LinkedList Class In Java
LinkedList class
In Java collections, LinkedList class uses doubly linked list to store the elements. It extends the AbstractList class and implements the list and deque the interfaces. It can contain duplicate elements and maintains an insertion order. This class is non-synchronized and its manipulation is fast because no shifting requires to be occurred. LinkedList class can be used as a list, stack or queue.
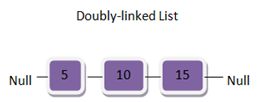
Constructors of LinkedList class
LinkedList( )
This constructor is used to build an empty linked list.
LnkedList(Collection c)
This constructor is used to build a linked list, which is initialized with the elements of the collection c.
Methods of LinkedList class
void add(int index, Object element)
This method is used to insert the specified element at the specified position index in the list. It throws IndexOutOfBoundsException, if the specified index is out of range.
boolean add(Object o)
This method is used to append the particular element to the end of the list.
boolean addAll(Collection c)
This method is used to append all the elements in the specified collection to the end of the list and in the order in which they are returned by the particular collection's iterator.
boolean addAll(int index, Collection c)
This method is used to insert all the elements in the specified collection into the list, starting at the specified position.
void addFirst(Object o)
This method is used to insert the given element at the beginning of the list.
void addLast(Object o)
This method is used to append the given element to the end of the list.
void clear()
This method is used to remove all the elements from the list.
Object clone()
This method is used to return a shallow copy of the LinkedList.
boolean contains(Object o)
This method is used to return true, if this list contains the specified element or not. More formally, it returns true, if the list contains at least one element.
Object get(int index)
This method is used to return the element at the specified position in the list. It throws IndexOutOfBoundsException, if the particular index is out of range.
Object getFirst()
This method is used to return the first element in the list and throws NoSuchElementException, if the list is empty.
Object getLast()
This method is used to return the last element in the list and throws NoSuchElementException, if the list is empty.
int indexOf(Object o)
This method is used to return the index in the list of the first occurrence of the specified element or-1, if the list does not contain the element.
int lastIndexOf(Object o)
This method is used to return the index in the list of the last occurrence of the specified element or -1, if the list does not contain the element.
ListIterator listIterator(int index)
This method is used to return a list-iterator of the elements in the list (in proper sequence) and starting at the specified position in the list. Throws IndexOutOfBoundsException, if the specified index is out of range.
Object remove(int index)
This method is used to remove the element at the specified position in the list. It throws NoSuchElementException, if the list is empty.
boolean remove(Object o)
This method is used to remove the first occurrence of the specified element in the list. It throws NoSuchElementException, if the list is empty. It throws IndexOutOfBoundsException, if the specified index is out of range.
Object removeFirst()
This method is used to remove and return the first element from the list. It throws NoSuchElementException, if the list is empty.
Object removeLast()
This method is used to remove and return the last element from the list. It throws NoSuchElementException, if the list is empty.
Object set(int index, Object element)
This method is used to replace the element at the particular position in the list with the specified element. It throws IndexOutOfBoundsException, if the specified index is out of range.
int size()
This method is used to return the number of elements in the list.
Object[] toArray()
This method is used to return an array, which contains all the elements in the list in the correct order and throws NullPointerException, if the specified array is null.
Object[] toArray(Object[] a)
This method is used to return an array, which contains all the elements in the list in the correct order and the runtime type of the returned array is that of the particular array.
Let’s see an example, given below.
Code
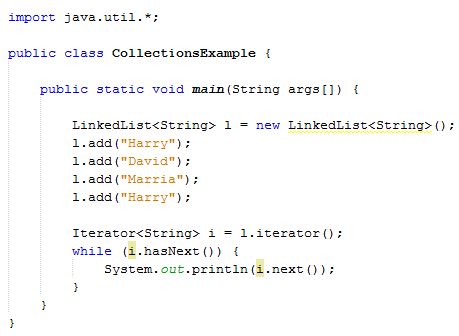
- import java.util.*;
- public class CollectionsExample {
- public static void main(String args[]) {
- LinkedList < String > l = new LinkedList < String > ();
- l.add("Harry");
- l.add("David");
- l.add("Marria");
- l.add("Harry");
- Iterator < String > i = l.iterator();
- while (i.hasNext()) {
- System.out.println(i.next());
- }
- }
- }
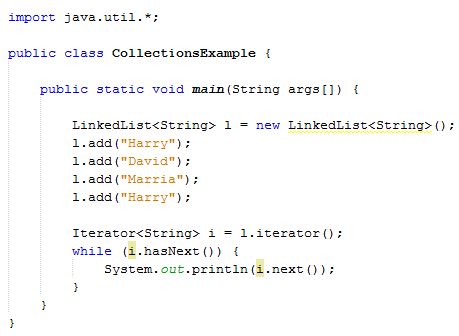
Output
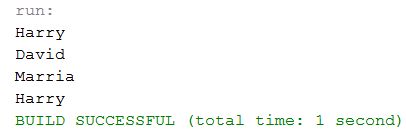
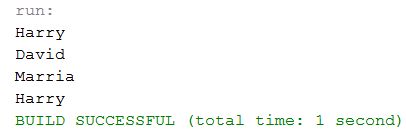
Summary
Thus, we learnt that Java collections, LinkedList class uses doubly linked list to store the elements. It extends the AbstractList class and implements the list and deque the interfaces. It can contain the duplicate elements, maintains an insertion order and also learnt how to create it in Java.