How To Connect To The Oracle Database
Java application connects with the oracle database.
There are some important steps to perform database connectivity.
We are using Oracle10g as the database.
- In JDBC, the driver class for Oracle database is oracle.jdbc.driver.OracleDriver.
- In JDBC, the connection URL for Oracle10G database is jdbc:oracle:thin:@localhost:1521:xe. In it, jdbc is API, Oracle is the database, thin is the driver and localhost is the Server name on which Oracle is running. We use IP address, 1521 is the port number and Oracle Service name is a XE and get all the information from the tnsnames.ora file.
- Username for Oracle database is a system by default and the password is given by the user at the time of installing Oracle database.
First create a table in oracle database.
create table student(id number(20),name varchar2(50),age number(3));
Now, let’s see an example to connect Java Application with Oracle database, given below.
Code
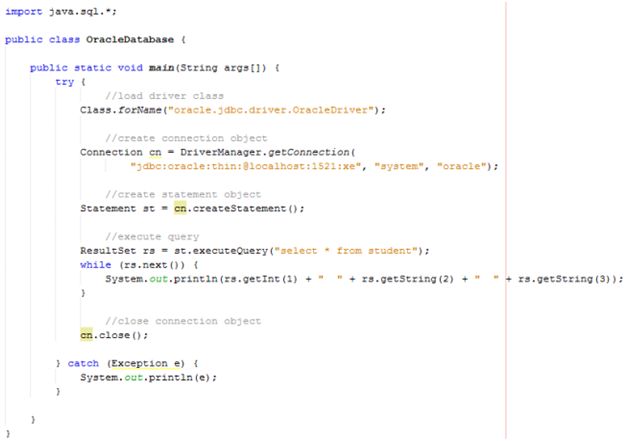
- import java.sql.*;
- public class OracleDatabase {
- public static void main(String args[]) {
- try {
- //load driver class
- Class.forName("oracle.jdbc.driver.OracleDriver");
- //create connection object
- Connection cn = DriverManager.getConnection(
- "jdbc:oracle:thin:@localhost:1521:xe", "system", "oracle");
- //create statement object
- Statement st = cn.createStatement();
- //execute query
- ResultSet rs = st.executeQuery("select * from student");
- while (rs.next()) {
- System.out.println(rs.getInt(1) + " " + rs.getString(2) + " " + rs.getString(3));
- }
- //close connection object
- cn.close();
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
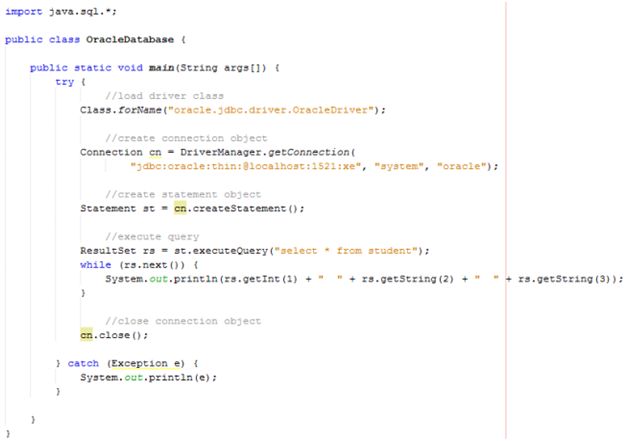
In the example, shown above, system is the username and Oracle is the password of the Oracle database. Moreover, we are fetching all the records of student table.
Java Application connects with Oracle database ojdbc14.jar file, which is required to be loaded.
There are two ways to load the jar file.
- paste the ojdbc14.jar file in jre/lib/ext folder
- set classpath
- paste the ojdbc14.jar file in JRE/lib/ext folder
Search the ojdbc14.jar file.
Go to JRE/lib/ext folder and paste the jar file at this point. - set classpath
There are 2 ways to set the classpath, which are given below.
Temporary set classpath.
Permanent set classpath.
Temporary set classpath
- Search the ojdbc14.jar file.
- Open command prompt and write: C:>set classpath=c:\folder\ojdbc14.jar;.;
Permanent set classpath
- Go to environment variable.
- Click new tab: In variable name write classpath and in variable value paste the path to ojdbc14.jar by adding ojdbc14.jar;.; as C:\oraclexe\app\oracle\product\10.2.0\server\jdbc\lib\ojdbc14.jar;.;
Summary
Thus, we learnt, some important steps to perform Oracle database connectivity and also learn how we can set a classpath in Java.