Introduction Of Reflection API In Java
Reflection API
In Java, Reflection is a procedure of examining or modifying the run time behavior of a class at the run time. The java.lang.Class class gives many methods, which can be used to get metadata, examine and change the run time behavior of a class.
The java.lang package and java.lang.reflect package give classes for Java reflection.
Usage of Reflection API
The Reflection API is generally used in,
- IDE (Integrated Development Environment) like Eclipse, MyEclipse, NetBeans
- Debugger
- Test Tools
java.lang.Class class
The java.lang.Class class is involved mainly in two tasks, which are,
- This class helps the methods to get the metadata of a class at the run time.
- This class helps the methods to examine and change the run time behavior of a class.
Methods of Class “class”
public String getName()
This method is used to return the class name.
public static Class forName(String className)throws ClassNotFoundException
This method is used to load the class and return the reference of Class “class.”
public Object newInstance()throws InstantiationException,IllegalAccessException
This method is used to create new instance.
public boolean isInterface()
This method is used to check, if it is an interface.
public boolean isArray()
This method is used to check, if it is an array.
public boolean isPrimitive()
This method is used to check, if it is primitive.
public Class getSuperclass()
This method is used to return the super class “class” reference.
public Field[] getDeclaredFields()throws SecurityException
This method is used to return the total number of fields of the class.
public Method[] getDeclaredMethods()throws SecurityException
This method is used to return the total number of the methods of the class.
public Constructor[] getDeclaredConstructors()throws SecurityException
This method is used to return the total number of the constructors of this class.
public Method getDeclaredMethod(String name,Class[] parameterTypes)throws NoSuchMethodException,SecurityException
This method is used to return the method class object.
Get the object of Class class
There are three ways to get the instance of Class “class”, which are,
- forName() method of Class class
- getClass() method of Object class
- the .class syntax
forName() method of Class class
This method is used to load the class dynamically and also return the object of Class “class”.
It should be used, if we know the full qualified name of the class and it cannot be used for primitive types.
Let's see an example of forName() method, given below,
Code
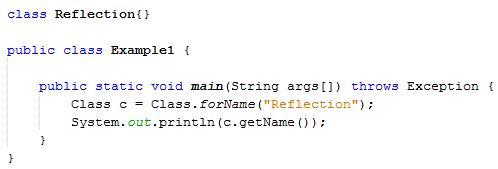
- class Reflection{}
- public class Example1 {
- public static void main(String args[]) throws Exception {
- Class c = Class.forName("Reflection");
- System.out.println(c.getName());
- }
- }
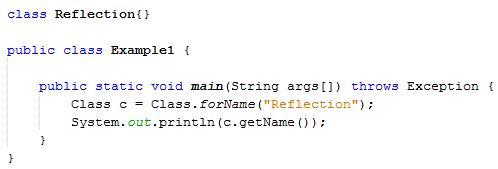
getClass() method of Object class
This method is used to return the object of Class “class” and it should be used, if we know the type. Additionally, it can be used with primitives.
Let’s see an example of getClass() method, given below.
Code
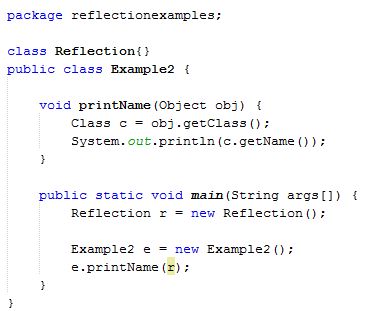
- package reflectionexamples;
- class Reflection {}
- public class Example2 {
- void printName(Object obj) {
- Class c = obj.getClass();
- System.out.println(c.getName());
- }
- public static void main(String args[]) {
- Reflection r = new Reflection();
- Example2 e = new Example2();
- e.printName(r);
- }
- }
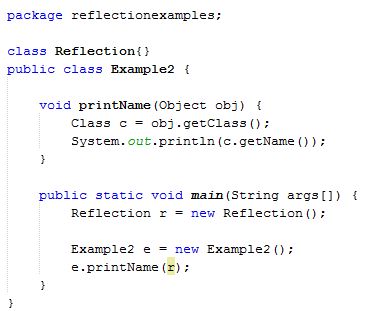
Output


The .class syntax
When a type is presented but there is no object, it is possible to obtain a Class by appending ".class" to the name of the type and it can be used for primitive data type also.
Let’s see an example of .class syntax.
Code
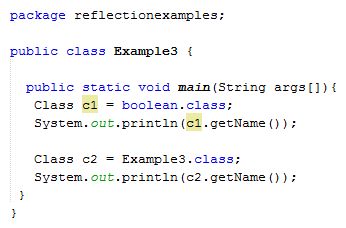
- package reflectionexamples;
- public class Example3 {
- public static void main(String args[]) {
- Class c1 = boolean.class;
- System.out.println(c1.getName());
- Class c2 = Example3.class;
- System.out.println(c2.getName());
- }
- }
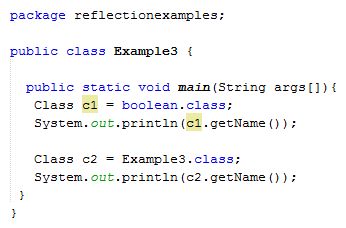
Output
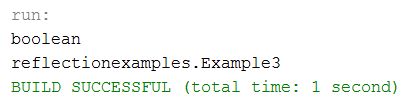
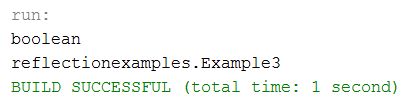
Determine the class object
Some methods of Class “class” are used to determine the class object, which are,
public boolean isInterface()
This method is used to determine, if the particular class object represents an interface type.
public boolean isArray()
This method is used to determine, if the class object represents an array class.
public boolean isPrimitive()
This method is used to determine, if the particular class object represents a primitive type.
Let's see an example of reflection API to determine the object type, given below.
Code
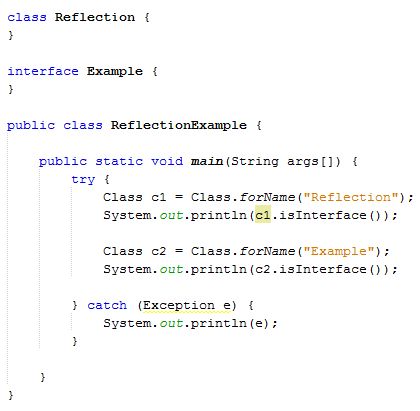
- class Reflection {}
- interface Example {}
- public class ReflectionExample {
- public static void main(String args[]) {
- try {
- Class c1 = Class.forName("Reflection");
- System.out.println(c1.isInterface());
- Class c2 = Class.forName("Example");
- System.out.println(c2.isInterface());
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
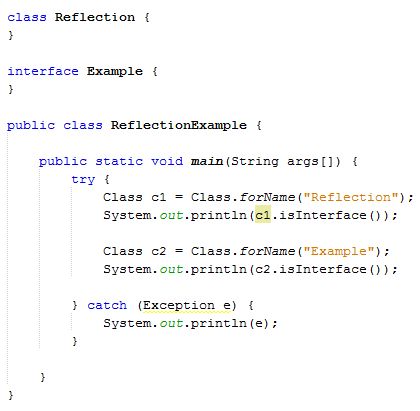
Summary
Thus, we learnt that Java Reflection is a procedure to examine or modify the run time behavior of a class at the run time and also learnt how we can use it in Java.