sleep() Method In Java
sleep() method
The Thread class sleep() method is used to sleep a thread for the particular duration of time.
In other words, java.lang.Thread.sleep(long millis) method causes the presently executing thread to sleep for the specific number of milliseconds, subject to the precision and accurateness of the system timers and schedulers.
The Thread class provides two methods for sleeping a thread, which are,
Syntax
public static void sleep(long miliseconds)throws InterruptedException
public static void sleep(long miliseconds, int nanos)throws InterruptedException
Let’s see an example of sleep method, which is given below.
Code
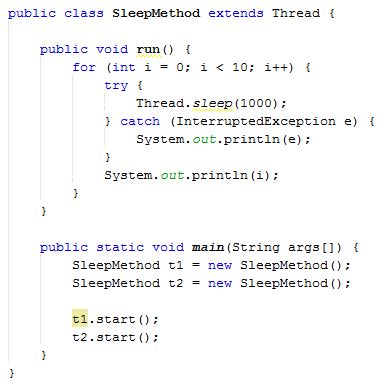
- public class SleepMethod extends Thread {
- public void run() {
- for (int i = 0; i < 10; i++) {
- try {
- Thread.sleep(1000);
- } catch (InterruptedException e) {
- System.out.println(e);
- }
- System.out.println(i);
- }
- }
- public static void main(String args[]) {
- SleepMethod t1 = new SleepMethod();
- SleepMethod t2 = new SleepMethod();
- t1.start();
- t2.start();
- }
- }
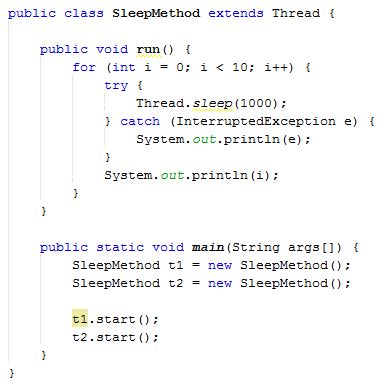
Output
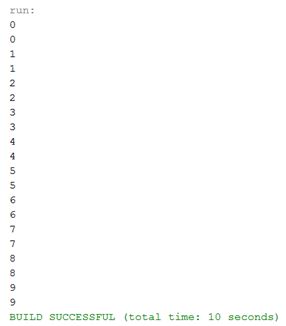
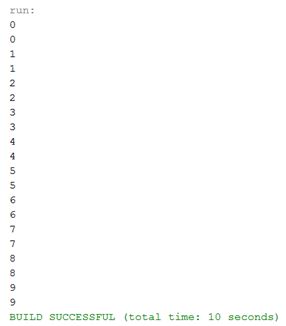
In the example, mentioned above, we know that at a time, only one thread is executed. If one thread sleeps for the specified time then the thread scheduler picks up another thread and so on.
Summary
Thus, we learnt that the Thread class sleep() method is used to sleep a thread for the particular amount of time and also learnt, how to use join() method in Java.