Introduction To Date And Time In Java
The java.util, java.sql and java.text packages contain classes for representing date and time in Java.
There are many classes in Java, which deals with the date.
- java.util.Date class
- java.sql.Date class
- java.text.DateFormat class
- java.text.SimpleDateFormat class
java.util.Date
In Java, java.util.Date class represents the date and time. It provides many constructors and methods to deal with the date and time.
The java.util.Date class implements Serializable, Cloneable and Comparable<Date> interface, extends by java.sql.Date interface, java.sql.Time interface and java.sql.Timestamp interface.
After Calendar class, many constructors and methods of java.util.Date class has been deprecated.
Constructors of java.util.Date
Date( )
This constructor is used to initialize the object with the current date and time.
Date(long millisec)
This constructor is used to accept an argument, which equals the number of milliseconds, which have elapsed since midnight, January 10, 1990.
Methods of java.util.Date
boolean after(Date date)
This method is used to return true, if the calling date object contains a date, which is later than the one specified by the date else it returns false.
boolean before(Date date)
This method is used to return true, if the calling date object contains a date, which is earlier than the one specified by the date, else it returns false.
Object clone( )
This method is used to duplicate the calling date object.
int compareTo(Date date)
This method is used to compare the value of the calling object with the date. It returns 0, if the values are equal, returns a negative value, if the invoking object is earlier than the date and returns a positive value, if the invoking objects is later than the date.
int compareTo(Object obj)
This method is used to operate identically to compareTo(Date), if the object is of class date, else it throws a ClassCastException.
boolean equals(Object date)
This method is used to return true, if the calling date object contains the same time and the date as one particular date, else it returns false.
long getTime( )
This method is used to return the number of milliseconds, which have elapsed since January 13, 1960.
int hashCode( )
This method is used to return a hash code for the calling object.
void setTime(long time)
This method is used to set the time and date as particular by the time, which represents an elapsed time in milliseconds from the midnight, January 16, 1950.
String toString( )
This method is used to convert the calling date object into a string and returns the result.
static Date from(Instant instant)
This method is used to return an instance of the date object from the Instant date.
Instant toInstant()
This method is used to convert the current date into an Instant object.
Get Current Date
There are many ways to print the current date.
Let’s see an example1, given below.
Code
- public class DateExample {
- public static void main(String args[]) {
- java.util.Date d = new java.util.Date();
- System.out.println("Current Date:" + d);
- }
- }
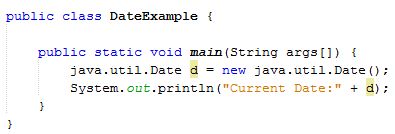
Output


Let’s see an example2, given below.
Code
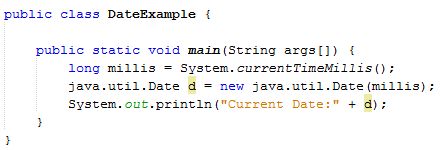
- public class DateExample {
- public static void main(String args[]) {
- long millis = System.currentTimeMillis();
- java.util.Date d = new java.util.Date(millis);
- System.out.println("Current Date:" + d);
- }
- }
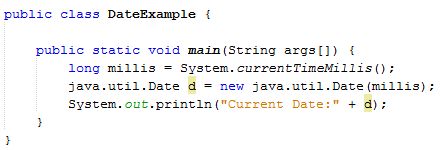
Output


Get Current Date with java.util.Calendar class
Calendar class can also used to get the object of date class. The getTime() method of calendar class returns the object of java.util.Date. The Calendar.getInstance() method returns the object of calendar class.
Let’s see an example, given below.
Code
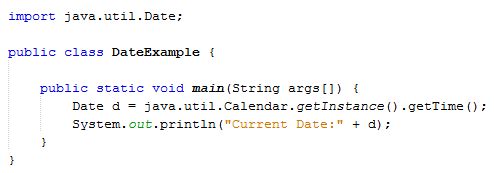
- import java.util.Date;
- public class DateExample {
- public static void main(String args[]) {
- Date d = java.util.Calendar.getInstance().getTime();
- System.out.println("Current Date:" + d);
- }
- }
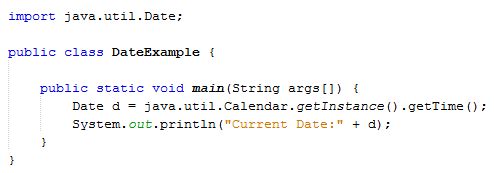
Output


java.sql.Date
In Java, java.sql.Date class represents only date. It extends java.util.Date class. It represents the date that can be stored in the database that’s why java.sql.Date object is generally used in JDBC.
But some constructors and methods of java.sql.Date class has been deprecated.
Constructor of java.sql.Date
Date(long milliseconds)
This constructor is used to create a SQL date object for the given milliseconds, since January 19, 1990, 00:00:00 GMT.
Methods of java.sql.Date
void setTime(long time)
This method is used to change the current SQL date to given time.
Instant toInstant()
This method is used to convert current SQL date into an Instant object.
LocalDate toLocalDate()
This method is used to convert current SQL date into LocalDate object.
String toString()
This method is used to convert SQL date object to a string.
static Date valueOf(LocalDate date)
This method is used to returns SQL date object for the given LocalDate.
static Date valueOf(String date)
This method is used to return SQL date object for the given String.
Let's see an example: get current Date, given below.
Code
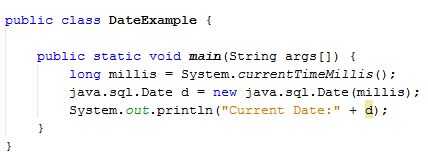
- public class DateExample {
- public static void main(String args[]) {
- long millis = System.currentTimeMillis();
- java.sql.Date d = new java.sql.Date(millis);
- System.out.println("Current Date:" + d);
- }
- }
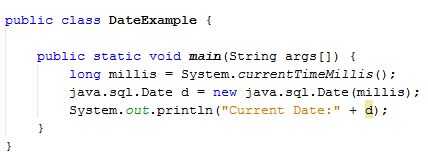
Output
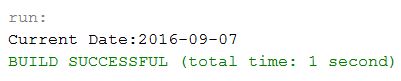
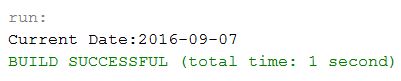
String to java.sql.Date
Let's see an example of convert string into java.sql.Date, given below.
Code
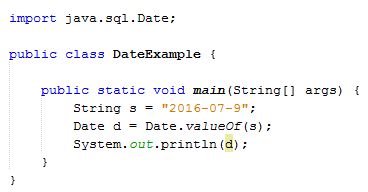
- import java.sql.Date;
- public class DateExample {
- public static void main(String[] args) {
- String s = "2016-07-9";
- Date d = Date.valueOf(s);
- System.out.println(d);
- }
- }
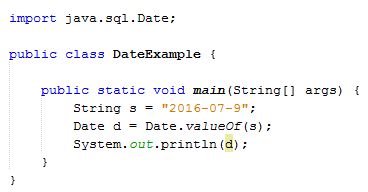
Output


Summary
Thus, we learnt, java.util, java.sql and java.text packages contains the classes to represent the date and time and we also learnt, how to create it in Java.