Abstraction In Java
Abstraction
In Java, abstraction is a procedure to hide the implementation details from the user and provides only functionality to the user.
In other words, it hides all the internal details and provides only important things to the user. For example in calculator, when we want to calculate some data, we use its keys but we don’t know, how it works internally. It provides only important things to the user and hides the internal process from the user.
In Java, there are two ways to achieve abstraction, which are,
- Abstract class
- Interface
Abstract class in Java
In Java, when we use abstract keyword with any class, this class is called as an abstract class.
- Abstract class can have abstract methods and non-abstract methods.
- If a class has an abstract method, this class must be abstract class.
- If we want to use an abstract class, it needs to be extended and its method implemented.
- If we extend an abstract class, we have to provide implementation for all the abstract methods in it.
- We can’t instantiate an abstract class.
Let’s see an example of an abstract class, given below.
Code
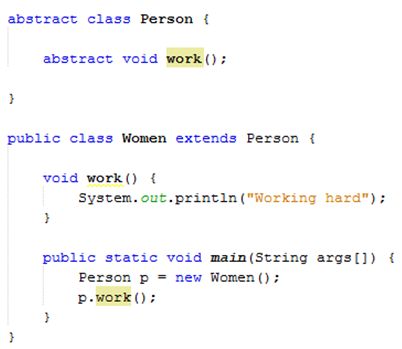
- abstract class Person {
- abstract void work();
- }
- public class Women extends Person {
- void work() {
- System.out.println("Working hard");
- }
- public static void main(String args[]) {
- Person p = new Women();
- p.work();
- }
- }
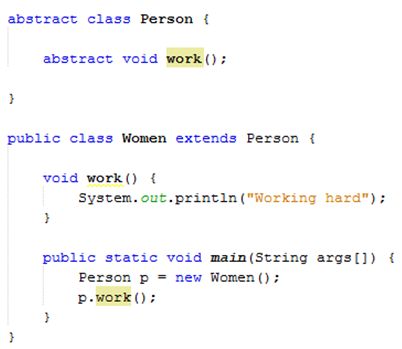
Output


In this example, we create person class, which contains one abstract method work and its implementation is provided by the women class.
Abstract method in Java
In Java, when we use abstract keyword with any method, the method is called as an abstract method and an abstract method contains a method signature, but no method body.
For example


Another example of abstraction is given below.
Code
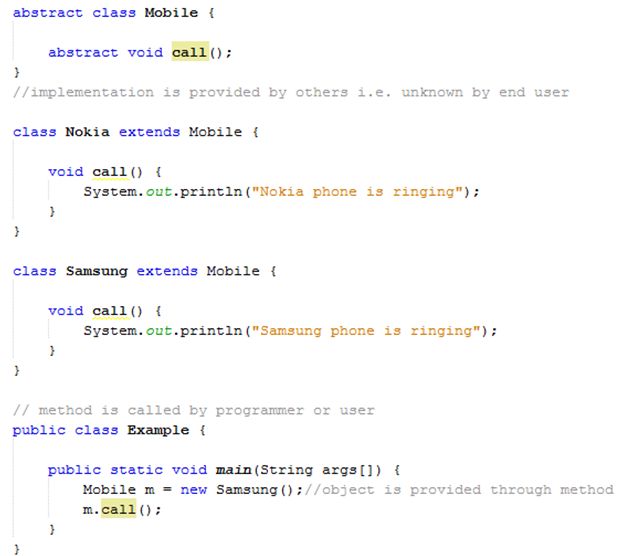
- abstract class Mobile {
- abstract void call();
- }
- //implementation is provided by others i.e. unknown by end user
- class Nokia extends Mobile {
- void call() {
- System.out.println("Nokia phone is ringing");
- }
- }
- class Samsung extends Mobile {
- void call() {
- System.out.println("Samsung phone is ringing");
- }
- }
- // method is called by programmer or user
- public class Example {
- public static void main(String args[]) {
- Mobile m = new Samsung(); //object is provided through method
- m.call();
- }
- }
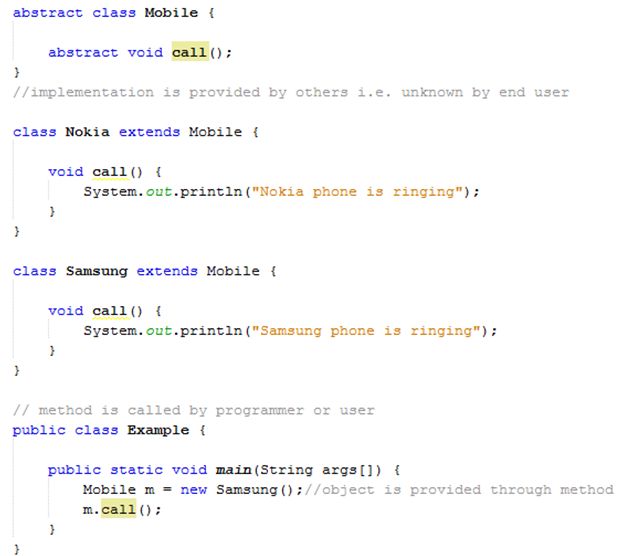
Output


In this example, Mobile class is an abstract class and its implementation is provided by Nokia and Samsung classes. In it, if we create an instance of Nokia class, call () method of Nokia class will be invoked similar to Samsung class. In reality, we don’t know about the implementation class i.e. hidden to the user and object of the implementation class is provided by the factory method.
Factory method is used to return the instance of the class.
An abstract class can have variables, abstract method, method body, constructor and main () method also.
Let’s see an example, given below.
Code
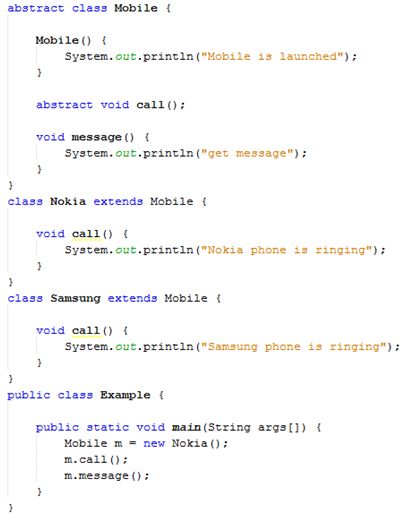
- abstract class Mobile {
- Mobile() {
- System.out.println("Mobile is launched");
- }
- abstract void call();
- void message() {
- System.out.println("get message");
- }
- }
- class Nokia extends Mobile {
- void call() {
- System.out.println("Nokia phone is ringing");
- }
- }
- class Samsung extends Mobile {
- void call() {
- System.out.println("Samsung phone is ringing");
- }
- }
- public class Example {
- public static void main(String args[]) {
- Mobile m = new Nokia();
- m.call();
- m.message();
- }
- }
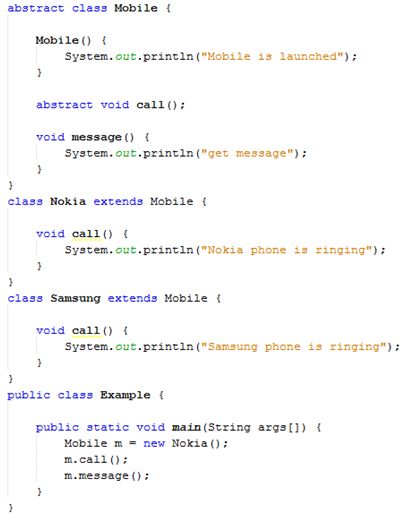
Output
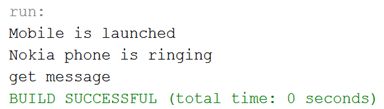
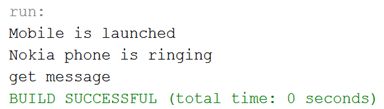
In the example, shown above, Mobile class has a constructor, abstract method and non-abstract method and returns an output.
Summary
Thus, we learnt that an abstraction hides all the internal details and provides only important things to the user and also learnt how to create an abstract class and method in Java.