Hashtable Class In Java
Hashtable Class
In Java, Hashtable is an array of list and that list is known as a bucket. The position of the bucket is identified by invoking the hashcode() method. A Hashtable holds the values, based on the key. It implements the map interface and extends Dictionary class. It holds the unique elements only and may or may not have any null key or value. It is a synchronized class.
Constructor of Hashtable Class
Hashtable( )
This constructor is the default constructor of the hash table and it is used to instantiate the Hashtable class.
Hashtable(int size)
This constructor is used to accept an integer parameter and creates a hash table, which has an initial size, specified by the integer value size.
Hashtable(int size, float fillRatio)
This constructor is used to create a hash table, which has an initial size, specified by the size and a fill ratio, specified by fillRatio.
Hashtable(Map<? extends K, ? extends V> t)
This constructor is used to construct a Hashtable with the given mappings.
Methods of Hashtable Class
void clear( )
This method is used to reset and empty the hash table.
Object clone( )
This method is used to return a duplicate of the calling object.
boolean contains(Object value)
This method is used to return true, if some value is equal to the value exists within the hash table. It returns false, if the value isn't found.
boolean containsKey(Object key)
This method is used to return true, if some key is equal to key, which exists within the hash table. It returns false, if the key isn't found.
boolean containsValue(Object value)
This method is used to return true, if some value is equal to value, which exists within the hash table. It returns false, if the value isn't found.
Enumeration elements( )
This method is used to return an enumeration of the values contained in the hash table.
Object get(Object key)
This method is used to returns the object, which contains the value associated with the key. If the key is not in the hash table, a null object is returned.
boolean isEmpty( )
This method is used to return true, if the hash table is empty and returns false, if it contains at least one key.
Enumeration keys( )
This method is used to return an enumeration of the keys contained in the hash table.
Object put(Object key, Object value)
This method is used to insert a key and a value into the hash table. It returns null, if the key isn't already in the hash table; returns the previous value, associated with the key, if the key is already in the hash table.
void rehash( )
This method is used to increase the size of the hash table and rehashes all its keys.
Object remove(Object key)
This method is used to remove the key and its value. It returns the value associated with the key. If key is not in the hash table, a null object is returned.
int size( )
This method is used to return the number of entries in the hash table.
String toString( )
This method is used to return the string equivalent of a hash table.
Let’s see an example, given below.
Code
- import java.util.*;
- public class CollectionsExample {
- public static void main(String args[]) {
- Hashtable < Integer, String > ht = new Hashtable < Integer, String > ();
- ht.put(236, "Mia");
- ht.put(456, "Marria");
- ht.put(859, "Harry");
- ht.put(147, "Lucy");
- for (Map.Entry m: ht.entrySet()) {
- System.out.println(m.getKey() + " " + m.getValue());
- }
- }
- }
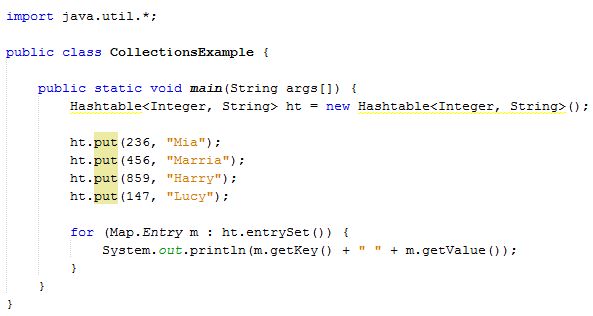
Output
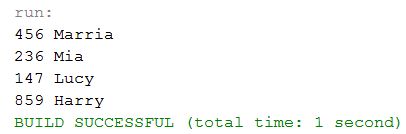
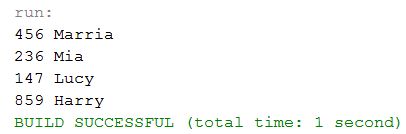
Summary
Thus, we learnt that Java Hashtable is an array of the list and the list is known as a bucket. The position of the bucket is identified by invoking the hashcode() method. A Hashtable holds values are based on the key and also learnt how to create it in Java.