(Pattern.matches("[ams]?", "am"));
- System.out.println("+ Quantifier :");
- System.out.println(Pattern.matches("[ams]+", "a"));
- System.out.println(Pattern.matches("[ams]+", "aaa"));
- System.out.println("* Quantifier :");
- System.out.println(Pattern.matches("[ams]*", "amsssa"));
- }
- }
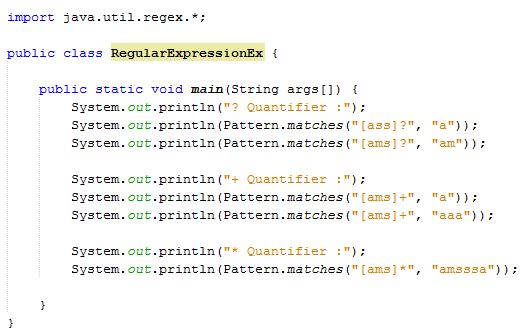
Output
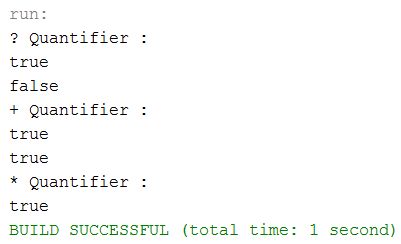
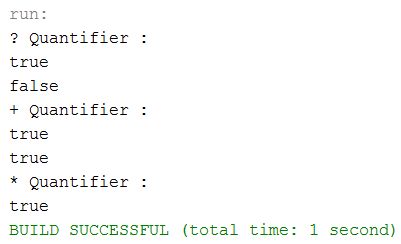
Let’s see an example5 regular expression Metacharacters, given below.
Code
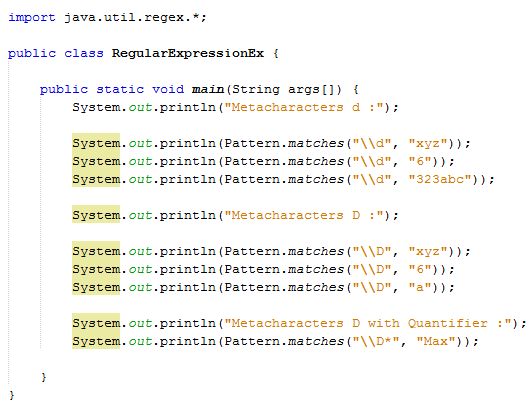
- import java.util.regex.*;
- public class RegularExpressionEx {
- public static void main(String args[]) {
- System.out.println("Metacharacters d :");
- System.out.println(Pattern.matches("\\d", "xyz"));
- System.out.println(Pattern.matches("\\d", "6"));
- System.out.println(Pattern.matches("\\d", "323abc"));
- System.out.println("Metacharacters D :");
- System.out.println(Pattern.matches("\\D", "xyz"));
- System.out.println(Pattern.matches("\\D", "6"));
- System.out.println(Pattern.matches("\\D", "a"));
- System.out.println("Metacharacters D with Quantifier :");
- System.out.println(Pattern.matches("\\D*", "Max"));
- }
- }
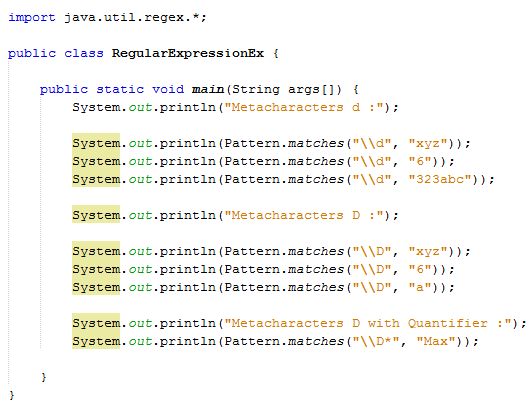
Output
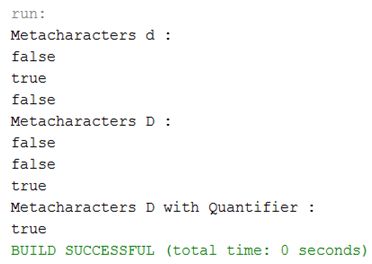
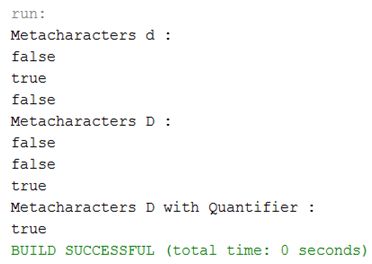
Let’s see an example6, given below of a regular expression, which accepts alpha numeric characters only.
Code
- import java.util.regex.*;
- public class RegularExpressionEx {
- public static void main(String args[]) {
- System.out.println(Pattern.matches("[a-zA-Z0-9]{6}", "jack2"));
- System.out.println(Pattern.matches("[a-zA-Z0-9]{6}", "ddavid52"));
- System.out.println(Pattern.matches("[a-zA-Z0-9]{6}", "Harry2"));
- System.out.println(Pattern.matches("[a-zA-Z0-9]{6}", "John$20"));
- }
- }
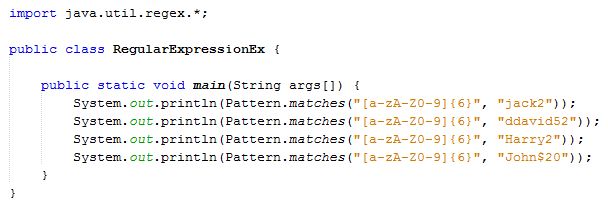
Output
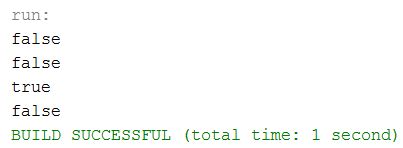
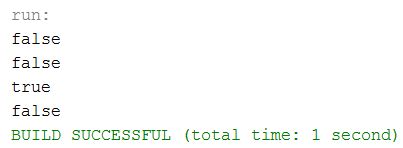
Let’s see an example7, given below of a regular expression, which accepts 10 digit numeric characters.
Code
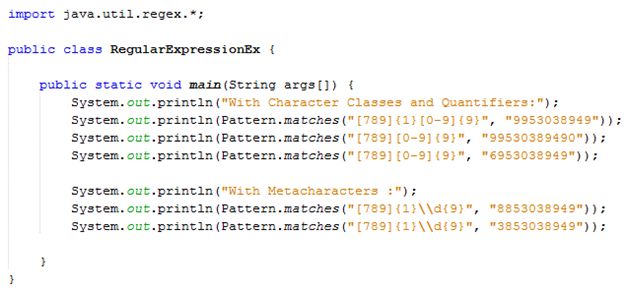
- import java.util.regex.*;
- public class RegularExpressionEx {
- public static void main(String args[]) {
- System.out.println("With Character Classes and Quantifiers:");
- System.out.println(Pattern.matches("[789]{1}[0-9]{9}", "9953038949"));
- System.out.println(Pattern.matches("[789][0-9]{9}", "99530389490"));
- System.out.println(Pattern.matches("[789][0-9]{9}", "6953038949"));
- System.out.println("With Metacharacters :");
- System.out.println(Pattern.matches("[789]{1}\\d{9}", "8853038949"));
- System.out.println(Pattern.matches("[789]{1}\\d{9}", "3853038949"));
- }
- }
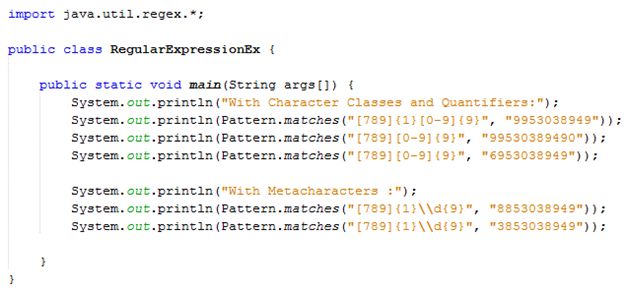
Output
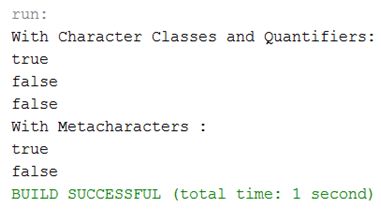
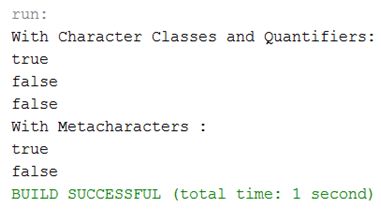
Summary
Thus, we learnt, Regular expressions or Java Regex is an API to define the string patterns, which can be used to search, manipulate, edit a text and also learnt, how to use Regex in Java.