Recursion In Java
Recursion
Recursion in Java is a procedure where a method calls itself continuously. A method that calls itself is called recursive method in Java.
In other words, recursion is the process of defining something in terms of itself. Recursion is an attribute, which allows a method to call itself. A method that calls itself is said to be recursive in Java.
Syntax
returntype methodname(){
//code
methodname();//calling same method
}
Let’s see an example of recursion, given below.
Code
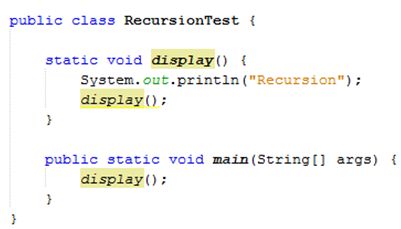
- public class RecursionTest {
- static void display() {
- System.out.println("Recursion");
- display();
- }
- public static void main(String[] args) {
- display();
- }
- }
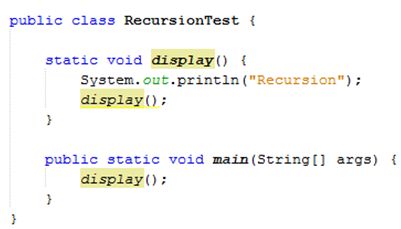
Output
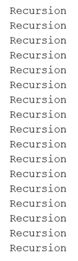
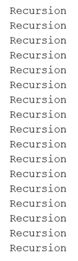
Infinite times……………
Let’s see an example of recursion, given below.
Code
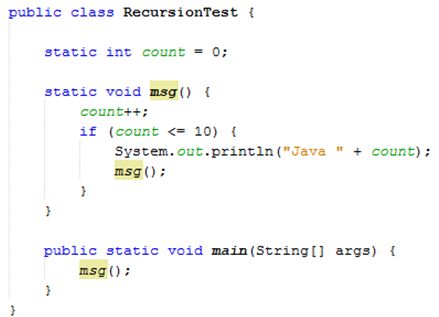
Output
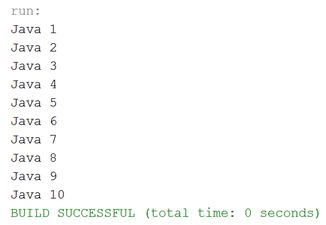
- public class RecursionTest {
- static int count = 0;
- static void msg() {
- count++;
- if (count <= 10) {
- System.out.println("Java " + count);
- msg();
- }
- }
- public static void main(String[] args) {
- msg();
- }
- }
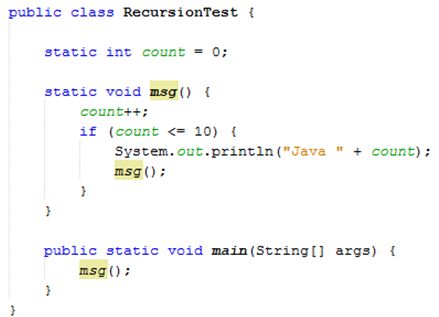
Output
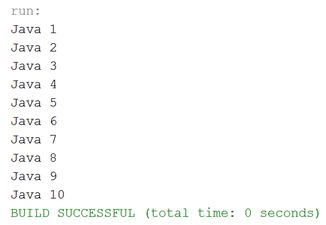
Finite times…….
Let’s see an example of recursion, given below.
Code
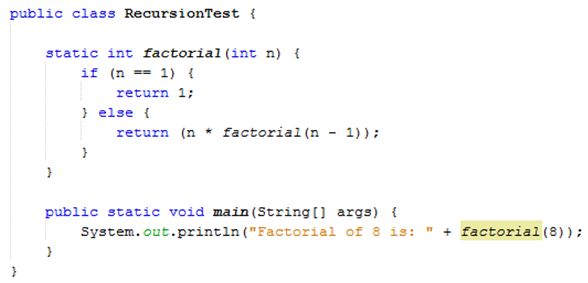
- public class RecursionTest {
- static int factorial(int n) {
- if (n == 1) {
- return 1;
- } else {
- return (n * factorial(n - 1));
- }
- }
- public static void main(String[] args) {
- System.out.println("Factorial of 8 is: " + factorial(8));
- }
- }
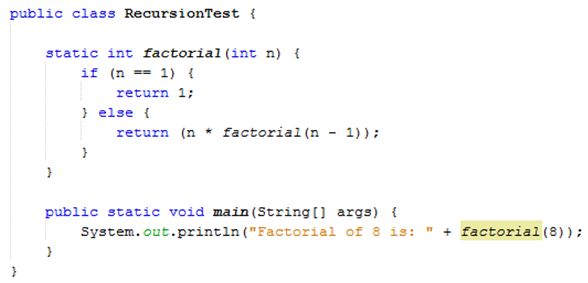
Output


Factorial number……
Let’s see an example of recursion, given below.
Code
- public class RecursionTest {
- static int n1 = 0, n2 = 1, n3 = 0;
- static void fibon(int count) {
- if (count > 0) {
- n3 = n1 + n2;
- n1 = n2;
- n2 = n3;
- System.out.print(" " + n3);
- fibon(count - 1);
- }
- }
- public static void main(String[] args) {
- int count = 10;
- System.out.print(n1 + " " + n2); //printing 0 and 1
- fibon(count - 2); //n-2 because 2 numbers are already printed
- }
- }
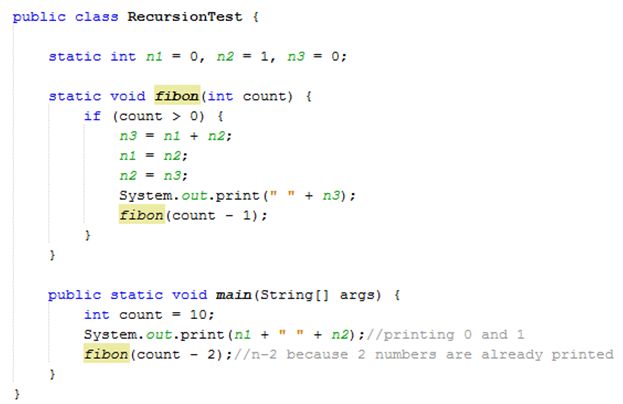
Output
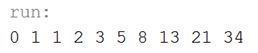
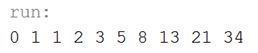
Fibonacci series……….
Summary
Thus, we learnt that recursion in Java is a procedure in which a method calls itself continuously and also learnt how we can create a program, using recursion in Java.