ResultSet Interface In Java
ResultSet Interface
In JDBC, ResultSet object maintains a cursor pointing to a row of a table. First, the cursor points to before the first row. ResultSet object can be moved forward only and it is not updatable by default.
We can make the ResultSet object to move the forward and backward direction by passing either TYPE_SCROLL_INSENSITIVE or TYPE_SCROLL_SENSITIVE in createStatement(int,int) method and we can make the ResultSet object as updatable, given below.
Statement stmt = con.createStatement(ResultSet.TYPE_SCROLL_INSENSITIVE,ResultSet.CONCUR_UPDATABLE);
Methods of ResultSet interface
public boolean next()
This method is used to move the cursor to the one row next from the current position.
public boolean previous()
This method is used to move the cursor to the one row before from the current position.
public boolean first()
This method is used to move the cursor to the first row in the result set object.
public boolean last()
This method is used to move the cursor to the last row in the result set object.
public boolean absolute(int row)
This method is used to move the cursor to the particular row number in the ResultSet object.
public boolean relative(int row)
This method is used to move the cursor to the relative row number in the ResultSet object, as it might be positive or negative.
public int getInt(int columnIndex)
This method is used to return the data of the particular column index of the current row as int.
public int getInt(String columnName)
This method is used to return the data of the particular column name of the current row as int.
public String getString(int columnIndex)
This method is used to return the data of the particular column index of the current row as String.
public String getString(String columnName)
This method is used to return the data of the particular column name of the current row as String.
Let’s see an example of Scrollable ResultSet, given below.
Code
- import java.sql.*;
- public class StudentDatabase1 {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- Statement st = conn.createStatement(ResultSet.TYPE_SCROLL_SENSITIVE, ResultSet.CONCUR_UPDATABLE);
- ResultSet rs = st.executeQuery("select * from STUDENT.STUDENTDB");
- rs.absolute(3);
- System.out.println(rs.getString(1) + " " + rs.getString(2) + " " + rs.getString(3) + " " + rs.getString(4));
- conn.close();
- }
- }
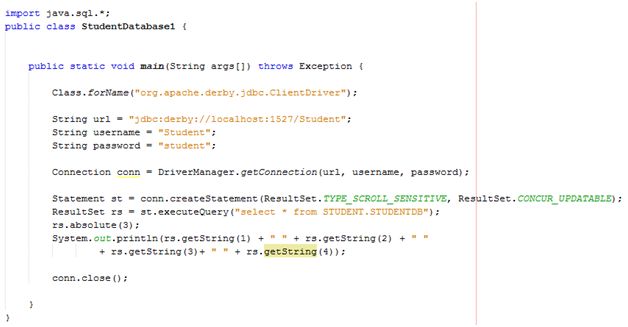
Output


In the example, mentioned above, ResultSet interface to retrieve the data of 3rd row.
Summary
Thus, we learnt, JDBC ResultSet object maintains a cursor pointing to a row of a table. First, cursor points to, before the first row. ResultSet object can be moved forward only and it is not updatable by default and also learnt its important methods in Java.