Difference Between ArrayList And LinkedList In Java
In Java collections, both ArrayList and LinkedList classes implements List interface and maintains an insertion order and both classes are non-synchronized.
Difference between ArrayList and LinkedList classes
There are many differences between ArrayList and LinkedList classes, which are,
ArrayList | LinkedList |
ArrayList uses dynamic array to store the elements internally. | LinkedList uses doubly linked list to store the elements internally. |
Its manipulation is slow because it internally uses an array. If any element is removed from the array, all the bits are shifted in the memory. | Its manipulation is faster than ArrayList because it uses doubly linked list. Thus no bit shifting is required in memory. |
ArrayList class can perform as a list only because it implements List only. | LinkedList class can perform as a list and queue both because it implements List and Deque interfaces. |
ArrayList is good for storing and accessing data. | LinkedList is good for manipulating data. |
ArrayList cannot be iterated in reverse direction. | LinkedList can be iterated in reverse direction. |
Let’s see an example of ArrayList and LinkedList.
Code
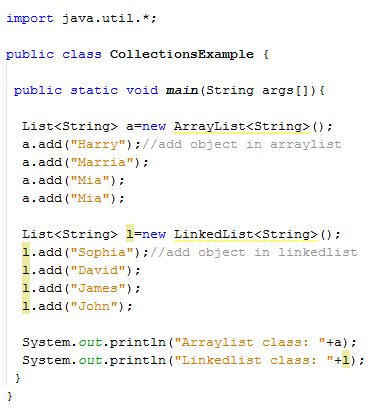
- import java.util.*;
- public class CollectionsExample {
- public static void main(String args[]) {
- List < String > a = new ArrayList < String > ();
- a.add("Harry"); //add object in arraylist
- a.add("Marria");
- a.add("Mia");
- a.add("Mia");
- List < String > l = new LinkedList < String > ();
- l.add("Sophia"); //add object in linkedlist
- l.add("David");
- l.add("James");
- l.add("John");
- System.out.println("Arraylist class: " + a);
- System.out.println("Linkedlist class: " + l);
- }
- }
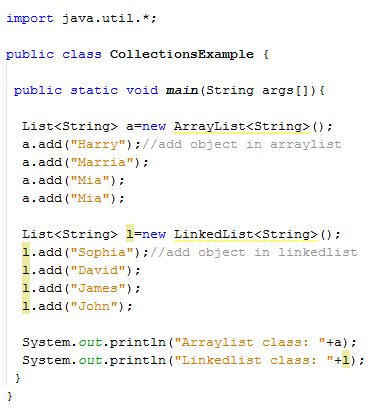
Output
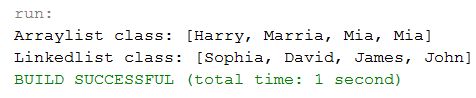
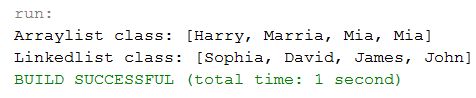
Summary
Thus, we learnt that both ArrayList and LinkedList classes implements list interface, maintains an insertion order as both classes are non-synchronized and also learnt their differences in Java.