Introduction Of Internationalization And Localization In Java
Internationalization and Localization
In Java, internationalization is shortened as I18N since there are total 18 characters between the first letter 'I' and the last letter 'N'. It is a mechanism to create such an Application, which can be adapted by the different languages and regions. It is one of the robust concepts of Java.
In Java, localization is shortened as I10N. Due to this, there are total 10 characters between the first letter 'L' and last letter 'N'. It is a mechanism to create such an Application, which can be adapted to a particular language and region by adding locale-specific text and component.
Let’s first understand the culturally dependent data, before starting the internationalization.
- Messages
- Dates
- Times
- Numbers
- Currencies
- Measurements
- Phone Numbers
- Postal Addresses
- Labels on GUI components etc.
Locale class
An object of Locale class shows a geographical or cultural region and this object can be used to get the locale specific information like country name, language, etc.
Fields of Locale class
There are many fields of Locale class, which are,
- public static final Locale ENGLISH
- public static final Locale FRENCH
- public static final Locale GERMAN
- public static final Locale ITALIAN
- public static final Locale JAPANESE
- public static final Locale KOREAN
- public static final Locale CHINESE
- public static final Locale SIMPLIFIED_CHINESE
- public static final Locale TRADITIONAL_CHINESE
- public static final Locale FRANCE
- public static final Locale GERMANY
- public static final Locale ITALY
- public static final Locale JAPAN
- public static final Locale KOREA
- public static final Locale CHINA
- public static final Locale PRC
- public static final Locale TAIWAN
- public static final Locale UK
- public static final Locale US
- public static final Locale CANADA
- public static final Locale CANADA_FRENCH
- public static final Locale ROOT
- Constructors of Locale class
Constructors of Locale class
Locale(String language)
Locale(String language, String country)
Locale(String language, String country, String variant)
Methods of Locale class
public static Locale getDefault()
This method is used to return the object of the current locale
public static Locale[] getAvailableLocales()
This method is used to return an array of the available locales.
public String getDisplayCountry()
This method is used to return the country name of the locale object.
public String getDisplayLanguage()
This method is used to return the language name of the locale object.
public String getDisplayVariant()
This method is used to return the variant code for this locale object.
public String getISO3Country()
This method is used to return the three letter abbreviation for the current locale's country.
public String getISO3Language()
This method is used to return the three letter abbreviation for the current locale's language.
Let’s see an example of Local class, given below.
Code
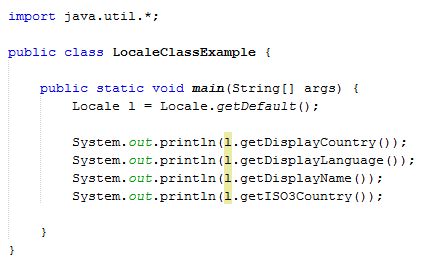
- import java.util.*;
- public class LocaleClassExample {
- public static void main(String[] args) {
- Locale l = Locale.getDefault();
- System.out.println(l.getDisplayCountry());
- System.out.println(l.getDisplayLanguage());
- System.out.println(l.getDisplayName());
- System.out.println(l.getISO3Country());
- }
- }
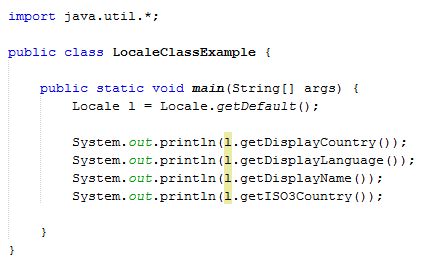
Output
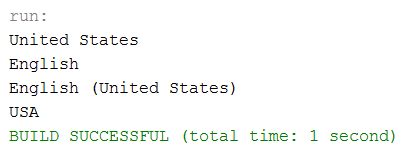
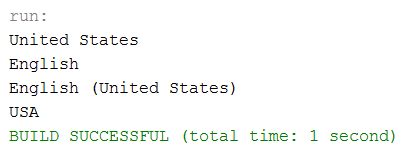
In the example, shown above, we display the information’s of the default locale and we can get the information’s about any particular locale.
Let’s see another example of Local class, given below.
Code
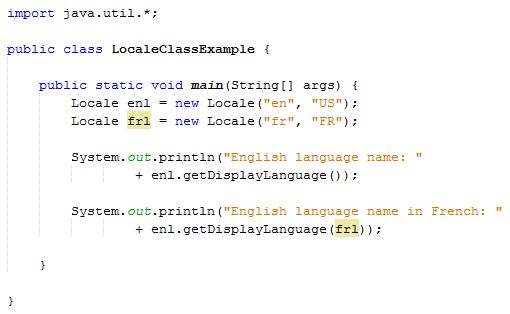
- import java.util.*;
- public class LocaleClassExample {
- public static void main(String[] args) {
- Locale enl = new Locale("en", "US");
- Locale frl = new Locale("fr", "FR");
- System.out.println("English language name: " +
- enl.getDisplayLanguage());
- System.out.println("English language name in French: " +
- enl.getDisplayLanguage(frl));
- }
- }
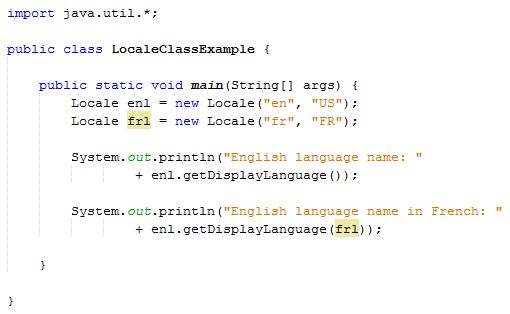
Output
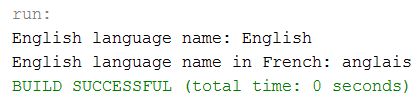
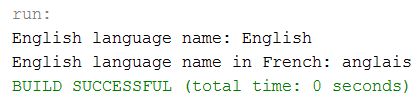
In the example, mentioned above, we display English language in different language.
Let’s see another example of Local class, given below.
Code
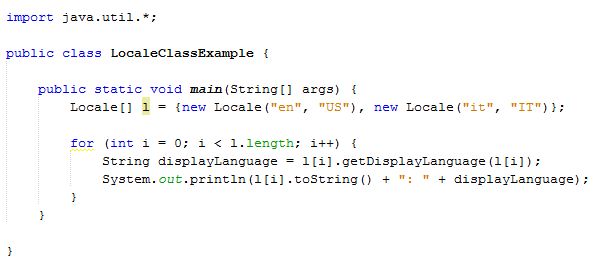
- import java.util.*;
- public class LocaleClassExample {
- public static void main(String[] args) {
- Locale[] l = {
- new Locale("en", "US"),
- new Locale("it", "IT")
- };
- for (int i = 0; i < l.length; i++) {
- String displayLanguage = l[i].getDisplayLanguage(l[i]);
- System.out.println(l[i].toString() + ": " + displayLanguage);
- }
- }
- }
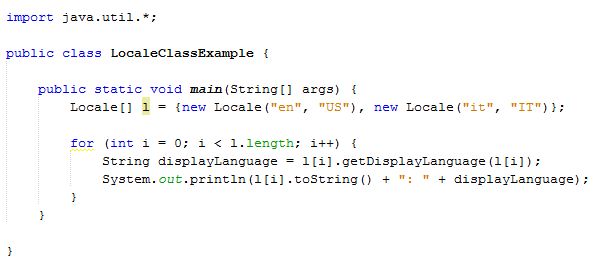
Output
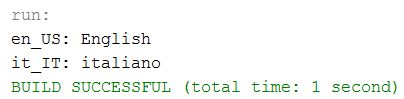
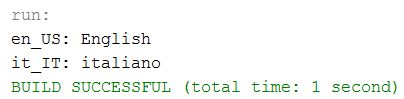
In the example, shown above, we display the language of many other locales.
Summary
Thus, we learnt that Internationalization is a mechanism to create an Application, which can be adapted to different languages and regions and localization is a mechanism to create an Application, which can be adapted to a particular language and region by adding locale-specific text, component and also learnt how we can use it in Java.