CallableStatement Interface In Java
CallableStatement Interface
In JDBC, CallableStatement interface is used to call the stored procedures and functions. We can have a business logic on the database with the use of the stored procedures and functions, which will make the performance better because these are precompiled.
Difference between Stored Procedures and Functions
Stored Procedure | Function |
Stored Procedure is used to perform the business logic. | Function is used to perform the calculation. |
Stored procedure must not have the return type. | Function must have the return type. |
Stored procedure may return 0 or more values. | Function may return only one value. |
We can call functions from the procedure. | Procedure cannot be called from function. |
Stored procedure supports input and output parameters. | Function supports only an input parameter. |
In the stored procedures, exception handling uses try/catch block can be used. | In the user defined functions, exception handling uses try/catch can't be used. |
Get the object of CallableStatement
The connection interface prepareCall() method is used to return the object of CallableStatement.
Syntax
public CallableStatement prepareCall("{ call procedurename(?,?...?)}");
For example
CallableStatement st=con.prepareCall("{call mysprocedure(?,?)}");
It calls the procedure mysprocedure which receives two arguments.
Call the Stored procedure using JDBC
Let’s see an example, given below, to call the stored procedure, using JDBC.
Code
To call the stored procedure, first we need to create it in the database.
- create or replace procedure "STRPRO"
- (id IN NUMBER,
- name IN VARCHAR2)
- is
- begin
- insert into Student values(id,name);
- end;
- /
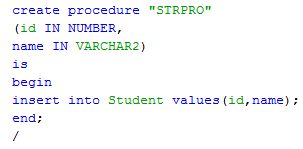
The table structure is given below.
create table student(id number(10), name varchar2(100));
Code
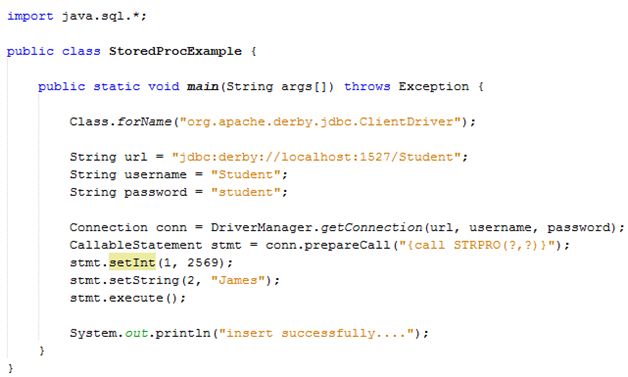
- import java.sql.*;
- public class StoredProcExample {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- CallableStatement stmt = conn.prepareCall("{call STRPRO(?,?)}");
- stmt.setInt(1, 2569);
- stmt.setString(2, "James");
- stmt.execute();
- System.out.println("insert successfully....");
- }
- }
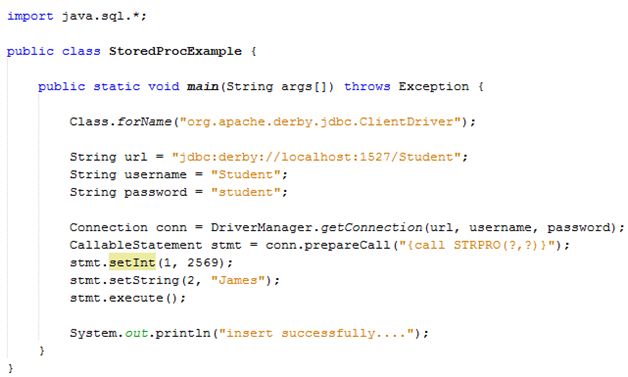
In the above example, we want to call the stored procedure STRPRO that receives id and name as the parameter and inserts it into the table Student. Now check the table in the database, value is inserted in the Student table.
Call the function using JDBC
Let’s see another example: to call the function using JDBC
Code
First create the simple function in the database.
- create or replace function sum1
- (n1 in number,n2 in number)
- return number
- is
- temp number(8);
- begin
- temp :=n1+n2;
- return temp;
- end;
- /
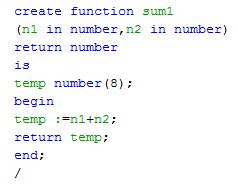
In this program, we are calling the sum1 function, which receives two inputs and returns the sum of the given number. Now, we have used the registerOutParameter method of CallableStatement interface, which registers the output parameter with its corresponding type. It gives information to the CallableStatement about the type of result being displayed.
The Types class describes many constants such as INTEGER, VARCHAR, FLOAT, DOUBLE etc.
Code
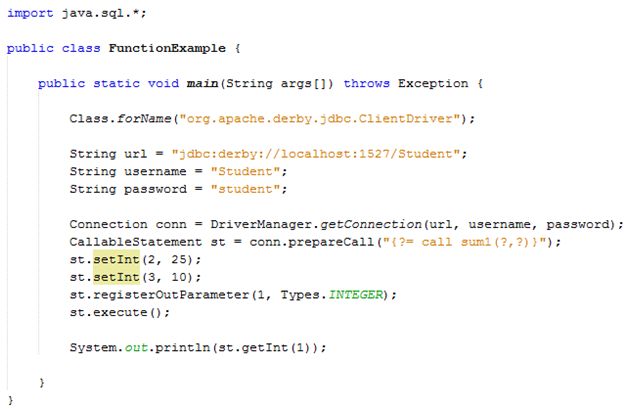
- import java.sql.*;
- public class FunctionExample {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- CallableStatement st = conn.prepareCall("{?= call sum1(?,?)}");
- st.setInt(2, 25);
- st.setInt(3, 10);
- st.registerOutParameter(1, Types.INTEGER);
- st.execute();
- System.out.println(st.getInt(1));
- }
- }
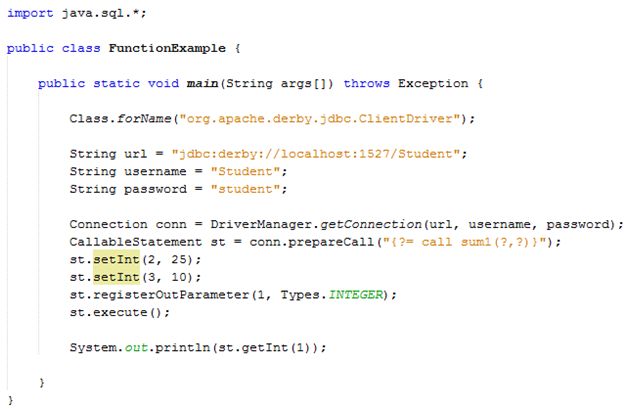
Summary
Thus, we learnt, JDBC CallableStatement interface is used to call the stored procedures and functions. We also learnt the difference between the stored procedures and functions in Java.