Method Overloading In Java
Method Overloading
Method overloading in Java says when we use two or more methods as a same name within a same class but their parameter statements should be different. This case is said to be a method overloading in Java.
Java has a capability to overload the same method in a class. Method overloading is very important and has useful features in Java. With the help of it, we can enhance the readability of the code.
Suppose, we have two methods and both name are different, but there are any number of arguments like: x (int) and y (int, int). Hence, it may be to create a problem to understand its functionality of the code because the method name is different. Due to this, we use method overloading.
In Java, we can overload the method by two ways, which are,
- Change the number of arguments
- Change the data type
Example of method overriding with change number of arguments is given below.
Code
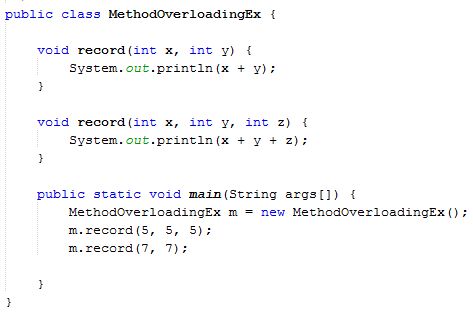
- public class MethodOverloadingEx {
- void record(int x, int y) {
- System.out.println(x + y);
- }
- void record(int x, int y, int z) {
- System.out.println(x + y + z);
- }
- public static void main(String args[]) {
- MethodOverloadingEx m = new MethodOverloadingEx();
- m.record(5, 5, 5);
- m.record(7, 7);
- }
- }
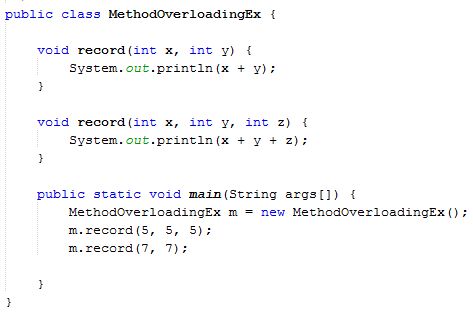
Output
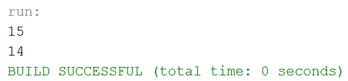
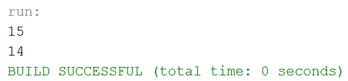
Example of method Overriding with change in the data type is given below.
Code
- public class MethodOverloadingEx {
- void record(int x, int y) {
- System.out.println(x + y);
- }
- void record(long x, long y, long z) {
- System.out.println(x + y + z);
- }
- public static void main(String args[]) {
- MethodOverloadingEx m = new MethodOverloadingEx();
- m.record(9, 6, 3);
- m.record(2, 5);
- }
- }
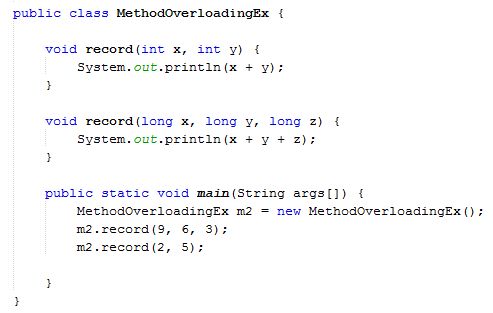
Output
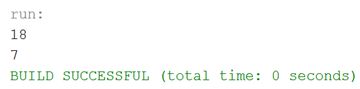
Java is not capable to overload the method by changing the return type because it may be create confusion (ambiguity).
Let’s see an example, when we change the return type of method of a class, which may be to create a problem.
Code
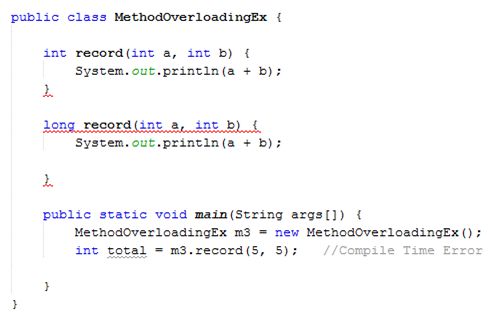
- public class MethodOverloadingEx {
- int record(int a, int b) {
- System.out.println(a + b);
- }
- long record(int a, int b) {
- System.out.println(a + b);
- }
- public static void main(String args[]) {
- MethodOverloadingEx m3 = new MethodOverloadingEx();
- int total = m3.record(5, 5); //Compile Time Error
- }
- }
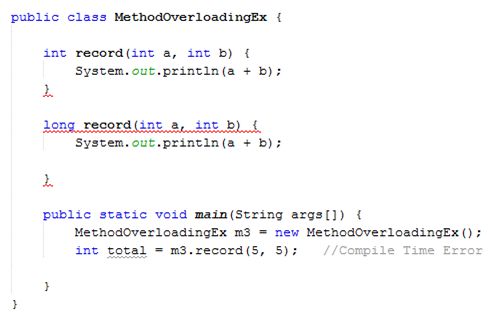
One thing is important to know that in Java, we can overload the main method.
public static void main(String args[]){
We can overload this method with the same name.
For example
Code
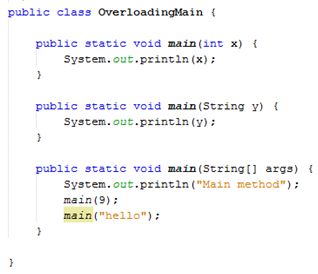
- public class OverloadingMain {
- public static void main(int x) {
- System.out.println(x);
- }
- public static void main(String y) {
- System.out.println(y);
- }
- public static void main(String[] args) {
- System.out.println("Main method");
- main(9);
- main("hello");
- }
- }
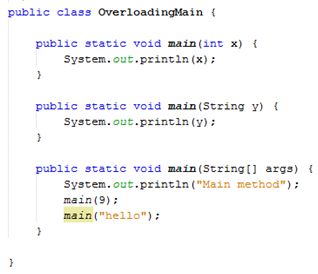
Output
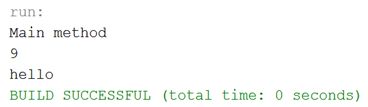
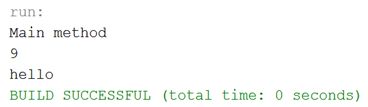
Summary
Thus, we learnt when we use two or more methods with a same name within a same class is called as a method overloading in Java and also learnt how we can overload the method.