HashMap Class In Java
HashMap Class
In Java, HashMap class holds values, based on the key. It implements the map interface and extends AbstractMap class. It contains only unique elements, which may have one null key and multiple null values. It maintains no order.
Constructors of HashMap Class
HashMap( )
This constructor is used to construct a default HashMap.
HashMap(Map m)
This constructor is used to initialize the hash map, using the elements of the given map object m.
HashMap(int capacity)
This constructor is used to initialize the capacity of the hash map to the given integer value and capacity.
HashMap(int capacity, float fillRatio)
This constructor is used to initialize both the capacity and fill ratio of the hash map, using its arguments.
Methods of HashMap Class
void clear()
This method is used to remove all the mappings from the map.
Object clone()
This method is used to return a shallow copy of the HashMap object- the keys and values themselves are not cloned.
boolean containsKey(Object key)
This method is used to return true, if the map contains a mapping for the specified key or not.
boolean containsValue(Object value)
This method is used to return true, if the map maps one or more keys to the specified value.
Set entrySet()
This method is used to return a collection view of the mappings contained in the map.
Object get(Object key)
This method is used to return the value to which the specified key is mapped in the identity hash map or null, if the map contains no mapping for the key.
boolean isEmpty()
This method is used to return true, if the map contains no key-value mappings.
Set keySet()
This method is used to return a set view of the keys contained in the map.
Object put(Object key, Object value)
This method is used to associate the specified value with the specified key in the map.
putAll(Map m)
This method is used to copy all the mappings from the specified map to the map. These mappings will replace any mappings, which the map had for any of the keys, currently in the specified map.
Object remove(Object key)
This method is used to remove the mapping for the key from the map, if present.
int size()
This method is used to return the number of key-value mappings in the map.
Collection values()
This method is used to return a collection view of the values contained in the map.
Let’s see an example, given below.
Code
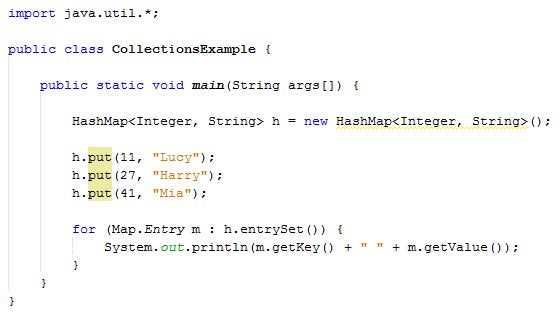
- import java.util.*;
- public class CollectionsExample {
- public static void main(String args[]) {
- HashMap < Integer, String > h = new HashMap < Integer, String > ();
- h.put(11, "Lucy");
- h.put(27, "Harry");
- h.put(41, "Mia");
- for (Map.Entry m: h.entrySet()) {
- System.out.println(m.getKey() + " " + m.getValue());
- }
- }
- }
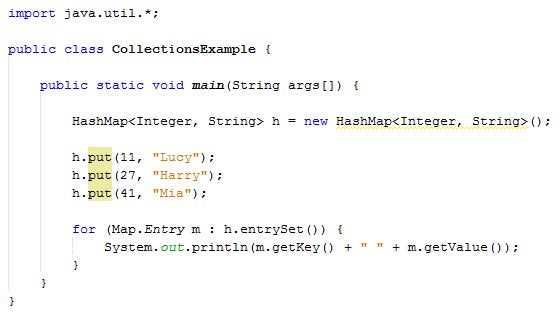
Output
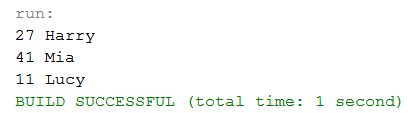
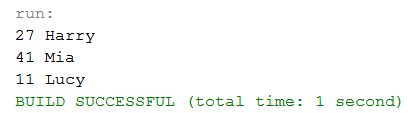
One important difference between HashSet and HashMap is HashSet contains only the values whereas HashMap contains the entry (key and value).
Summary
Thus, we learnt that Java HashMap class holds the values, based on the key. It implements the map interface and extends AbstractMap class. It holds only the unique elements and also learnt how to create it in Java.