Multiple Catch In Java
Multiple catch blocks
If we want to do different tasks at the occurrence of the different exceptions, we need to use Java multi catch block.
A try block can have any number of catch blocks, i.e. written for catching the class Exception, which can catch all other exceptions.
Syntax
catch(Exception e){
//This catch block catches all the exceptions
}
- When multiple catch blocks are present in a program, the catch block, shown above, should be placed at the last as per the exception handling.
- When the try block is not throwing any exception, the catch block will be ignored, the program continues and if the try block throws an exception then, the appropriate catch block will catch it. All the statements in the catch block will be executed and then the program continues.
Let's see an example of multi-catch block.
Code
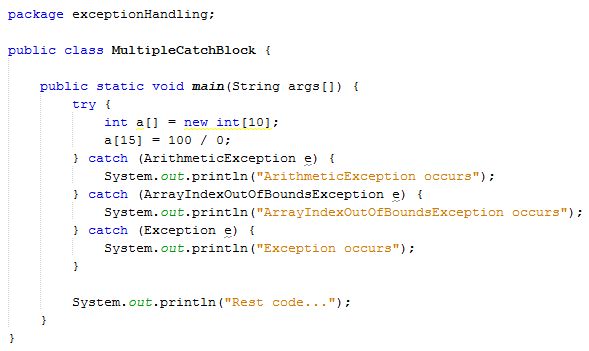
- package exceptionHandling;
- public class MultipleCatchBlock {
- public static void main(String args[]) {
- try {
- int a[] = new int[10];
- a[15] = 100 / 0;
- } catch (ArithmeticException e) {
- System.out.println("ArithmeticException occurs");
- } catch (ArrayIndexOutOfBoundsException e) {
- System.out.println("ArrayIndexOutOfBoundsException occurs");
- } catch (Exception e) {
- System.out.println("Exception occurs");
- }
- System.out.println("Rest code...");
- }
- }
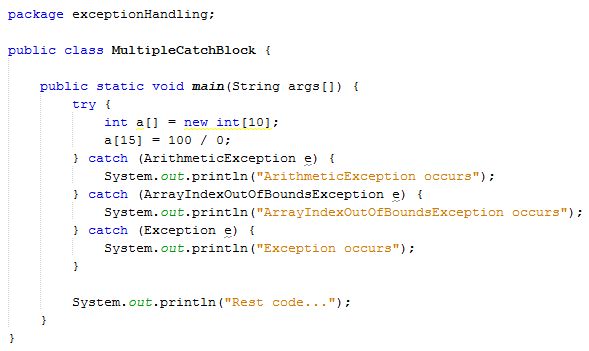
Output
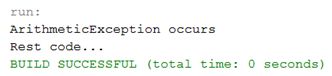
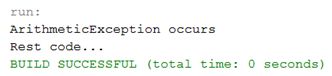
Let’s see another example, given below.
Code
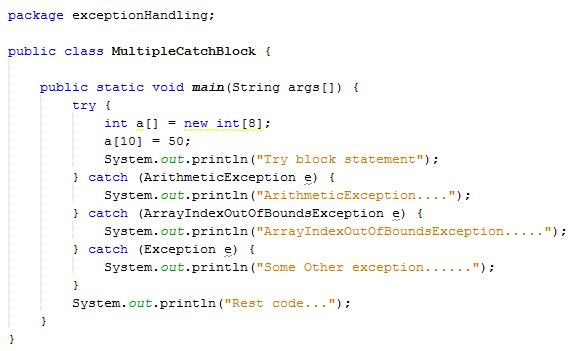
- package exceptionHandling;
- public class MultipleCatchBlock {
- public static void main(String args[]) {
- try {
- int a[] = new int[8];
- a[10] = 50;
- System.out.println("Try block statement");
- } catch (ArithmeticException e) {
- System.out.println("ArithmeticException....");
- } catch (ArrayIndexOutOfBoundsException e) {
- System.out.println("ArrayIndexOutOfBoundsException.....");
- } catch (Exception e) {
- System.out.println("Some Other exception......");
- }
- System.out.println("Rest code...");
- }
- }
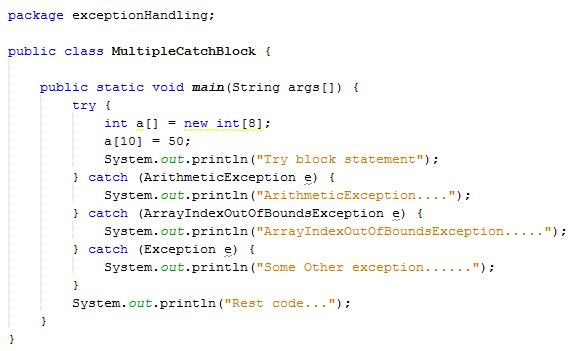
Output
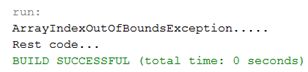
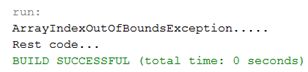
In the example, mentioned above, there are multiple catch blocks in this program and these catch blocks executes in sequence, when an exception occurs in try block.
Summary
Thus, we learnt that if we have to do different tasks at the occurrence of different exceptions, we need to use Java multi catch block in Java and also learnt its rules.