Map Interface In Java
Map Interface
In Java, map interface is used to contain the values on the basis of key i.e. key and value pair. Each key and value pair is known as an entry. Map contains unique keys only. It is useful, when we have to search, update or delete elements on the basis of key.
Methods of Map Interface
void clear( )
This method is used to remove all key/value pairs from the calling map.
boolean containsKey(Object k)
This method is used to return true, if the calling map contains k as a key, else it returns false.
boolean containsValue(Object v)
This method is used to return true, if the map contains v as a value, else, it returns false.
Set entrySet( )
This method is used to return a set, which contains the entries in the map. The set contains objects of type Map.Entry.
boolean equals(Object obj)
This method is used to return true, if object is a map and contains the same entries, else, it returns false.
Object get(Object k)
This method is used to return the value associated with the key k.
int hashCode( )
This method is used to return the hash code for the calling map.
boolean isEmpty( )
This method is used to return true, if the calling map is empty, else, it returns false.
Set keySet( )
This method is used to return a set, which contains the keys in the calling map. This method provides a set-view of the keys in the calling map.
Object put(Object k, Object v)
This method is used to put an entry in the calling map, overwriting any previous value associated with the key. It returns null, if the key does not already exist.
void putAll(Map m)
This method is used to put all the entries from m into the map.
Object remove(Object k)
This method is used to remove the entry, where key equals k.
int size( )
This method is used to return the number of key/value pairs in the map.
Collection values( )
This method is used to return a collection containing the values in the map. This method provides a collection-view of the values in the map.
Let’s see an example, given below.
Code
- import java.util.*;
- public class CollectionsExample {
- public static void main(String[] args) {
- Map m = new HashMap();
- m.put("Harry", "8");
- m.put("Potter", "3");
- m.put("Ash", "2");
- m.put("Mia", "1");
- System.out.println();
- System.out.println(" Map Elements:");
- System.out.print(m);
- }
- }
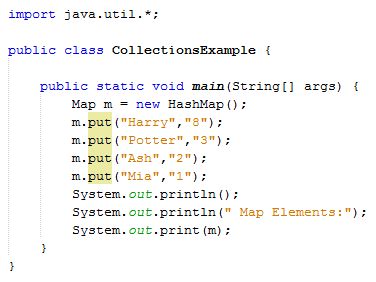
Output
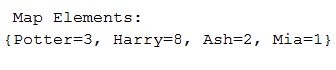
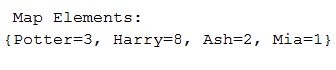
Entry Interface
Entry is the sub interface of the map. The Map.Entry interface enables us to work with a map entry.
The entrySet( ) method is declared by the map interface, which is used to return a set, which contains the map entries. Each of these set elements is a Map.Entry object.
Methods of Map.Entry Interface
boolean equals(Object obj)
This method is used to return true, if an object is a Map.Entry, where key and value are equal to that of the invoking object.
Object getKey( )
This method is used to return the key for the map entry.
Object getValue( )
This method is used to return the value for the map entry.
int hashCode( )
This method is used to return the hash code for the map entry.
Object setValue(Object v)
This method is used to set the value for the map entry to v and a ClassCastException is thrown, if v is not the correct type for the map. A NullPointerException is thrown, if v is null and the map does not allow null keys. An UnsupportedOperationException is thrown, if the map cannot be changed.
Let’s see an example, given below.
Code
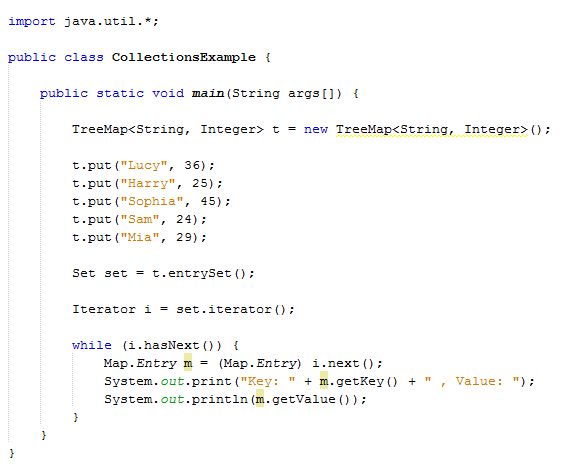
- import java.util.*;
- public class CollectionsExample {
- public static void main(String args[]) {
- TreeMap < String, Integer > t = new TreeMap < String, Integer > ();
- t.put("Lucy", 36);
- t.put("Harry", 25);
- t.put("Sophia", 45);
- t.put("Sam", 24);
- t.put("Mia", 29);
- Set set = t.entrySet();
- Iterator i = set.iterator();
- while (i.hasNext()) {
- Map.Entry m = (Map.Entry) i.next();
- System.out.print("Key: " + m.getKey() + " , Value: ");
- System.out.println(m.getValue());
- }
- }
- }
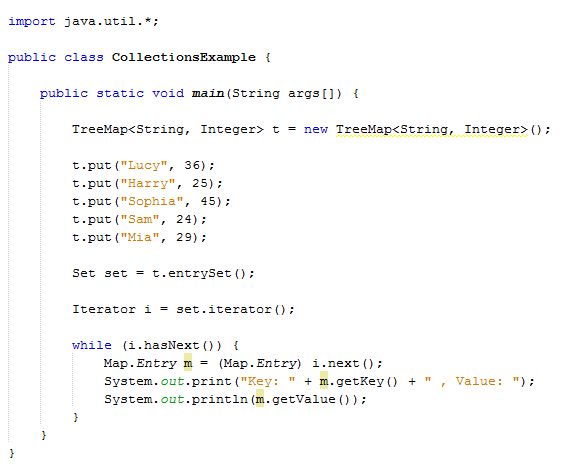
Output
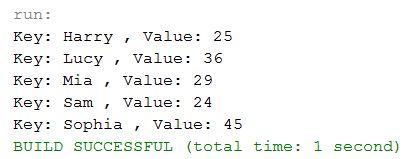
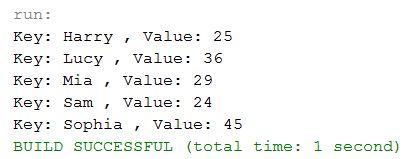
Summary
Thus, we learnt that Java map interface is used to contain the values on the basis of key i.e. key and value pair. Each key and value pair is known as an entry. Map contains unique keys only and also learnt how we can use Map.Entry interface in Java.