Synchronized Block In Java
Synchronized Block
In Java, Synchronized block can used to achieve synchronization on any particular resource of the method.
Assume that we have 100 lines of code in our method but we want to synchronize it only 10 lines then we can use synchronized block. If we put all the codes of the method in the synchronized block, it will work in similar fashion, as the synchronized method.
Important points for Synchronized block
- This block is mainly used to lock an object for any shared resource.
- Its scope is smaller than the method.
Syntax
synchronized (object reference expression) {
//code
}
Let's see an example of the synchronized block, given below.
Code
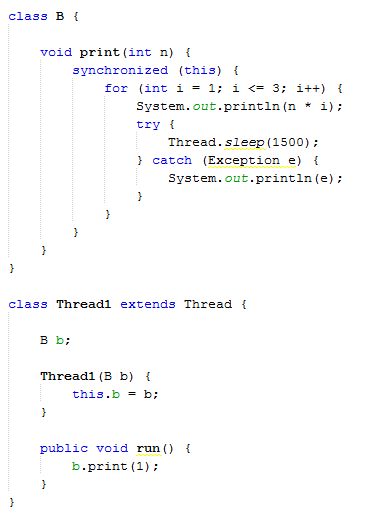
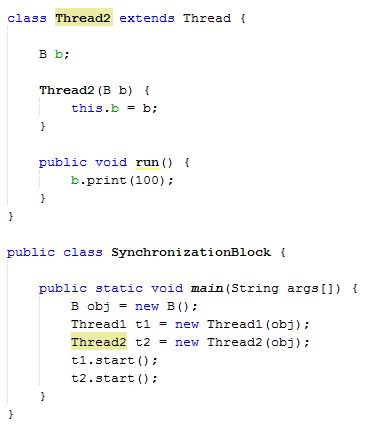
- class B {
- void print(int n) {
- synchronized(this) {
- for (int i = 1; i <= 3; i++) {
- System.out.println(n * i);
- try {
- Thread.sleep(1500);
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
- }
- }
- class Thread1 extends Thread {
- B b;
- Thread1(B b) {
- this.b = b;
- }
- public void run() {
- b.print(1);
- }
- }
- class Thread2 extends Thread {
- B b;
- Thread2(B b) {
- this.b = b;
- }
- public void run() {
- b.print(100);
- }
- }
- public class SynchronizationBlock {
- public static void main(String args[]) {
- B obj = new B();
- Thread1 t1 = new Thread1(obj);
- Thread2 t2 = new Thread2(obj);
- t1.start();
- t2.start();
- }
- }
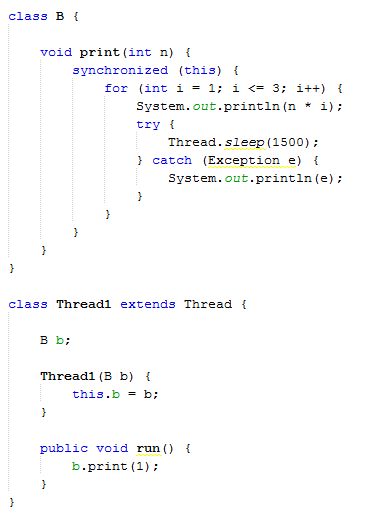
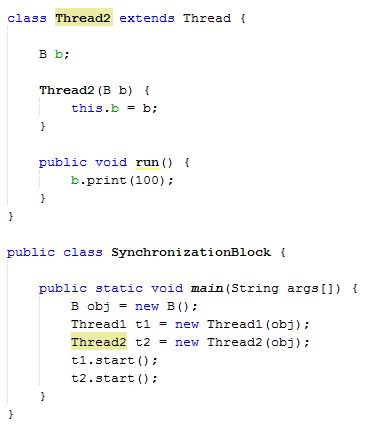
Output
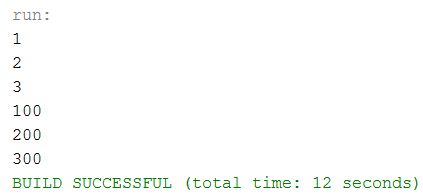
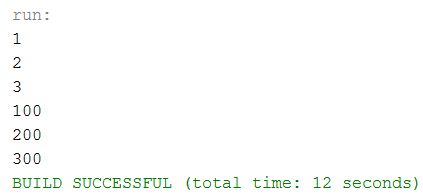
Let’s see an example of synchronized block with annonymous class.
Code
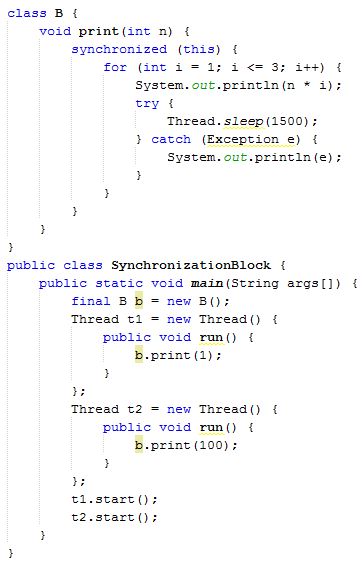
- class B {
- void print(int n) {
- synchronized(this) {
- for (int i = 1; i <= 3; i++) {
- System.out.println(n * i);
- try {
- Thread.sleep(1500);
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
- }
- }
- public class SynchronizationBlock {
- public static void main(String args[]) {
- final B b = new B();
- Thread t1 = new Thread() {
- public void run() {
- b.print(1);
- }
- };
- Thread t2 = new Thread() {
- public void run() {
- b.print(100);
- }
- };
- t1.start();
- t2.start();
- }
- }
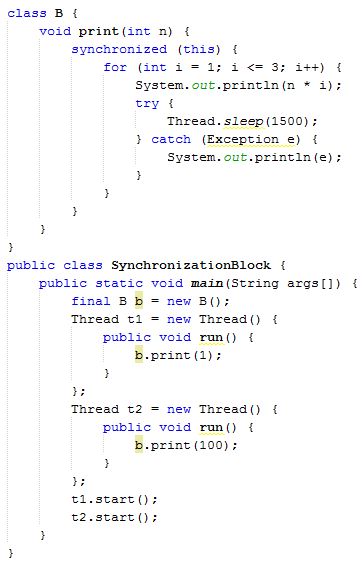
Output
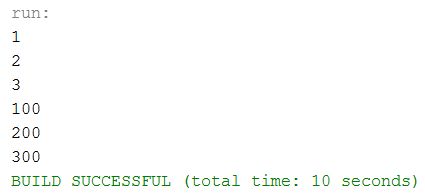
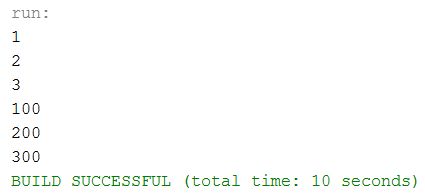
Summary
Thus, we learnt Java synchronized block can used to achieve synchronization on any particular resource of the method and also learnt how we can create it in Java.