Introduction Of Exception Handling In Java
What is Exception in Java?
In Java, exception is an abnormal condition, which means that it is an event, which disrupts the normal flow of the program. It is an object, which is thrown at the runtime.
In other words, we can say that exception can be anything, which disrupts the normal flow of the program. Once an exception occurs, program stops and doesn’t continue further. In such cases, we get a system generated error message.
An exception occurs at a runtime called as runtime exceptions and at compile-time called as compile-time exceptions.
There are various reasons for an exception. For example, opening a non-existing file, network connection problem and class file missing, which was supposed to be loaded etc. These all following situations can cause an exception.
Difference between error and exception in Java
An error shows the serious problems and abnormal conditions, which most Applications should not try to handle. Error describes the problems, which are not predictable to be caught under the normal circumstances by our program. For example, memory error, hardware error, JVM errors etc.
The exception refers to the conditions within the code. A programmer can handle such conditions and take the required corrective actions. For example DivideByZero exception, NullPointerException, ArithmeticException, ArrayIndexOutOfBoundsException etc.
Advantages of Exception Handling
- Exception handling is mainly used to allow us to control the normal flow of the program, using exception handling in the program.
- Exception handling throws an exception, when a calling method encounters an error providing that the calling method takes care of that error.
- Exception handling also gives us the scope of organizing and differentiating between the different errors types, using a separate block of codes. This is done with the help of try-catch blocks.
Why to handle exception?
If an exception is raised and that has not been handled by the developer, the program execution can be ended and the system prints a non user friendly error message.
What is Exception handling in Java
In Java, exception handling is a very powerful mechanism to handle the runtime errors such as ClassNotFound, IO, SQL, Remote etc. Hence, the normal flow of the Application can be maintained.
Exception handling is mainly used to continue the normal flow of the Application. Exception normally disrupts the normal flow of the Application due to which we use exception handling. For example,
Statement 1;
Statement 2;
Statement 3; //exception occurs
Statement 4;
Suppose, there are 4 statements in our program and there occurs an exception at the statement 3, rest of the code will not be executed i.e. statement 4 will not run. If we do an exception handling, rest of the statement will be executed. Due to this, we use exception handling in Java.
Hierarchy of Exception classes in Java
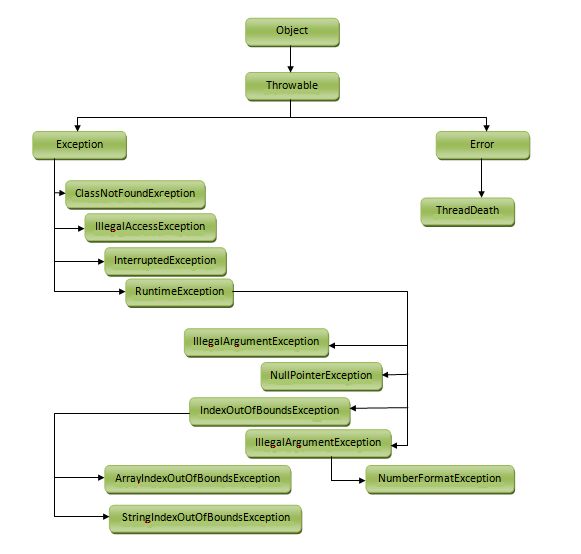
Types of Exceptions in Java
In Java, two types of exceptions- checked and unchecked, where an error is considered as unchecked exception. Sun microsystem declares that there are three types of exceptions, which are,
- Checked Exception
- Unchecked Exception
- Error
Difference between checked and unchecked exceptions in Java are,
- Checked Exception
The classes that extend Throwable class except RuntimeException and error are called checked exceptions. For example, IOException, SQLException etc. Checked exceptions can be checked at the compile-time. - Unchecked Exception
The classes that extend RuntimeException are called as unchecked exceptions. For example, ArithmeticException, NullPointerException etc. Unchecked exceptions cannot be checked at the compile-time. Rather, they are checked at the runtime only. - Error
Error is irrecoverable. For example, OutOfMemoryError, VirtualMachineError, AssertionError, JVM errors etc.
Many common scenarios where exceptions may occur in Java programs are,
- ArithmeticException occurs
ArithmeticException occurs if you divide any value with zero.
For example.- int n=36/0;//ArithmeticException
- NullPointerException occurs
NullPointerException occurs if we have null value in any variable and perform any operation with the help of the variable.
For example.- String n=null;
- System.out.println(n.length());//NullPointerException
- NumberFormatException occurs
NumberFormatException occurs if the wrong formatting of any value is done. Suppose we have a string variable that has characters, convert this variable into the digit will occur NumberFormatException.
For example.- String n="Bob";
- int i=Integer.parseInt(n);//NumberFormatException
- ArrayIndexOutOfBoundsException occurs
ArrayIndexOutOfBoundsException occurs if we insert any value in the incorrect index, it would result ArrayIndexOutOfBoundsException.
For example.- int a[]=new int[10];
- a[12]=100; //ArrayIndexOutOfBoundsException
Exception handling keywords in Java
There are five keywords used in exception handling, which are,
- try
- catch
- finally
- throw
- throws
Summary
Thus, we learnt that exception handling is a very powerful mechanism to handle the runtime errors in Java and also learnt its types and advantages.