How To Retrieve File From Database
Retrieve file from Database
In JDBC, the PreparedStatement getClob() method is used to get the file information from the database.
Syntax of getClob method
public Clob getClob(int columnIndex){}
Example
CREATE TABLE "FILETABLE"
( "ID" NUMBER,
"NAME" CLOB
)
Let’s see an example, given below.
Code
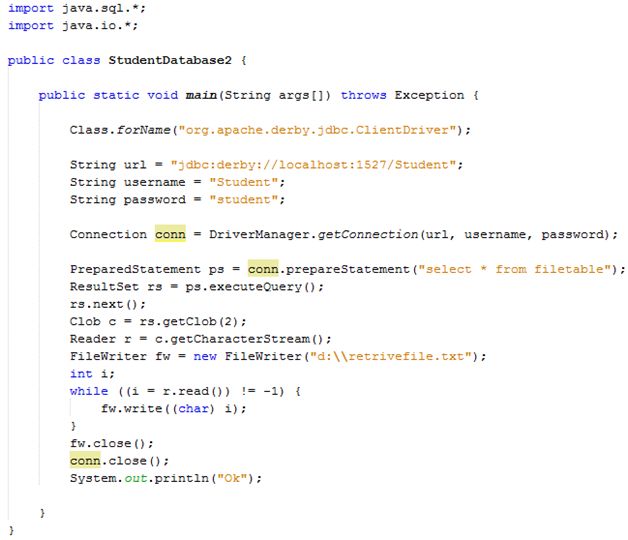
- import java.sql.*;
- import java.io.*;
- public class StudentDatabase2 {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- PreparedStatement ps = conn.prepareStatement("select * from filetable");
- ResultSet rs = ps.executeQuery();
- rs.next();
- Clob c = rs.getClob(2);
- Reader r = c.getCharacterStream();
- FileWriter fw = new FileWriter("d:\\retrivefile.txt");
- int i;
- while ((i = r.read()) != -1) {
- fw.write((char) i);
- }
- fw.close();
- conn.close();
- System.out.println("Ok");
- }
- }
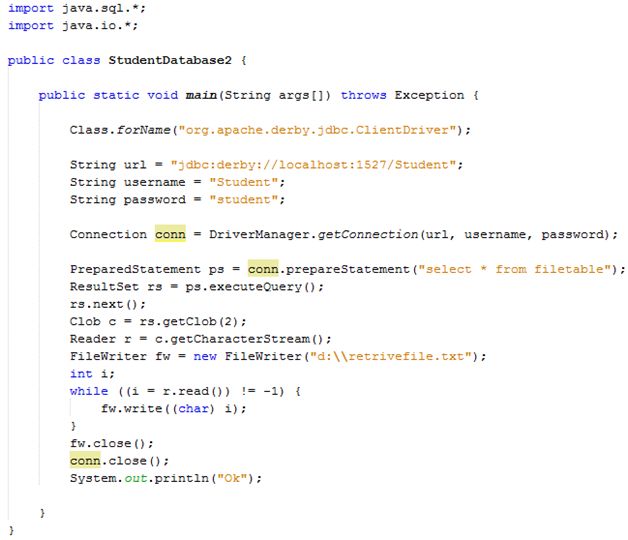
Output


Summary
Thus, we learnt, PreparedStatement getClob() method is used to get file information from the database and also learnt, how to retrieve the file from the database in Java.