Default Methods In Java
Default Methods
A concept of default method implementation in interfaces introduces in Java 8. It has a capability to add for backward compatibility thus that old interfaces can be used to leverage the lambda expression capability of Java 8.
Such as, Collection interfaces don’t have ‘forEach’ method declaration. Thus, adding such method will just break the collection framework implementations. With the help of default method, Collection interface can contain a default implementation of ‘forEach’ method and the class implements these interfaces no need to implement the same.
Syntax
public interface Car {
default void display(){
System.out.println("Car is running fast");
}
}
Multiple Defaults
With the help of default functions in interfaces, there is an option that a class is implementing two interfaces with same default methods.
Explanation how to ambiguity can resolve.
- public interface Car {
- default void display() {
- System.out.println("Car is running smooth");
- }
- }
- public interface Bike {
- default void display() {
- System.out.println("Bike is running fast");
- }
- }
Now, create an own method which overrides the default implementation.
- public class Test implements Car, Bike {
- default void display() {
- System.out.println("Car and Bike both are best");
- }
- }
Then, call the default method of the specific interface using super.
- public class Test implements Car, Bike {
- default void display() {
- Car.super.display();
- }
- }
Static Default Methods
An interface can have static helper methods from Java 8 beyond.
- public interface Car {
- default void display() {
- System.out.println("Car is running fast");
- }
- static void run() {
- System.out.println("Running……");
- }
- }
Let’s see an example of Default Method.
Code
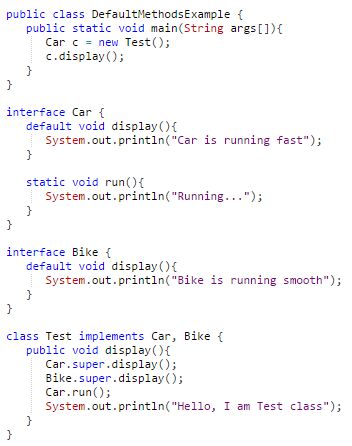
- public class DefaultMethodsExample {
- public static void main(String args[]) {
- Car c = new Test();
- c.display();
- }
- }
- interface Car {
- default void display() {
- System.out.println("Car is running fast");
- }
- static void run() {
- System.out.println("Running...");
- }
- }
- interface Bike {
- default void display() {
- System.out.println("Bike is running smooth");
- }
- }
- class Test implements Car, Bike {
- public void display() {
- Car.super.display();
- Bike.super.display();
- Car.run();
- System.out.println("Hello, I am Test class");
- }
- }
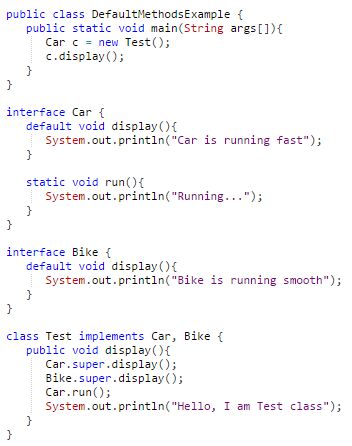
Output


Summary
Thus, we learned that Default methods has a capability to add for backward compatibility thus that old interfaces can use to leverage the lambda expression capability of Java 8 and also learns how to use it in Java.