DatabaseMetaData Interface In Java
DatabaseMetaData Interface
In JDBC, DatabaseMetaData interface provides many methods to get the meta data of a database like database product name, database product version, name of the total number of the tables and name of total number of views etc.
Methods of DatabaseMetaData interface
public String getDriverName()throws SQLException
This method is used to return the name of JDBC driver.
public String getDriverVersion()throws SQLException
This method is used to return the version number of JDBC driver.
public String getUserName()throws SQLException
This method is used to return the username of the database.
public String getDatabaseProductName()throws SQLException
This method is used to return the product name of the database.
public String getDatabaseProductVersion()throws SQLException
This method is used to return the product version of the database.
public ResultSet getTables(String catalog, String schemaPattern, String tableNamePattern, String[] types)throws SQLException
This method is used to return the explanation of the tables of the particular catalog. The table type can be TABLE, VIEW, ALIAS, SYSTEM TABLE, SYNONYM etc.
Get the DatabaseMetaData object
The getMetaData() method of the connection interface is used to return the DatabaseMetaData object.
Syntax
public DatabaseMetaData getMetaData()throws SQLException
Let’s see an example1, given below.
Code
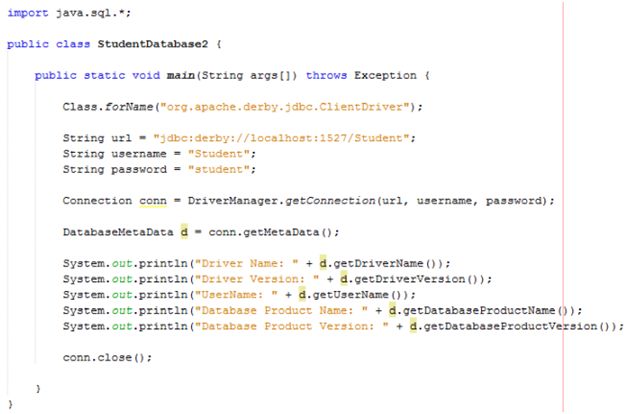
- import java.sql.*;
- public class StudentDatabase2 {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- DatabaseMetaData d = conn.getMetaData();
- System.out.println("Driver Name: " + d.getDriverName());
- System.out.println("Driver Version: " + d.getDriverVersion());
- System.out.println("UserName: " + d.getUserName());
- System.out.println("Database Product Name: " + d.getDatabaseProductName());
- System.out.println("Database Product Version: " + d.getDatabaseProductVersion());
- conn.close();
- }
- }
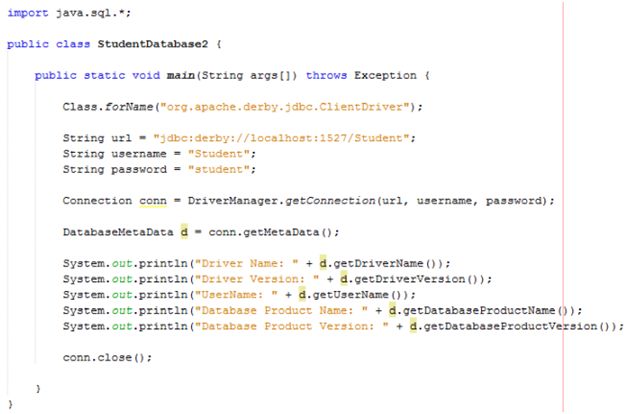
Output
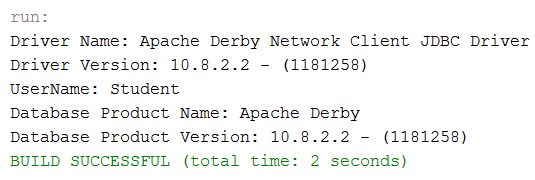
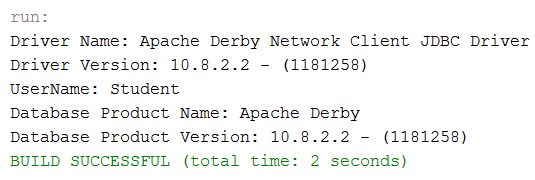
Let’s see an example2, which prints total number of tables, given below.
Code
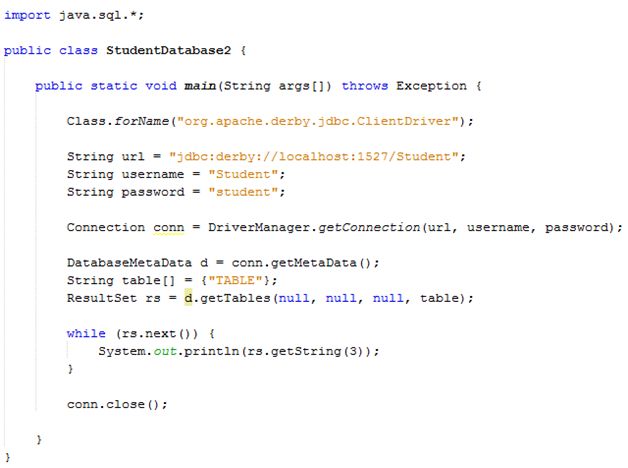
- import java.sql.*;
- public class StudentDatabase2 {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- DatabaseMetaData d = conn.getMetaData();
- String table[] = {"TABLE"};
- ResultSet rs = d.getTables(null, null, null, table);
- while (rs.next()) {
- System.out.println(rs.getString(3));
- }
- conn.close();
- }
- }
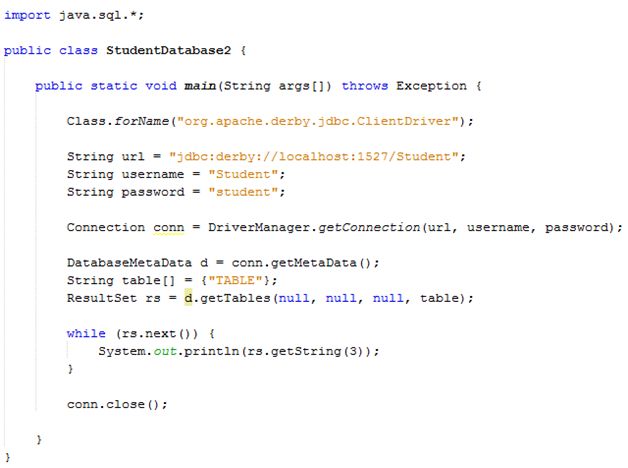
Output


Let’s see an example3, given below, which prints total number of views.
Code
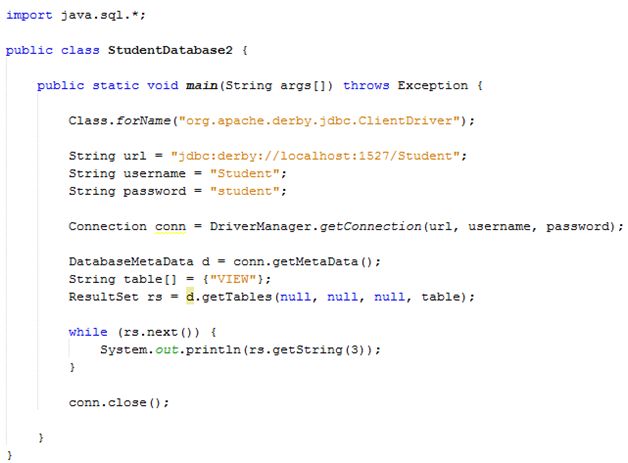
- import java.sql.*;
- public class StudentDatabase2 {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- DatabaseMetaData d = conn.getMetaData();
- String table[] = {"VIEW"};
- ResultSet rs = d.getTables(null, null, null, table);
- while (rs.next()) {
- System.out.println(rs.getString(3));
- }
- conn.close();
- }
- }
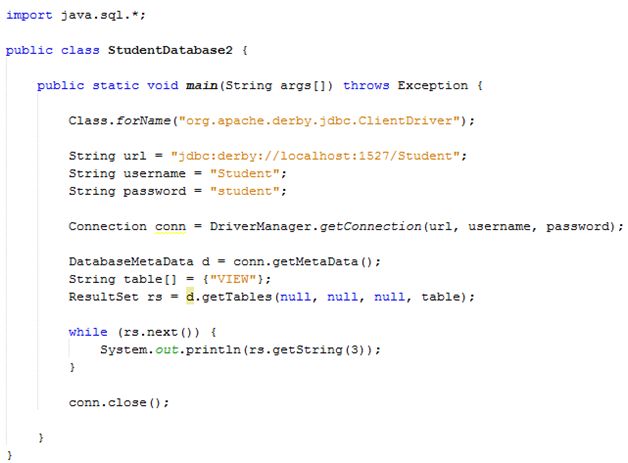
Output


In the above example, we can see that program don’t display anything because there is no view in the table of database.
Summary
Thus, we learnt, JDBC DatabaseMetaData interface provides many methods to get meta data of a database and also learnt its important methods in Java.