BufferedOutputStream And BufferedInputStream In Java
BufferedOutputStream class
BufferedOutputStream class is used as an internal buffer to store the data in Java.
It is more efficiency than to write the data directly into a stream and it makes the operation fast.
Let’s see an example, given below.
Code
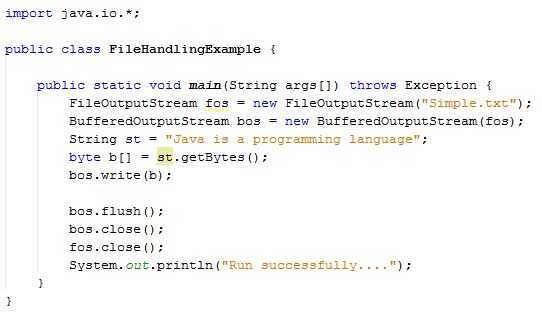
- import java.io.*;
- public class FileHandlingExample {
- public static void main(String args[]) throws Exception {
- FileOutputStream fos = new FileOutputStream("Simple.txt");
- BufferedOutputStream bos = new BufferedOutputStream(fos);
- String st = "Java is a programming language";
- byte b[] = st.getBytes();
- bos.write(b);
- bos.flush();
- bos.close();
- fos.close();
- System.out.println("Run successfully....");
- }
- }
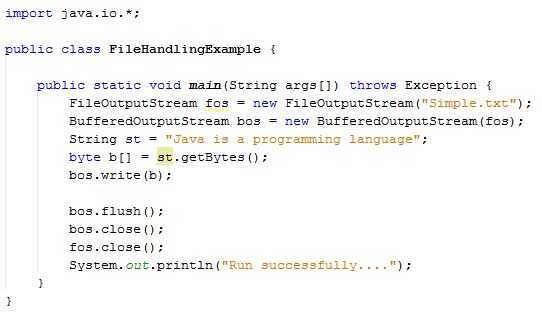
Output


In the example, shown above, we write some text in the BufferedOutputStream object, which is linked to the FileOutputStream object. The flush() method flushes the data of one stream and sends it into another but It is required, when we connect one stream to another.
BufferedInputStream class
To read information from the stream, BufferedInputStream class is used. It internally uses buffer which makes the performance fast.
Let's see an example, given below.
Code
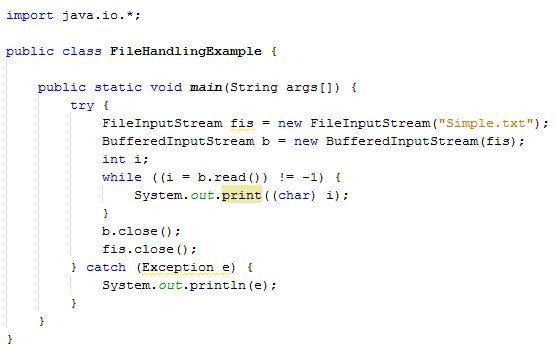
- import java.io.*;
- public class FileHandlingExample {
- public static void main(String args[]) {
- try {
- FileInputStream fis = new FileInputStream("Simple.txt");
- BufferedInputStream b = new BufferedInputStream(fis);
- int i;
- while ((i = b.read()) != -1) {
- System.out.print((char) i);
- }
- b.close();
- fis.close();
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
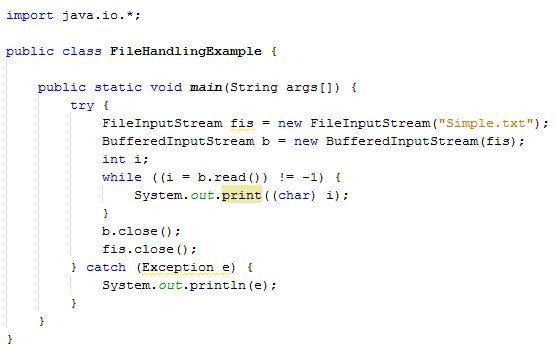
Output

Summary
Thus, we learnt that the BufferedOutputStream class is use as an internal buffer to store the data and to read information from the stream; BufferedInputStream class is used and also learnt how to create it in Java.