Naming Thread And Current Thread In Java
Naming Thread
In Java, Thread class provides methods to change or set and get the name of a thread.
By default, every thread has a name i.e. thread-0, thread-1 and so on.
We can change the name of the thread with the usage of setName() method.
Syntax
public String getName()
This method is used to return the name of a thread.
public void setName(String name)
This method is used to change the name of a thread.
Let’s see an example of naming a thread, given below.
Code
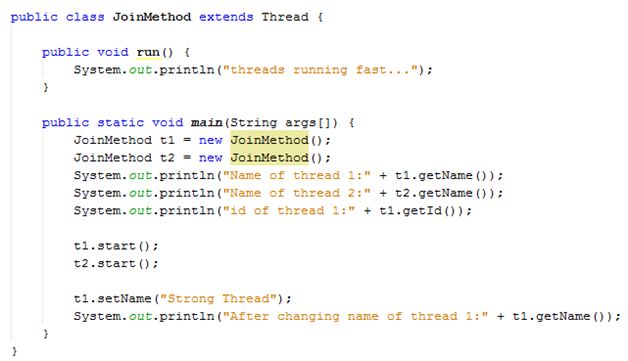
- public class JoinMethod extends Thread {
- public void run() {
- System.out.println("threads running fast...");
- }
- public static void main(String args[]) {
- JoinMethod t1 = new JoinMethod();
- JoinMethod t2 = new JoinMethod();
- System.out.println("Name of thread 1:" + t1.getName());
- System.out.println("Name of thread 2:" + t2.getName());
- System.out.println("id of thread 1:" + t1.getId());
- t1.start();
- t2.start();
- t1.setName("Strong Thread");
- System.out.println("After changing name of thread 1:" + t1.getName());
- }
- }
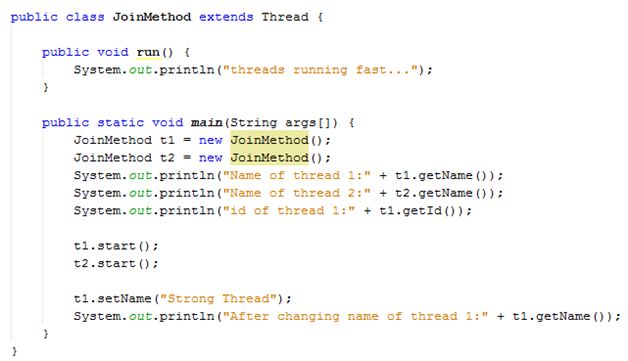
Output
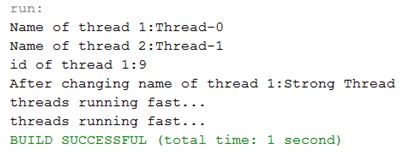
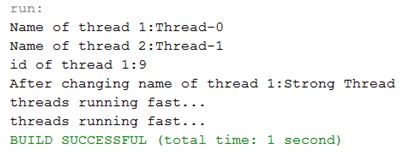
In the example, mentioned above, we can see that with the help of the methods, we can get or set the name of the thread and also change it.
CurrentThread() method
The CurrentThread() method is used to return a reference to the currently executing thread object.
Syntax
Public static Thread currentThread()
Let’s see a simple example, given below.
Code
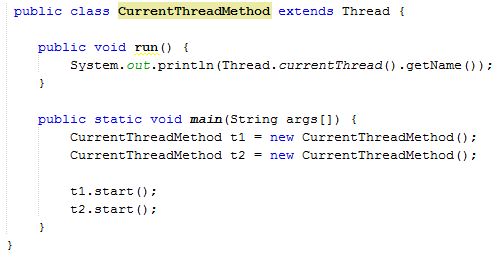
- public class CurrentThreadMethod extends Thread {
- public void run() {
- System.out.println(Thread.currentThread().getName());
- }
- public static void main(String args[]) {
- CurrentThreadMethod t1 = new CurrentThreadMethod();
- CurrentThreadMethod t2 = new CurrentThreadMethod();
- t1.start();
- t2.start();
- }
- }
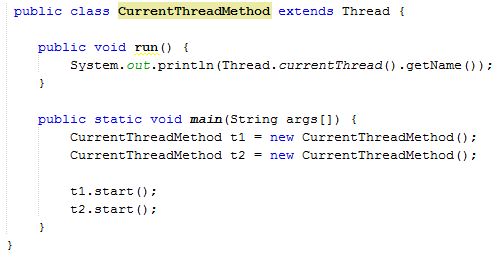
Output
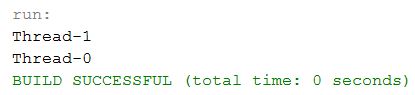
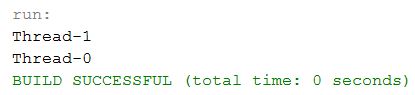
In the example, mentioned above, we can see that with the usage of currentThread() method, we can easily get the name of the current thread, which is currently running.
Summary
Thus, we learnt, the methods to set and get the name of a thread, currentThread() method and also learnt, how to use these methods in Java.