Static Keyword In Java
Static Keyword
Static is a non-access modifier in Java. A static keyword is a member of a class rather than instance of a class. This is mainly used for memory management in Java. Static keyword can apply with variables, methods, blocks, and nested class.
Static variable
In Java, when we use static keyword with any variable that variable called as a static variable. It is used to refer the common property of all objects and in class area at the time of class loading it gets memory only once. It helps to saves the memory. It is also used to retain the value.
Let’s see an example of static variable.
Code
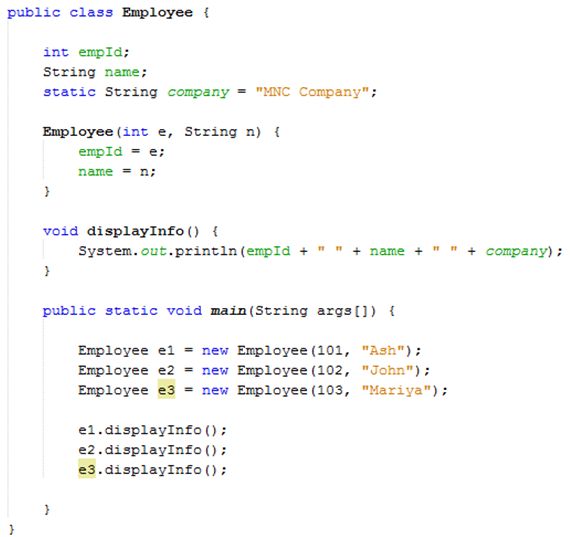
- public class Employee {
- int empId;
- String name;
- static String company = "MNC Company";
- Employee(int e, String n) {
- empId = e;
- name = n;
- }
- void displayInfo() {
- System.out.println(empId + " " + name + " " + company);
- }
- public static void main(String args[]) {
- Employee e1 = new Employee(101, "Ash");
- Employee e2 = new Employee(102, "John");
- Employee e3 = new Employee(103, "Mariya");
- e1.displayInfo();
- e2.displayInfo();
- e3.displayInfo();
- }
- }
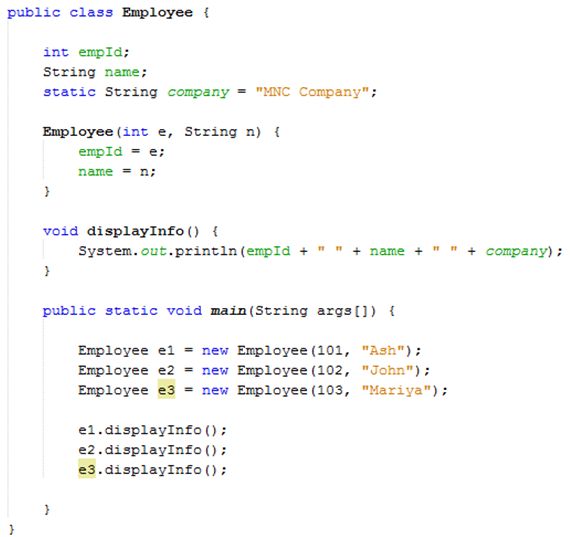
Output
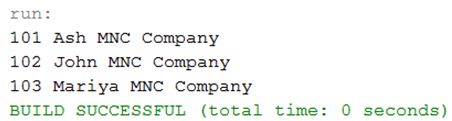
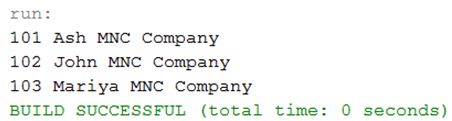
In the above example, the entire employees have different empId and name. But company name refers to the common property of all objects that’s why we use static keyword. Because static gets memory only one time and helps to saves the memory.
Static method
In Java, when we use static keyword with any method that method called as a static method. Static method is also a member of a class than object of a class. Static method can invoked without creating an object of a class. Static method can directly access static data members and we can change the value of it with the use of static.
Limitations of static method
- We cannot use this and super in static framework.
- Static method cannot use non-static data member.
- Static method cannot call directly non-static method.
Let’s see an example of static method.
Code
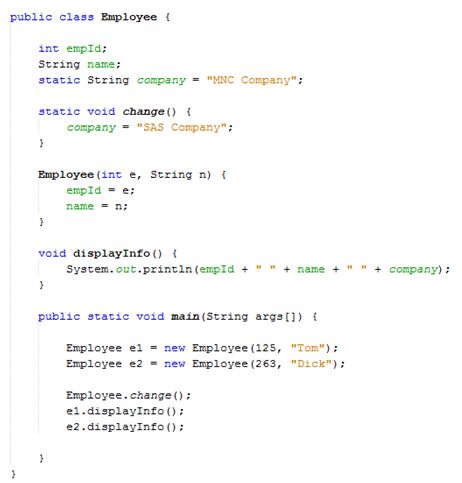
- public class Employee {
- int empId;
- String name;
- static String company = "MNC Company";
- static void change() {
- company = "SAS Company";
- }
- Employee(int e, String n) {
- empId = e;
- name = n;
- }
- void displayInfo() {
- System.out.println(empId + " " + name + " " + company);
- }
- public static void main(String args[]) {
- Employee e1 = new Employee(125, "Tom");
- Employee e2 = new Employee(263, "Dick");
- Employee.change();
- e1.displayInfo();
- e2.displayInfo();
- }
- }
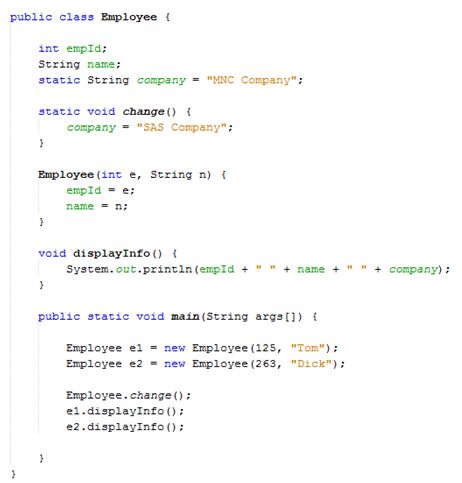
Output
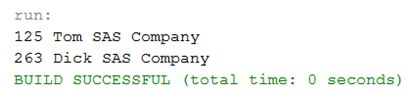
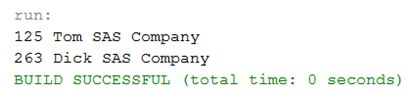
In Java main method is static. Why?
In Java main method is static because object is not necessary to call a static method. If main method is non static method than may be it creates a problem. JVM first create an instance of a class than call the main method and it may be gets extra memory allocation.
Static block
Static block mainly used to initialize the static data member and at runtime, it is executed before main method.
Let’s see an example of static block.
Code
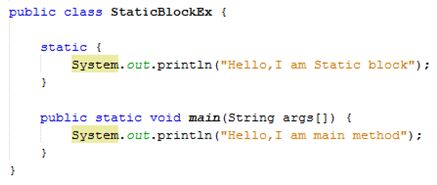
- public class StaticBlockEx {
- static {
- System.out.println("Hello,I am Static block");
- }
- public static void main(String args[]) {
- System.out.println("Hello,I am main method");
- }
- }
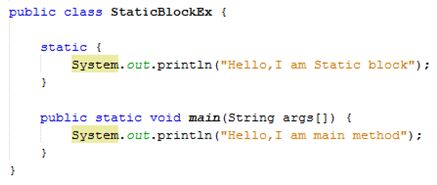
Output
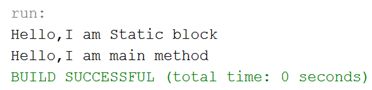
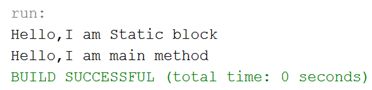
We can execute a program without main method with the use of static block. But (before JDK 1.7) only in previous version of JDK not in new version.
Summary
Summary
Thus, we learned that a static keyword is a member of a class rather than instance of a class. This is mainly used for memory management in Java and also learns how we can use it in Java.