Try Catch Block In Java
Java try block
In Java, try block holds a block of program code within which an exception might occur. A try block is always followed by a catch block, which handles the exception that occurs in a related try block. A try block must be followed by a catch or finally block or both.
In other words, we can say that try block is used to enclose the code that might throw an exception. It should be used within the method. A try block must be followed by either catch or finally block in Java exception handling.
Syntax of try block
try{
//code that may throw an exception
}
Java catch block
A catch block must be related with a try block. The corresponding catch block executes if an exception of a particular type occurs within the try block. For example, if a NullPointerException occurs in a try block, the statements is enclosed in catch block for NullPointerException executes.
In simple words, catch block is used to handle the exception. It must be used after the try block only and we can use multiple catch blocks with a single try.
Syntax of try-catch block
try {
//code that may throw an exception
} catch (exception (type) e (object))
{
//error handling code
}
Let’s see an example of Exception.
Code
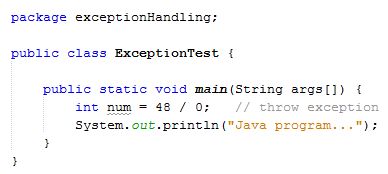
- package exceptionHandling;
- public class ExceptionTest {
- public static void main(String args[]) {
- int num = 48 / 0; // throw exception
- System.out.println("Java program...");
- }
- }
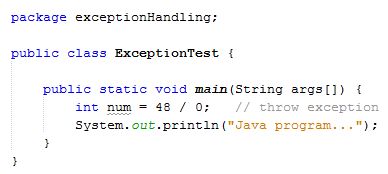
Output


In the example, mentioned above, print statement “Java program…” is not executed because of ArithmeticException. Thus, all the code after exception will not be executed.
Now, let’s see the example of an exception handling.
Let's see the solution of the problem, mentioned above, by Java try-catch block.
Code
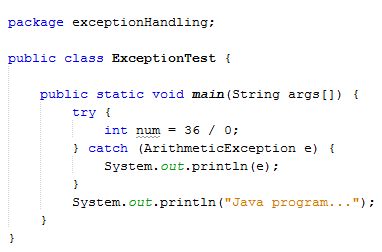
- package exceptionHandling;
- public class ExceptionTest {
- public static void main(String args[]) {
- try {
- int num = 36 / 0;
- } catch (ArithmeticException e) {
- System.out.println(e);
- }
- System.out.println("Java program...");
- }
- }
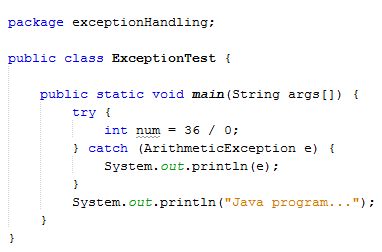
Output
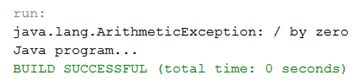
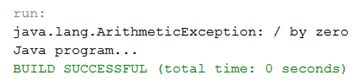
Now, in the example, mentioned above, rest code is executed i.e. Java program... statement is printed.
Internal working of Java try-catch block
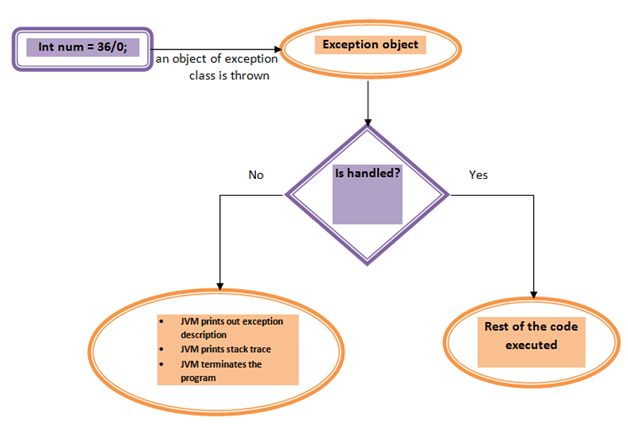
In the flowchart, mentioned above, first JVM checks that whether the exception is handled or not. If an exception is not handled, JVM provides a default exception handler, which performs,
- JVM prints out exception description.
- JVM prints the stack trace.
- JVM causes the program to terminate.
If an exception is handled by the programmer, the normal flow of the Application is maintained and rest of the code is executed normally.
Summary
Thus, we learnt that try block is used to enclose the code that might throw an exception and catch block is used to handle the exception. It must be used after the try block and we also learnt internal working of Java try-catch block in Java.