Comparable Interface In Java
Comparable Interface
In Java, comparable interface orders the objects of user-defined class. This interface is found in java.lang package and contains only one compareTo(Object) method. It provides the single sorting sequence only i.e. we can sort the elements, based on the single data member only.
Method
public int compareTo(Object obj)
This method is used to compare the current object with the particular object.
In Java, String class and Wrapper classes implements the comparable interface by default. Thus, if we store the objects of string or wrapper classes in the list, set or map, it will be comparable by default.
Let’s see an example, given below.
Code
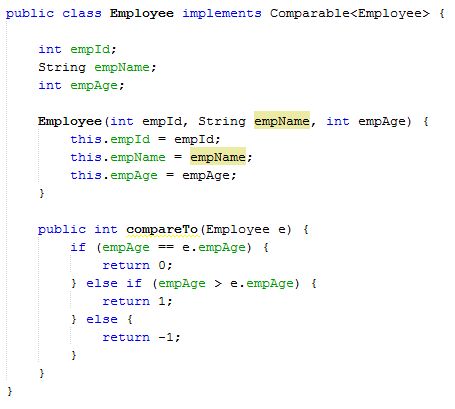
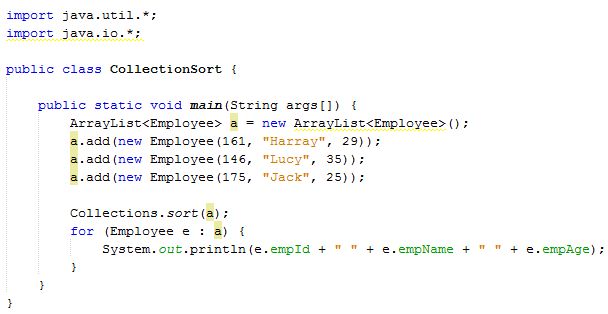
- public class Employee implements Comparable < Employee > {
- int empId;
- String empName;
- int empAge;
- Employee(int empId, String empName, int empAge) {
- this.empId = empId;
- this.empName = empName;
- this.empAge = empAge;
- }
- public int compareTo(Employee e) {
- if (empAge == e.empAge) {
- return 0;
- } else if (empAge > e.empAge) {
- return 1;
- } else {
- return -1;
- }
- }
- }
- import java.util.*;
- import java.io.*;
- public class CollectionSort {
- public static void main(String args[]) {
- ArrayList < Employee > a = new ArrayList < Employee > ();
- a.add(new Employee(161, "Harray", 29));
- a.add(new Employee(146, "Lucy", 35));
- a.add(new Employee(175, "Jack", 25));
- Collections.sort(a);
- for (Employee e: a) {
- System.out.println(e.empId + " " + e.empName + " " + e.empAge);
- }
- }
- }
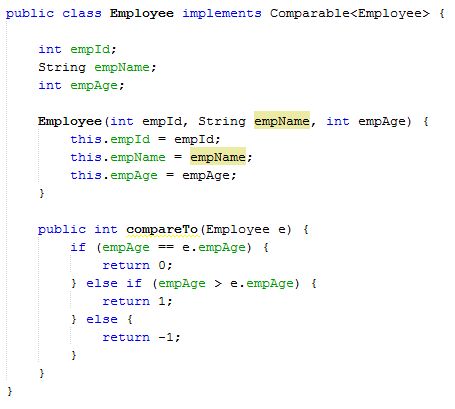
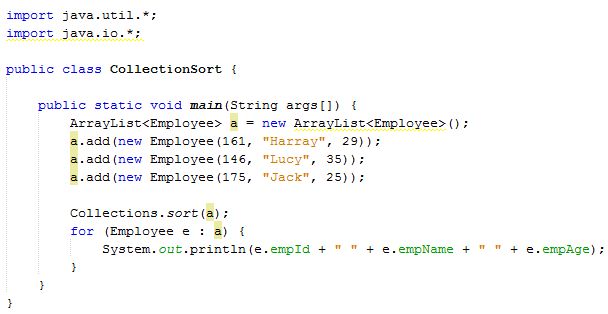
Output
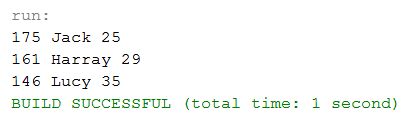
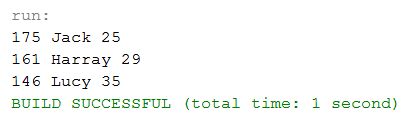
In the example, shown above of the comparable interface, which sorts the list elements on the basis of age.
Summary
Thus, we learnt that Java comparable interface orders the objects of user-defined class. This interface is found in java.lang package and contains only one compareTo(Object) method. We also learnt how to create it in Java.