join() Method In Java
join() method
The join() method is mainly used to hold the execution of currently running thread until the specified thread is dead.
In other words, we can say that join() method waits for a thread to die and It causes the presently running threads to stop until the thread it joins which finishes its entire task.
Syntax
public void join()throws InterruptedException
public void join(long milliseconds)throws InterruptedException
Let’s see an example of join() method.
Code
- public class JoinMethod extends Thread {
- public void run() {
- for (int i = 0; i <= 6; i++) {
- try {
- Thread.sleep(1000);
- } catch (Exception e) {
- System.out.println(e);
- }
- System.out.println(i);
- }
- }
- public static void main(String args[]) {
- JoinMethod t1 = new JoinMethod();
- JoinMethod t2 = new JoinMethod();
- JoinMethod t3 = new JoinMethod();
- t1.start();
- try {
- t1.join();
- } catch (Exception e) {
- System.out.println(e);
- }
- t2.start();
- t3.start();
- }
- }
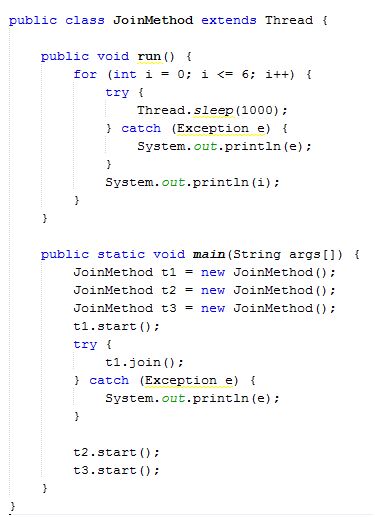
Output
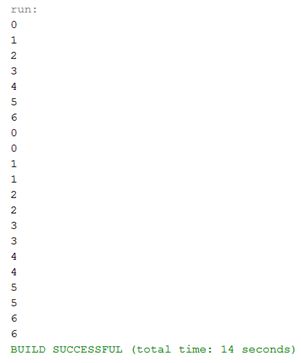
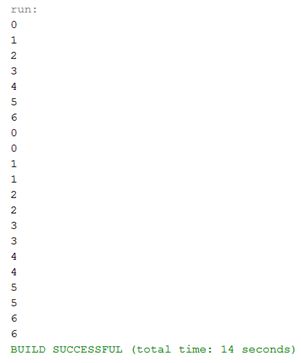
In the above example, we can observe that when t1 completes its task then t2 and t3 starts executing.
Let’s see an example of join(long miliseconds) method, given below.
Code
- public class JoinMethod extends Thread {
- public void run() {
- for (int i = 0; i <= 4; i++) {
- try {
- Thread.sleep(700);
- } catch (Exception e) {
- System.out.println(e);
- }
- System.out.println(i);
- }
- }
- public static void main(String args[]) {
- JoinMethod t1 = new JoinMethod();
- JoinMethod t2 = new JoinMethod();
- JoinMethod t3 = new JoinMethod();
- t1.start();
- try {
- t1.join(1000);
- } catch (Exception e) {
- System.out.println(e);
- }
- t2.start();
- t3.start();
- }
- }
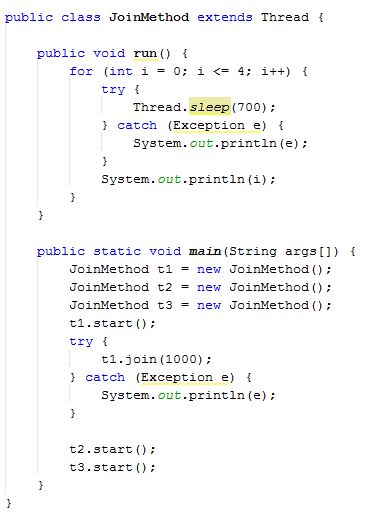
Output
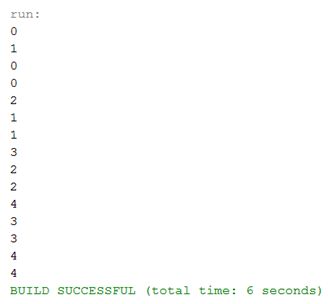
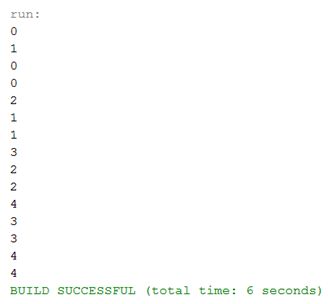
In the above example, t1 is completes its task for 1000 miliseconds then t2 and t3 starts executing.
Summary
Thus, we learnt, join() method is mainly used to hold the execution of currently running thread until the specified thread is dead and also learnt, how to use join() method in Java.