StringBuilder Class In Java
StringBuilder Class
StringBuilder class is also used to create mutable string in Java. StringBuilder class is available since JDK 1.5.
StringBuilder class is similar as StringBuffer class except that it is non-synchronized, which means that it is not thread safe due to which all its methods are also non-synchronised in Java.
In other words, we can say that StringBuilder class is mutable sequence of characters, which provides an API compatible with StringBuffer, but it is not thread safe.
Three important constructors of StringBuilder class are,
- StringBuilder()- This constructor is creates a blank string builder with the initial capacity of 16.
- StringBuilder(String str)- This constructor creates a string builder with the particular string.
- StringBuilder(int length)- This constructor creates an blank string builder with the specified capacity as length.
There are many important methods of StringBuilder class in Java, which are,
public StringBuilder append(String s)
This method is used to append the particular string with this string and the append() method is overloaded like append(int), append(double) etc.
This method is used to append the particular string with this string and the append() method is overloaded like append(int), append(double) etc.
public StringBuilder insert(int offset, String s)
This method is used to insert the particular string with this string at the specified position and the insert() method is overloaded like insert(int, int), insert(int, float) etc.
This method is used to insert the particular string with this string at the specified position and the insert() method is overloaded like insert(int, int), insert(int, float) etc.
public StringBuilder replace(int startIndex, int endIndex, String str)
This method is used to replace the string from the particular startIndex and endIndex.
This method is used to replace the string from the particular startIndex and endIndex.
public StringBuilder delete(int startIndex, int endIndex)
This method is used to delete the string from the particular startIndex and endIndex.
This method is used to delete the string from the particular startIndex and endIndex.
public StringBuilder reverse()
This method is used to reverse the string.
This method is used to reverse the string.
public int capacity()
This method is used to return the current capacity of the string.
This method is used to return the current capacity of the string.
public void ensureCapacity(int minimumCapacity)
This method is used to ensure the capacity, at least equal to the given minimum.
This method is used to ensure the capacity, at least equal to the given minimum.
public char charAt(int index)
This method is used to return the character at the specified position.
This method is used to return the character at the specified position.
public String substring(int beginIndex)
This method is used to return the substring from the particular beginIndex.
This method is used to return the substring from the particular beginIndex.
public String substring(int beginIndex, int endIndex)
This method is used to return the substring from the particular beginIndex and endIndex.
This method is used to return the substring from the particular beginIndex and endIndex.
There are many methods of StringBuilder class which methods are also non-synchronised in Java.
Let's see the examples of methods of StringBuilder class.
StringBuilder append() method
The append() method is used to concatenates the given argument with the string.
Let’s see an example.
Code
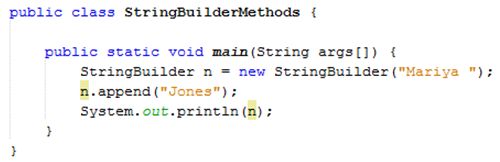
- public class StringBuilderMethods {
- public static void main(String args[]) {
- StringBuilder n = new StringBuilder("Mariya ");
- n.append("Jones");
- System.out.println(n);
- }
- }
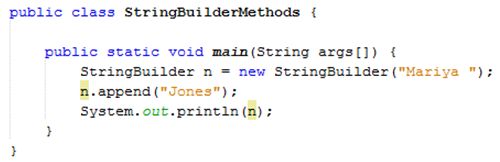
Output


In the above example, Original string is changed and gives the output.
StringBuilder insert() method
The insert() method is used to insert the given string with the string at the specified position.
Let’s see an example.
Code
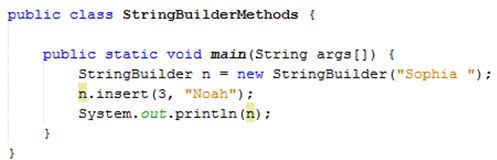
- public class StringBuilderMethods {
- public static void main(String args[]) {
- StringBuilder n = new StringBuilder("Sophia ");
- n.insert(3, "Noah");
- System.out.println(n);
- }
- }
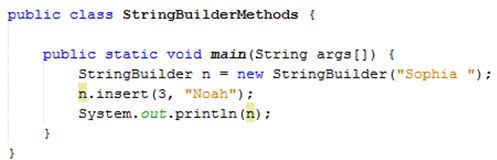
Output


In the above example, Original string is changed and gives the output.
StringBuilder replace() method
The replace() method is used to replace the given string from the particular beginIndex and endIndex.
Let’s see an example.
Code
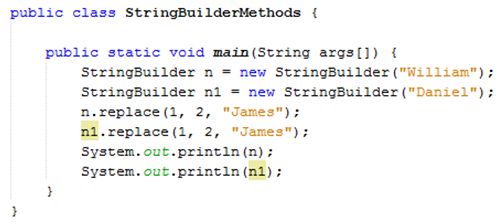
- public class StringBuilderMethods {
- public static void main(String args[]) {
- StringBuilder n = new StringBuilder("William");
- StringBuilder n1 = new StringBuilder("Daniel");
- n.replace(1, 2, "James");
- n1.replace(1, 2, "James");
- System.out.println(n);
- System.out.println(n1);
- }
- }
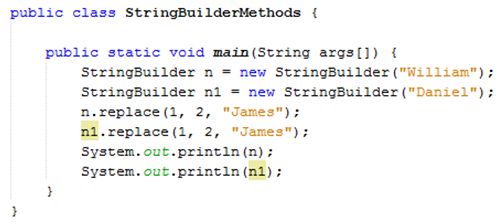
Output
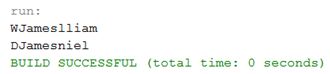
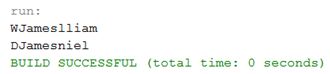
StringBuilder delete() method
The delete() method is used to delete the string from the particular beginIndex to endIndex.
Let’s see an example.
Code
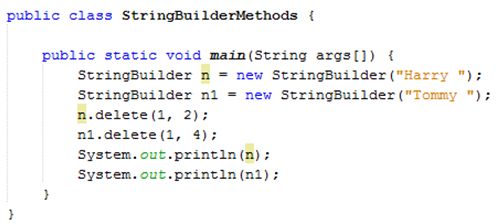
- public class StringBuilderMethods {
- public static void main(String args[]) {
- StringBuilder n = new StringBuilder("Harry ");
- StringBuilder n1 = new StringBuilder("Tommy ");
- n.delete(1, 2);
- n1.delete(1, 4);
- System.out.println(n);
- System.out.println(n1);
- }
- }
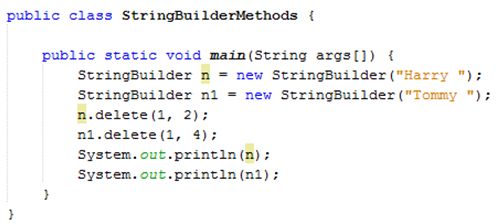
Output
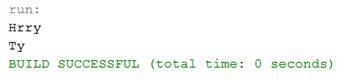
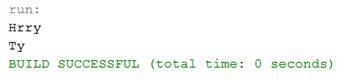
StringBuilder reverse() method
The reverse() method is used to reverse the current string.
Let’s see an example.
Code
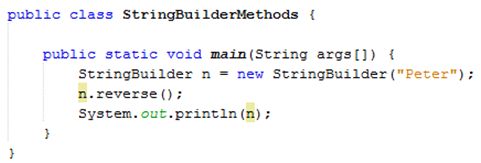
- public class StringBuilderMethods {
- public static void main(String args[]) {
- StringBuilder n = new StringBuilder("Peter");
- n.reverse();
- System.out.println(n);
- }
- }
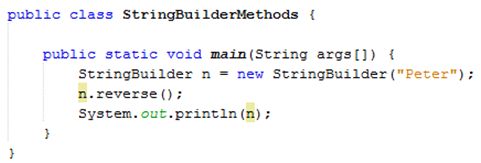
Output


StringBuilder capacity() method
The capacity() method is used to returns the current capacity of the Builder in Java. The default capacity of the Builder is 16 and If the number of character increases from its current capacity then, it increases the capacity by (old capacity*2) +2.
Let’s see an example.
Code
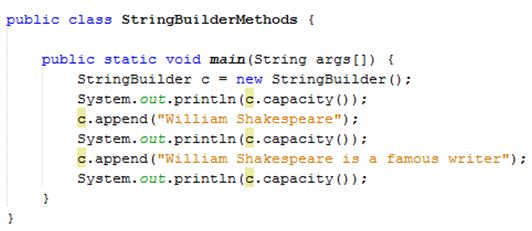
- public class StringBuilderMethods {
- public static void main(String args[]) {
- StringBuilder c = new StringBuilder();
- System.out.println(c.capacity());
- c.append("William Shakespeare");
- System.out.println(c.capacity());
- c.append("William Shakespeare is a famous writer");
- System.out.println(c.capacity());
- }
- }
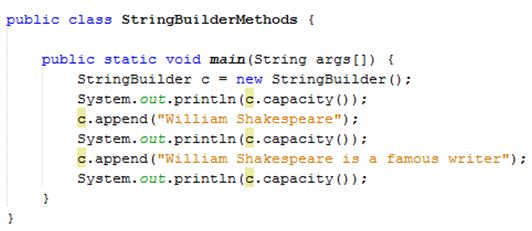
Output
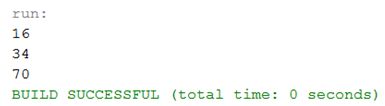
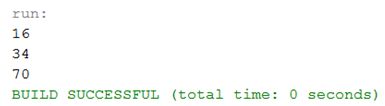
StringBuilder ensureCapacity() method
The ensureCapacity() method is used to ensures that the given capacity is the minimum to the current capacity and If it is greater than the current capacity then, it increases the capacity by (old capacity*2)+2.
Let’s see an example.
Code
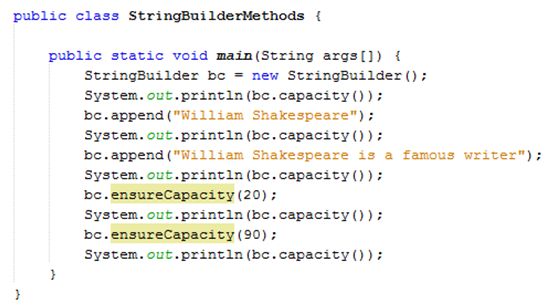
- public class StringBuilderMethods {
- public static void main(String args[]) {
- StringBuilder bc = new StringBuilder();
- System.out.println(bc.capacity());
- bc.append("William Shakespeare");
- System.out.println(bc.capacity());
- bc.append("William Shakespeare is a famous writer");
- System.out.println(bc.capacity());
- bc.ensureCapacity(20);
- System.out.println(bc.capacity());
- bc.ensureCapacity(90);
- System.out.println(bc.capacity());
- }
- }
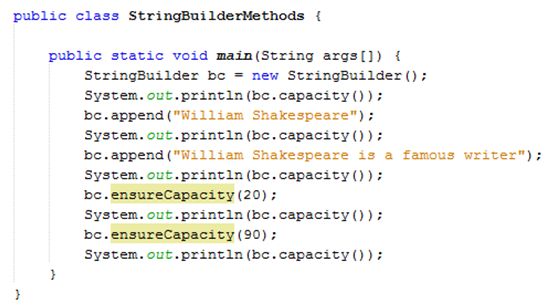
Output
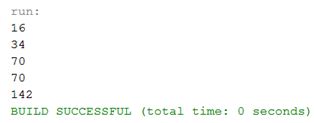
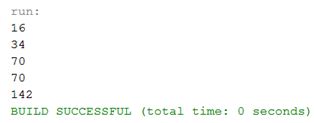
Summary
Thus, we learned that StringBuilder class is same as StringBuffer class except that it is non-synchronized in Java and also learn its important methods with example.