Substring In Java
Substring
In Java, substring is a part of string.
In other words, substring is a subset of another string.
Method substring() is used to get a substring of a particular string in Java.
In Java, we have two methods to get the substring from the given string object.
- public String substring(int startIndex)
It returns new string object, which contains the substring of the given string from the particular startIndex (inclusive). - public String substring(int startIndex, int endIndex)
It returns new string object, which contains the substring of the given string from particular startIndex to endIndex.
Case of string in Java
- startIndex: inclusive
- endIndex: exclusive
Index starts from 0 always.
Let's see an example, given below.
Code
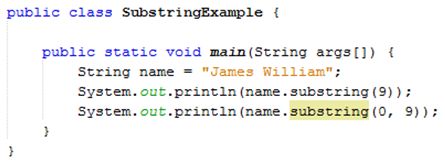
- public class SubstringExample {
- public static void main(String args[]) {
- String name = "James William";
- System.out.println(name.substring(9));
- System.out.println(name.substring(0, 9));
- }
- }
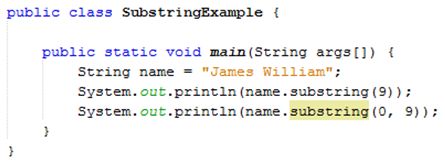
Output
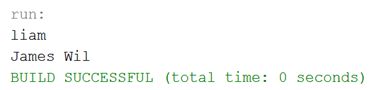
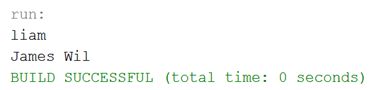
Let’s see another example, given below.
Code
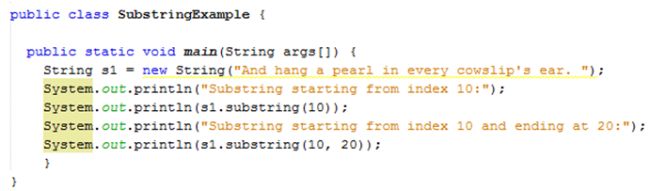
Output
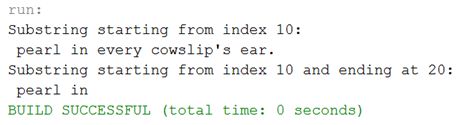
- public class SubstringExample {
- public static void main(String args[]) {
- String s1 = new String("And hang a pearl in every cowslip's ear. ");
- System.out.println("Substring starting from index 10:");
- System.out.println(s1.substring(10));
- System.out.println("Substring starting from index 10 and ending at 20:");
- System.out.println(s1.substring(10, 20));
- }
- }
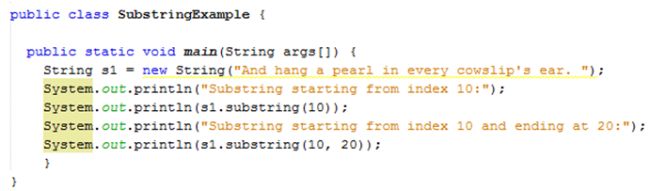
Output
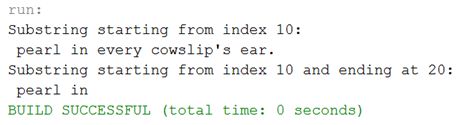
Summary
Thus, we learnt that the substring is a subset of another string and also learnt their two methods, which are used to get a substring of a particular string in Java.