Method Overriding In Java
Method Overriding
In Java, method overriding means to override the method of an existing method. With the use of method overriding, we can create two methods with same name.
If child class provides the specific implementation of the method, which is already provided by its parent class. It is called as a method overriding.
Advantage of Method Overriding
- It is used to achieve runtime polymorphism.
- It is used to provide specific implementation of a method through child class, which is already provided by its super class.
Let’s see an example, given below.
Code
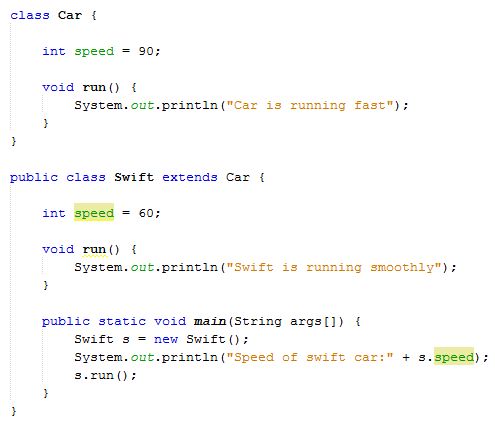
- class Car {
- int speed = 90;
- void run() {
- System.out.println("Car is running fast");
- }
- }
- public class Swift extends Car {
- int speed = 60;
- void run() {
- System.out.println("Swift is running smoothly");
- }
- public static void main(String args[]) {
- Swift s = new Swift();
- System.out.println("Speed of swift car:" + s.speed);
- s.run();
- }
- }
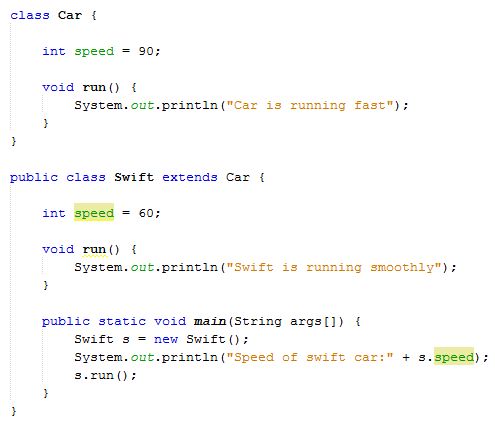
Output
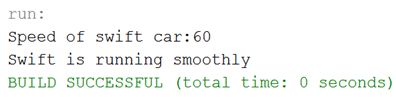
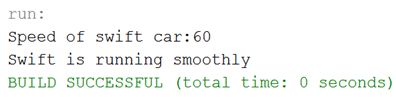
In the example, mentioned above, we defined the run method in the subclass, which is already defined in its parent class also but subclass has some specific implementation. There is IS-A relationship (inheritance) between the classes. Both classes have same method name and parameter. Hence, there is a method overriding.
Rules of Method Overriding in Java
- Method argument list must be same name as the parent class.
- Must have IS-A relationship.
- Return type must be same.
- Access level cannot be more restrictive than the overridden method’s access level and super class method, which is not declared private.
- Final method cannot be overridden.
- Static method cannot be overridden but can be re-declared.
- Constructor cannot be overridden.
In Java, we cannot override static method because static method is bound with class and instance method, which is bound with the object. Their memories are also different. Static belongs to class area and instance belongs to heap area.
We cannot override Java main method also because main method is static method.
Summary
Thus, we learnt that method overriding means to override the method of an existing method in Java and also learnt how we can override the method.