How To Store File In Database
Store file in Database
In JDBC, the PreparedStatement setCharacterStream() method is used to set the character information into the parameterIndex.
Syntax
public void setBinaryStream(int paramIndex,InputStream stream)throws SQLException
public void setBinaryStream(int paramIndex,InputStream stream,long length)throws SQLException
CLOB (Character Large Object) data type is used in the table for storing file into the database.
Example
CREATE TABLE "FILETABLE"
( "ID" NUMBER,
"NAME" CLOB
)
Let’s see an example, given below.
Code
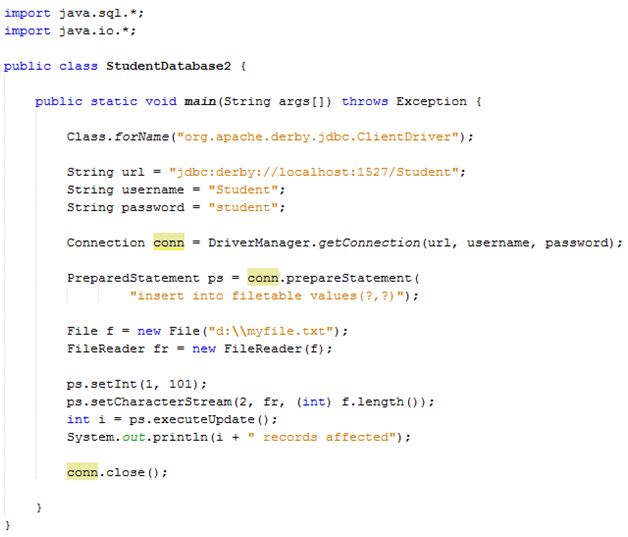
- import java.sql.*;
- import java.io.*;
- public class StudentDatabase2 {
- public static void main(String args[]) throws Exception {
- Class.forName("org.apache.derby.jdbc.ClientDriver");
- String url = "jdbc:derby://localhost:1527/Student";
- String username = "Student";
- String password = "student";
- Connection conn = DriverManager.getConnection(url, username, password);
- PreparedStatement ps = conn.prepareStatement(
- "insert into filetable values(?,?)");
- File f = new File("d:\\myfile.txt");
- FileReader fr = new FileReader(f);
- ps.setInt(1, 101);
- ps.setCharacterStream(2, fr, (int) f.length());
- int i = ps.executeUpdate();
- System.out.println(i + " records affected");
- conn.close();
- }
- }
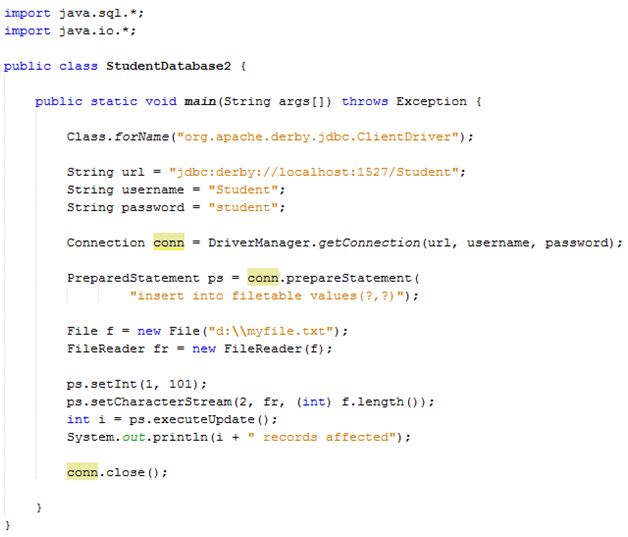
Output


Summary
Thus, we learnt, PreparedStatement setCharacterStream() method is used to set the character information into the parameterIndex and also learnt, how to store file in Java database.