Introduction Of String In Java
String
In Java programming, strings are generally used. Strings are a sequence of characters.
In Java, string is an object, which represents a sequence of characters. String class is used to create string object.
In other words, string is basically an object, which represents a sequence of char values. Java platform provides the String class to create and manipulate the strings.
Java String provides the various concepts, which can be performed on a string such as compare, concat, equals length, format, join, replace, compareTo, toLowerCase, toUpperCase substring etc.
An array of characters works similar as Java string.
Let’s see an example, given below.


In string-
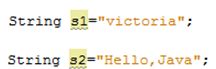
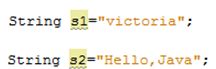
How to create String object in Java?
In Java, two ways to create String object.
- String Literal
In Java, string literal is created by using double quotes.
Let’s see an example, given below.
In the example, shown above, each time, when we create a string literal, JVM checks the string constant pool first. If the string is already present in the pool, a reference to the pooled instance is returned. if string is not present in the pool, a new string instance is created and placed in the pool.
Let’s see an example, given below.
In the example, shown above, only one object will be created. First, JVM checks and it will not find any string object with the value "Emma" in the string constant pool, so it will create a new object. Afterwards, it will find the string with the value "Emma" in the pool. It will not create a new object but it will return the reference to the same object.
Let’s understand with the figure, given below.
String objects are stored in a special memory area, which is called as a string constant pool in Java.
Java uses concept of string literal. Java uses the concept of string literal, because it makes Java more memory efficient or save memory. - By new keyword
In the example, shown above, JVM will create a new string object in normal heap memory and the literal "William" will be positioned in the string constant pool. The variable s1 will refer to the object in heap memory.
Let’s see an example, given below.
Code
- public class StringTest {
- public static void main(String args[]) {
- String name1 = "James";//creating string by java string literal
- char a[] = {'A', 'l', 'e', 'x', 'a', 'n', 'd', 'e', 'r'};
- String name2 = new String(a);//converting char array to string
- String name3 = new String("Olivia");//creating java string by new keyword
- System.out.println(name1);
- System.out.println(name2);
- System.out.println(name3);
- }
- }
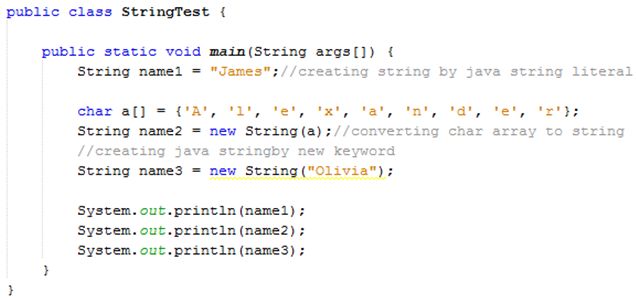
Output
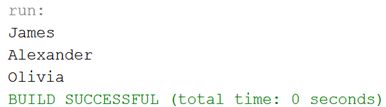
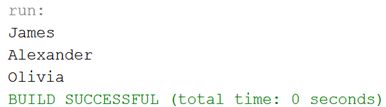
In the example, shown above, we create the string by Java string literal and by new keyword.
Java String class methods
In Java, java.lang.String class provides lots of useful methods to perform the operations on the sequence of char values.
Methods
char charAt(int index)
This method returns char value for the particular index.
int length()
This method returns string length.
static String format(String format, Object... args)
This method returns formatted string.
static String format(Locale l, String format, Object... args)
This method returns the formatted string with the given locale.
String substring(int beginIndex)
This method returns the substring for the given begin index.
String substring(int beginIndex, int endIndex)
This method returns the substring for the given begin index and end index.
boolean contains(CharSequence s)
This method returns true or false after matching the sequence of char value.
static String join(CharSequence delimiter, CharSequence... elements)
This method returns a joined string.
static String join(CharSequence delimiter, Iterable<? extends CharSequence> elements)
This method returns a joined string.
boolean equals(Object another)
This method checks the equality of the string with object.
boolean isEmpty()
This method checks if the string is empty.
String concat(String str)
This method concatenates the specified string.
String replace(char old, char new)
This method replaces all the occurrences of the specified char value.
String replace(CharSequence old, CharSequence new)
This method replaces all the occurrences of the specified CharSequence.
String trim()
This method returns the trimmed string omitting leading and trailing spaces.
String split(String regex)
This method returns split string matching regex.
String split(String regex, int limit)
This method returns split string matching regex and limit.
String intern()
The intern() method helps to compare two string objects.
int indexOf(int ch)
This method returns the specified char value index.
int indexOf(int ch, int fromIndex)
This method returns the specified char value index starting with given index.
int indexOf(String substring)
This method returns the specified substring index.
int indexOf(String substring, int fromIndex)
This method returns the specified substring index starting with given index.
String toLowerCase()
This method returns the string in lowercase.
String toLowerCase(Locale l)
This method returns the string in lowercase using specified locale.
String toUpperCase()
This method returns the string in uppercase.
String toUpperCase(Locale l)
This method returns the string in uppercase, using specified locale.
This method returns the string in uppercase, using specified locale.
Summary
Thus, we learnt that the string is an object, which represents a sequence of characters and also learnt how to create a string object in Java and string class methods.